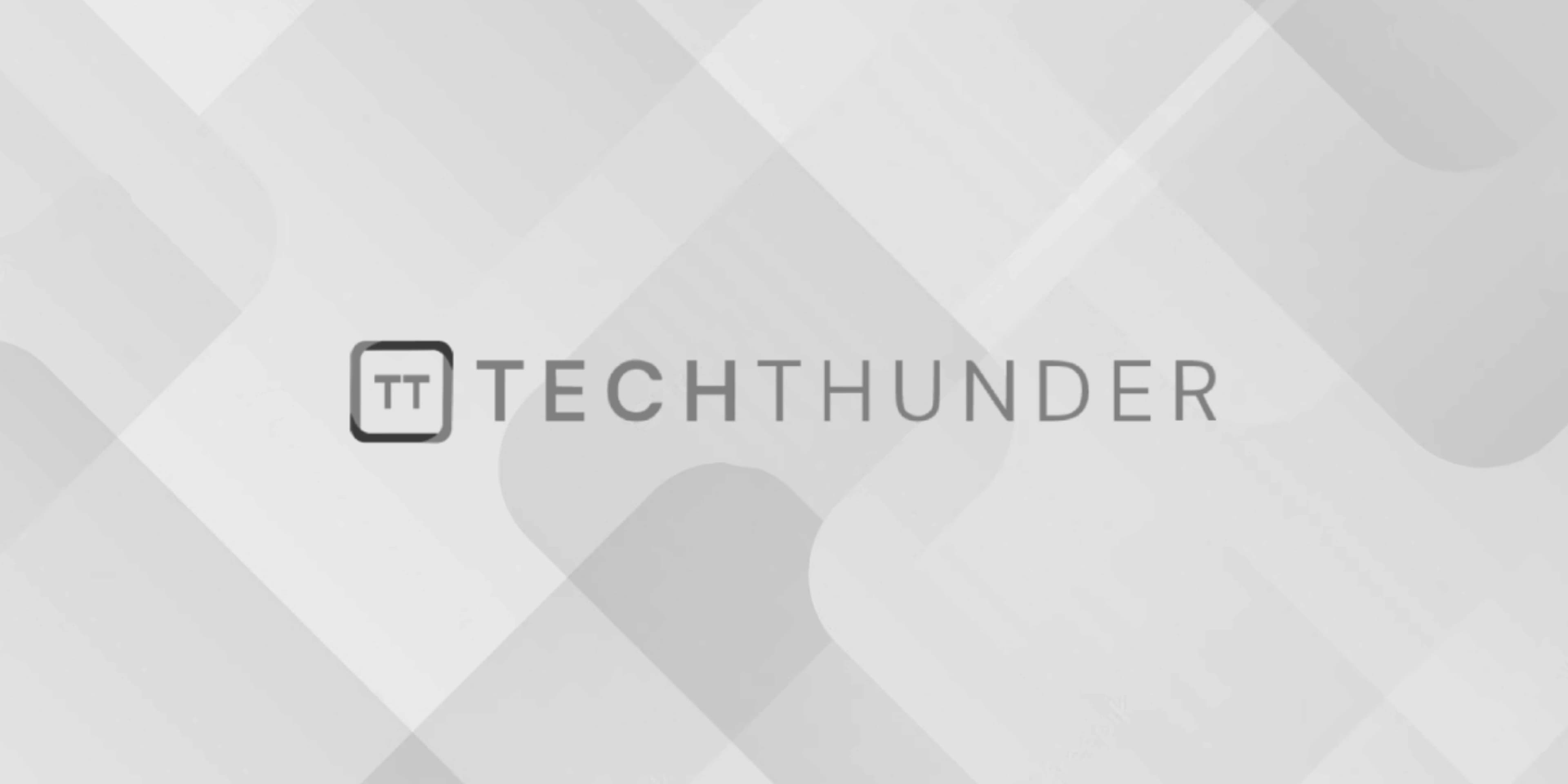
jQuery prevAll() method
The jQuery.prevAll()
method is used to select all the immediately preceding sibling elements of each element in the jQuery object. It allows you to target all the sibling elements that appear before the selected element in the DOM hierarchy.
Here’s the basic syntax of the prevAll()
method:
$(selector).prevAll([filter])
Parameters:
selector
: Optional. A selector expression used to filter the preceding sibling elements. If provided, only the preceding siblings that match the selector will be selected.filter
: Optional. A selector expression used to further filter the selected preceding siblings.
Return Value:
The prevAll()
method returns a new jQuery object containing the selected preceding sibling elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery prevAll() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div>Div 1</div>
<p>Paragraph 1</p>
<span>Span 1</span>
<div>Div 2</div>
<script>
$(document).ready(function() {
// Select all immediately preceding siblings of the <span> element
var precedingSiblings = $("span").prevAll();
// Log the selected preceding siblings
console.log(precedingSiblings);
});
</script>
</body>
</html>
In this example, we use the prevAll()
method to select all the immediately preceding sibling elements of the <span>
element. The method returns a jQuery object containing the <p>
and <div>
elements, which are the siblings that appear before the <span>
element in the DOM hierarchy.
The output of the above example will be:
[<p>Paragraph 1</p>, <div>Div 1</div>]
In this output, we see that the prevAll()
method successfully selected all the preceding sibling elements of the <span>
element.
If you provide a selector
or filter
argument to the prevAll()
method, it will only select the preceding siblings that match the specified selector or filter. For example:
var filteredPrecedingSiblings = $("span").prevAll("div");
This will select only the preceding siblings with the <div>
element type and exclude the <p>
element.
The prevAll()
method is useful when you want to target all the sibling elements that come immediately before a particular element in the DOM hierarchy. It provides a convenient way to traverse the DOM tree and select elements based on their position relative to other elements.