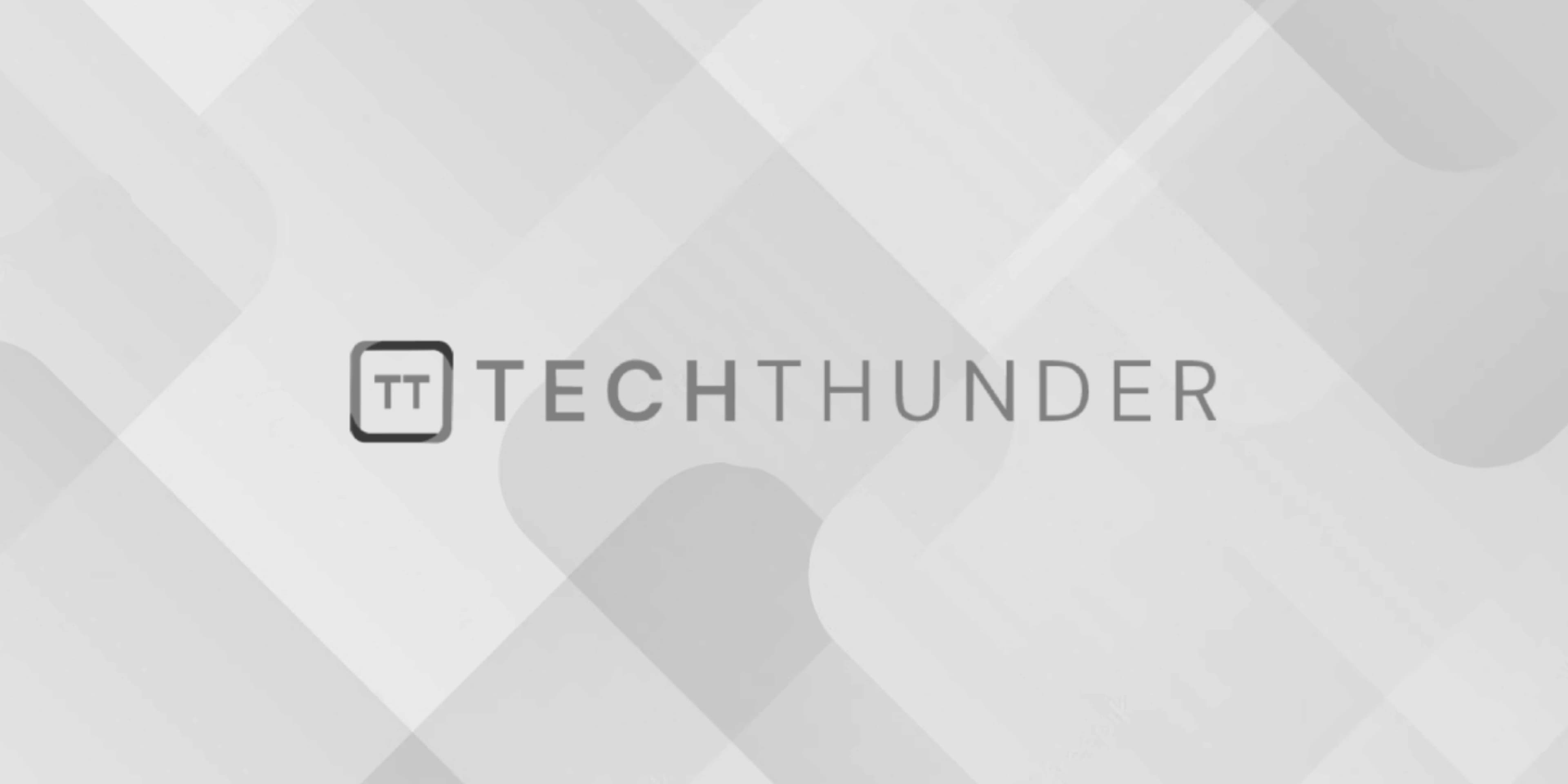
jQuery getJSON() method
The jQuery getJSON()
method is used to fetch JSON data from a server using an HTTP GET request. It is a shorthand method for making AJAX requests specifically designed for retrieving JSON data. The getJSON()
method provides an easy way to work with JSON data, as it automatically parses the JSON response and passes the resulting JavaScript object to the success callback function.
Here’s the basic syntax of the getJSON()
method:
$.getJSON(url, data, success)
Parameters:
url
: The URL of the server endpoint from which to fetch the JSON data.data
: (Optional) Data to be sent to the server. It can be a plain object or a string in query string format.success
: A callback function that will be executed when the request is successful. The function is passed the parsed JSON data as an argument.
Example:
Suppose you have a JSON file called data.json
with the following content:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
You can use the getJSON()
method to fetch and work with this JSON data as follows:
<!DOCTYPE html>
<html>
<head>
<title>jQuery getJSON() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="output"></div>
<script>
$(document).ready(function() {
// Fetch JSON data from data.json
$.getJSON("data.json", function(data) {
// This function will be executed upon successful retrieval of JSON data
// The 'data' parameter will contain the parsed JSON data
// Display the JSON data in the 'output' div
$("#output").html("Name: " + data.name + "<br>" +
"Age: " + data.age + "<br>" +
"City: " + data.city);
});
});
</script>
</body>
</html>
In this example, the getJSON()
method is used to fetch data from the data.json
file. The success callback function receives the parsed JSON data as the data
parameter. The data is then displayed in the output
div of the HTML page.
The getJSON()
method is a convenient way to work with JSON data in AJAX requests, especially when you expect JSON data as the response from the server. It handles the parsing of the JSON data for you, making it easier to work with JSON responses in your JavaScript code.