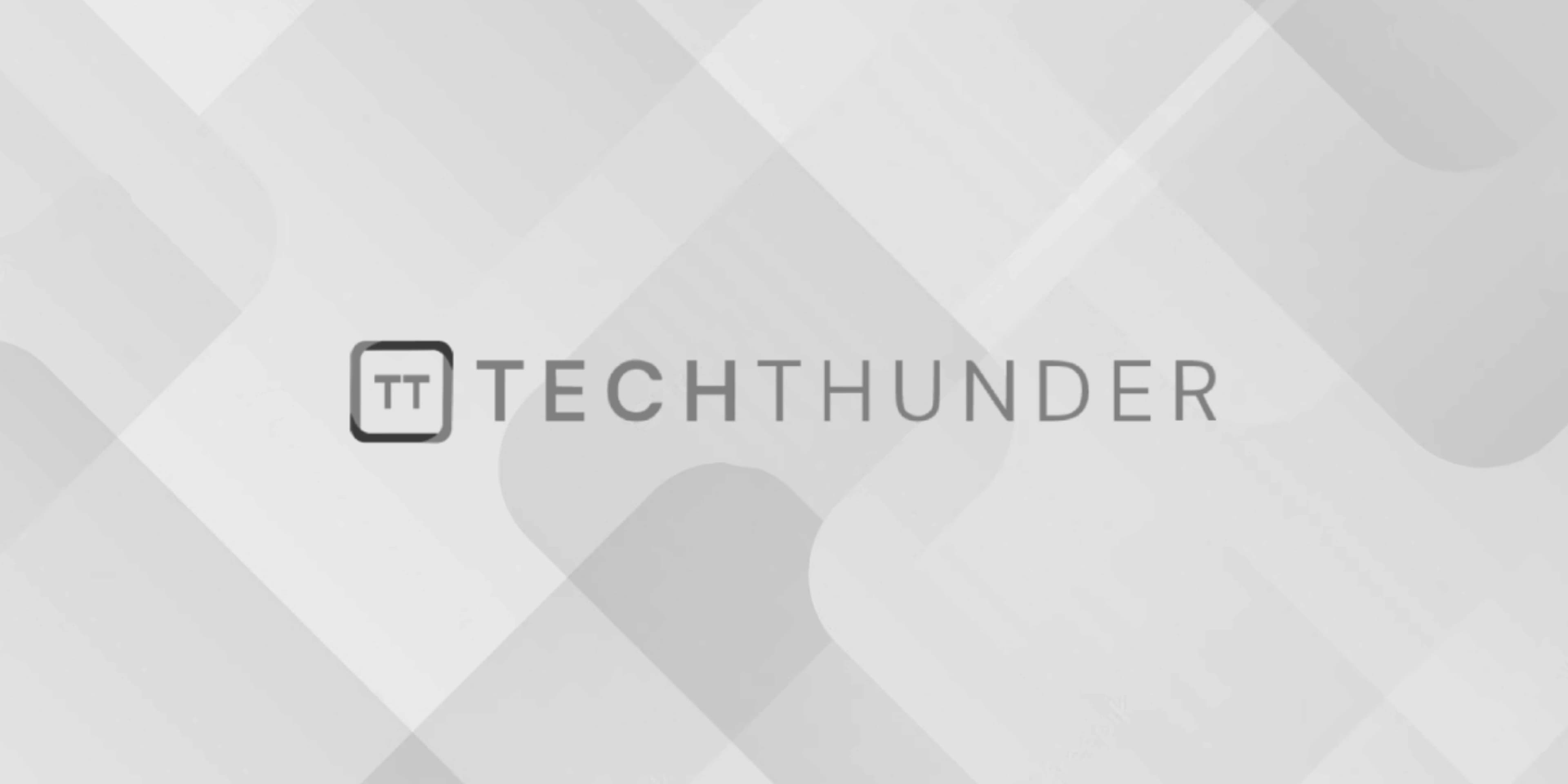
jQuery scrollLeft() method
The jQuery.scrollLeft()
method is used to get or set the horizontal scroll position of the first matched element. It allows you to determine the amount of horizontal scroll, or to scroll the element horizontally to a specified position.
Here’s the basic syntax of the scrollLeft()
method:
$(selector).scrollLeft()
Parameters:
selector
: The selector expression used to select the element whose scroll position will be retrieved or set.
Return Value:
The scrollLeft()
method returns the horizontal scroll position of the first matched element.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery scrollLeft() Method Example</title>
<style>
.scrollable {
width: 200px;
height: 100px;
overflow: auto;
border: 1px solid #ccc;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="scrollable">
<table>
<tr>
<td>Cell 1</td>
<td>Cell 2</td>
<!-- Add more cells to create horizontal scrollbar -->
</tr>
</table>
</div>
<script>
$(document).ready(function() {
// Get the horizontal scroll position of the first matched element
var scrollPosition = $(".scrollable").scrollLeft();
// Log the horizontal scroll position
console.log("Horizontal Scroll Position:", scrollPosition);
});
</script>
</body>
</html>
In this example, we have a <div>
element with the class “scrollable” that contains a table. The table is wider than the div, so it creates a horizontal scrollbar. We use the scrollLeft()
method to get the horizontal scroll position of the div.
If you scroll the div horizontally, the output of the above example will change based on the position you scrolled to.
To set the horizontal scroll position of the element, you can pass a value as an argument to the scrollLeft()
method:
$(".scrollable").scrollLeft(value);
Replace value
with the desired horizontal scroll position in pixels.
The scrollLeft()
method is useful when you need to work with elements that have overflow and need to determine or set the horizontal scroll position. It is commonly used when dealing with scrollable containers, such as divs with overflow: auto
or overflow: scroll
.