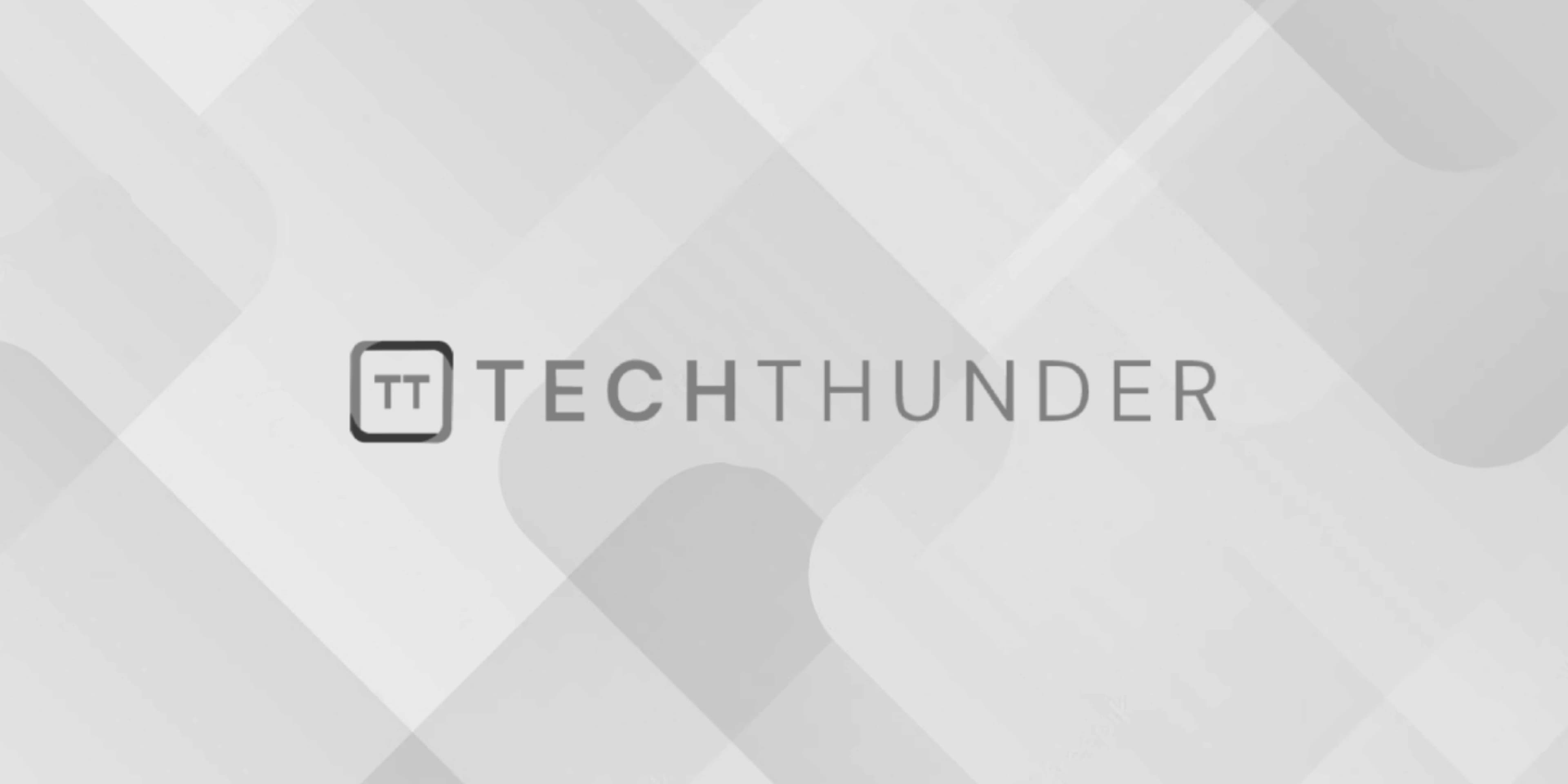
jQuery :visible selector
The :visible
selector is a built-in selector in jQuery used to select all elements that are currently visible on a web page. An element is considered visible if it has both a non-zero width and height and is not hidden using the CSS display
property or visibility: hidden
.
Here’s the basic syntax of the :visible
selector:
$(":visible")
This selector can be used to filter elements based on their visibility status and perform operations on them.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery :visible Selector Example</title>
<style>
.hidden-element {
display: none;
}
.visible-element {
width: 100px;
height: 50px;
background-color: lightblue;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="visible-element">This div is visible.</div>
<div class="hidden-element">This div is hidden.</div>
<button id="toggleVisibility">Toggle Visibility</button>
<script>
$(document).ready(function() {
$('#toggleVisibility').click(function() {
// Toggle visibility of the divs when the button is clicked
$('.visible-element, .hidden-element').toggle();
});
});
</script>
</body>
</html>
In this example, we have two div elements: one with the class visible-element
, which is initially visible due to the specified width and height, and another with the class hidden-element
, which is initially hidden using display: none
.
When you click the “Toggle Visibility” button, the :visible
selector is used to select all visible elements (in this case, the .visible-element
div), and the toggle()
method is applied to both the visible and hidden elements to toggle their visibility.
The visible div will be hidden, and the hidden div will become visible.
Please note that elements hidden using the CSS visibility: hidden
property will still be considered visible by the :visible
selector, as they occupy space on the page. Only elements hidden using display: none
will be excluded by the :visible
selector.