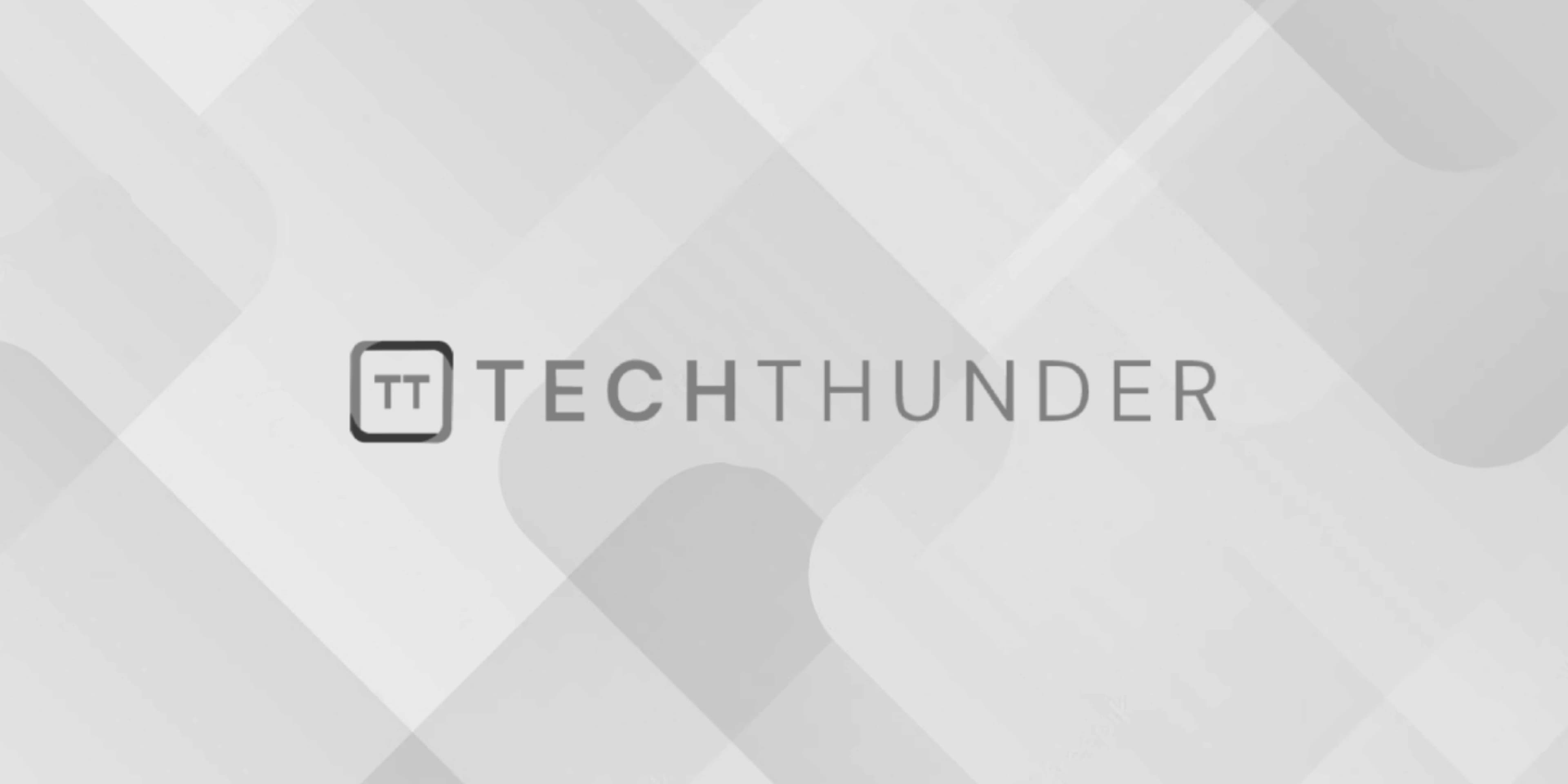
jQuery nth-of-type() selector
The jQuery does not have a specific nth-of-type()
selector like the one available in CSS. However, you can achieve the same functionality using the :eq()
selector or the .eq()
method combined with the element type selector.
The :eq()
selector selects the element at the specified index within the set of matched elements. It is zero-based, meaning the index starts at 0 for the first element. Here’s the basic syntax:
$('element:eq(index)')
Alternatively, you can use the .eq()
method to achieve the same result:
$('element').eq(index)
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery nth-of-type() Selector Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div>First div</div>
<p>First paragraph</p>
<div>Second div</div>
<p>Second paragraph</p>
<script>
$(document).ready(function() {
// Using :eq() selector
$('div:eq(1)').text('Modified second div using :eq() selector');
// Using .eq() method
$('p').eq(1).text('Modified second paragraph using .eq() method');
});
</script>
</body>
</html>
In this example, we use the :eq()
selector and the .eq()
method to target the second div
element and the second p
element, respectively. We then modify their content using the text()
method.
Keep in mind that the :eq()
selector and .eq()
method select only the elements at the specified index and do not perform filtering based on element types. If you need to select elements based on their type and index, you can combine the element type selector and the :eq()
selector or .eq()
method. For example:
$('element:eq(index)')
// or
$('element').eq(index)
Replace 'element'
with the specific element type (e.g., 'div'
, 'p'
, etc.), and 'index'
with the desired index (zero-based) to select the element at that position within the set of matched elements.