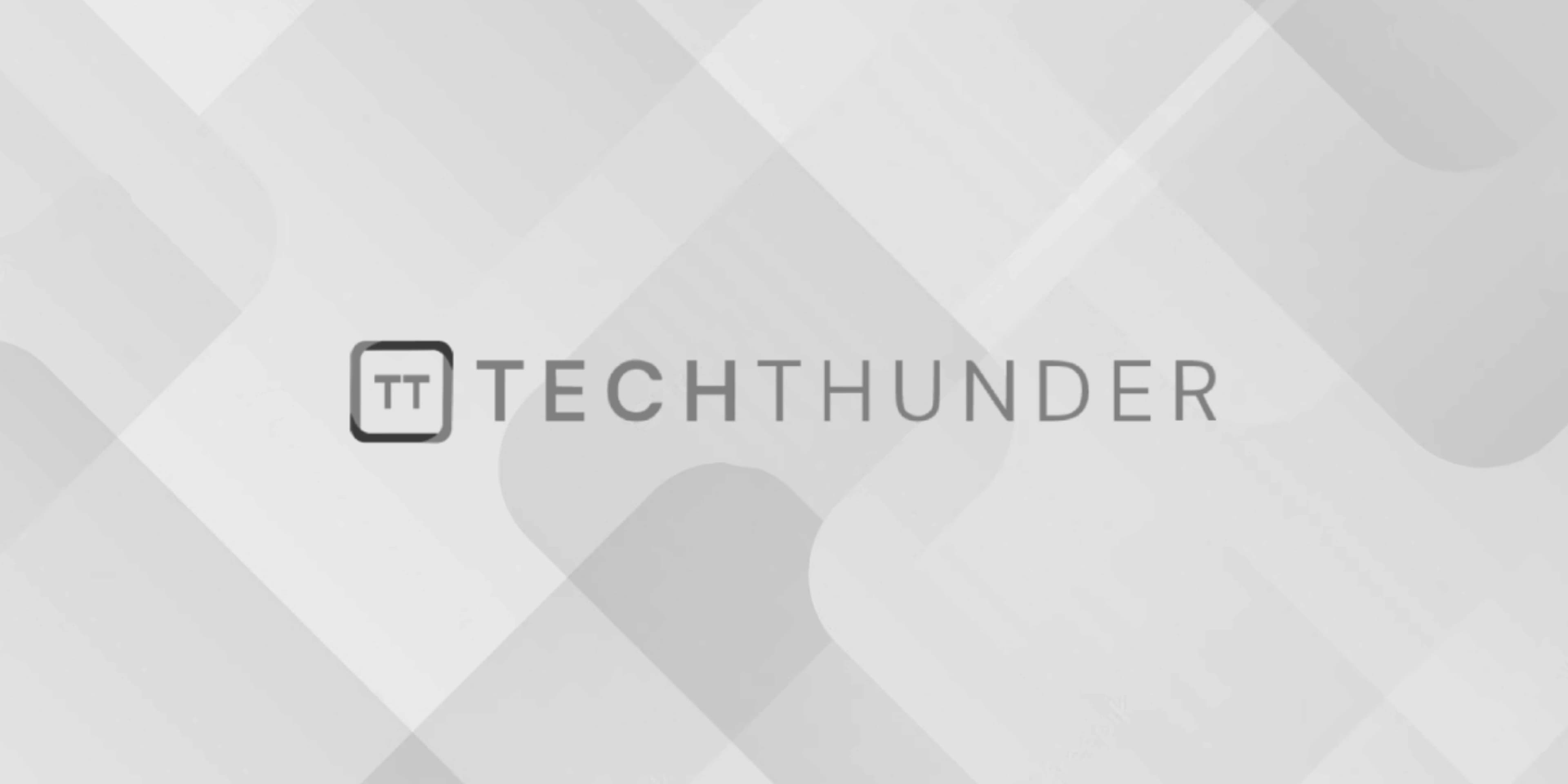
jQuery isEmptyObject() method
The jQuery.isEmptyObject()
method is a utility function in jQuery used to check whether an object is empty (contains no properties) or not. It is designed to provide a consistent way of determining if an object has any properties, even in cases where the native Object.keys()
method may not be available.
Here’s the basic syntax of the jQuery.isEmptyObject()
method:
jQuery.isEmptyObject(obj)
Parameters:
obj
: The object to be tested.
Return Value:
The jQuery.isEmptyObject()
method returns true
if the specified object has no properties (i.e., it is empty), and false
if the object has one or more properties.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery isEmptyObject() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<script>
$(document).ready(function() {
var emptyObject = {};
var nonEmptyObject = { name: "John", age: 30 };
// Check if the objects are empty
var isEmpty1 = jQuery.isEmptyObject(emptyObject);
var isEmpty2 = jQuery.isEmptyObject(nonEmptyObject);
// Log the results to the console
console.log("emptyObject is empty:", isEmpty1); // true
console.log("nonEmptyObject is empty:", isEmpty2); // false
});
</script>
</body>
</html>
In this example, we have two objects: emptyObject
and nonEmptyObject
. We use the jQuery.isEmptyObject()
method to check whether each of these objects is empty or not. The method returns true
for the emptyObject
because it has no properties (it is an empty object), and false
for the nonEmptyObject
because it has properties.
Keep in mind that starting from jQuery version 3.0, the recommended way to check if an object is empty is to use the native Object.keys()
method:
Object.keys(obj).length === 0
Using Object.keys()
is more widely supported in modern browsers and is the standard way to check for empty objects in JavaScript.
For modern projects, it is generally preferable to use Object.keys()
for better compatibility and performance. However, the jQuery.isEmptyObject()
method remains available in jQuery for backward compatibility with older codebases or specific use cases.