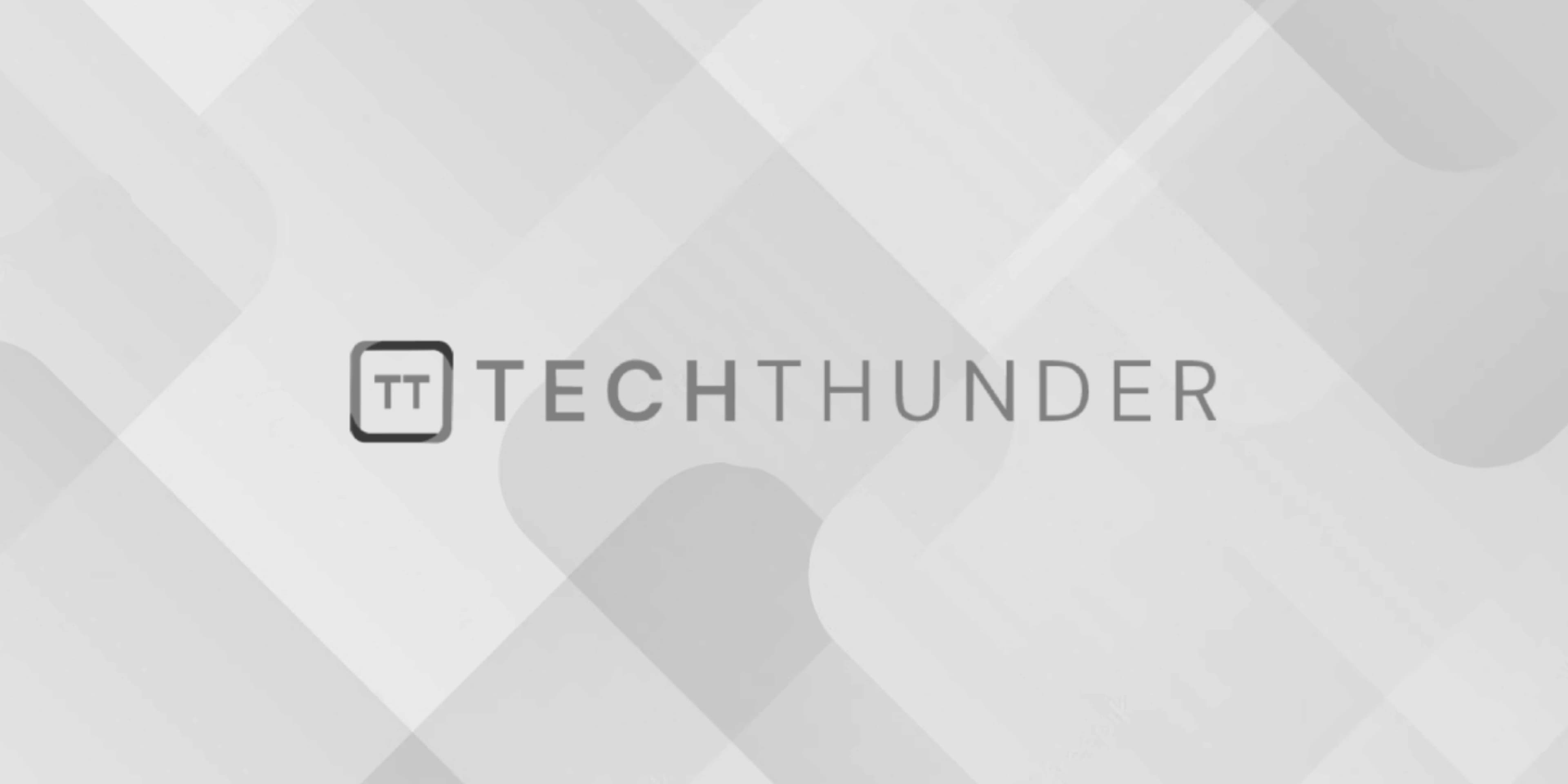
207 views
Allow only 10 numbers in textbox using Jquery
The restrict a text input field to allow only 10 numeric characters using jQuery, you can attach an event handler to the input field and check the input as the user types. If the input does not meet the criteria, you can prevent or modify the input. Here’s an example of how you can achieve this:
Assuming you have the following HTML for your text input field:
<input type="text" id="numericInput" maxlength="10">
You can use the following jQuery code to enforce the limit of 10 numeric characters:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#numericInput").on("input", function() {
// Get the current input value
var inputValue = $(this).val();
// Remove any non-numeric characters
var numericValue = inputValue.replace(/\D/g, "");
// Limit the input to 10 characters
var limitedValue = numericValue.slice(0, 10);
// Update the input field with the limited value
$(this).val(limitedValue);
});
});
</script>
In this example:
- We select the text input field with the ID “numericInput.”
- We attach an “input” event handler to the input field, which is triggered when the user enters or changes the input.
- In the event handler, we get the current input value.
- We use a regular expression (/\D/g) to remove any non-numeric characters from the input value, leaving only numeric characters.
- We limit the input to 10 characters by using the
slice()
method. - Finally, we update the input field with the limited value.
The text input field will allow only up to 10 numeric characters, and any non-numeric characters will be automatically removed as the user types.