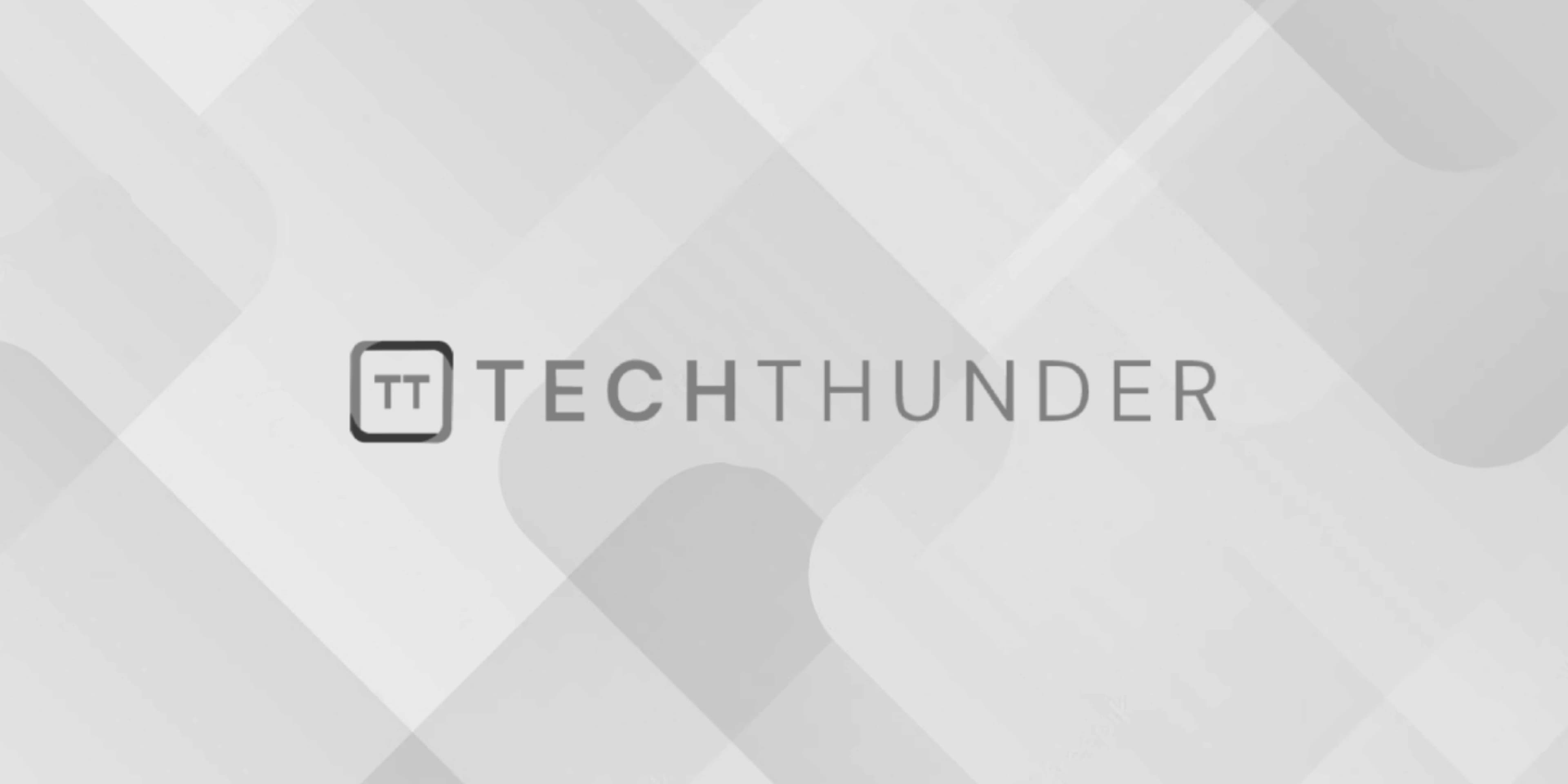
jQuery event.preventDefault() method
The event.preventDefault()
method in jQuery is used to prevent the default action of an event from occurring. When you bind an event handler to an element, some events have default behaviors associated with them. For example, clicking on a link (<a>
element) normally navigates to the linked URL, and submitting a form (<form>
element) typically triggers a form submission and reloads the page.
By using event.preventDefault()
inside the event handler function, you can stop the default behavior from happening, allowing you to define custom behavior for the event.
Here’s the basic syntax of the event.preventDefault()
method:
$(selector).on('event', function(event) {
event.preventDefault();
// Custom event handling code goes here
});
Parameters:
selector
: The selector for the element to which the event handler is attached.event
: The event object that represents the event that occurred.
Return Value:
The event.preventDefault()
method does not return a value.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery event.preventDefault() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<a href="https://www.example.com" id="myLink">Click Me</a>
<script>
$(document).ready(function() {
$("#myLink").on('click', function(event) {
event.preventDefault(); // Prevent the default link navigation behavior
alert("Link clicked, but default navigation prevented!");
});
});
</script>
</body>
</html>
In this example, we have a hyperlink (<a>
element) with the ID myLink
. When the link is clicked, the click
event is triggered. We use event.preventDefault()
inside the event handler function to prevent the default behavior of the link, which is to navigate to the URL specified in the href
attribute. Instead, an alert message will be shown, indicating that the default navigation behavior was prevented.
event.preventDefault()
is particularly useful when you want to add custom behavior to events without interfering with their default actions. For example, you might want to perform form validation before submitting a form or display a custom modal instead of navigating to a linked URL.
Keep in mind that the event.preventDefault()
method only prevents the default action of the event within the event handler function. Other event handlers or event propagation may still execute as usual unless you also stop the event propagation using event.stopPropagation()
or return false
from the event handler function (which has the same effect as calling both event.preventDefault()
and event.stopPropagation()
).