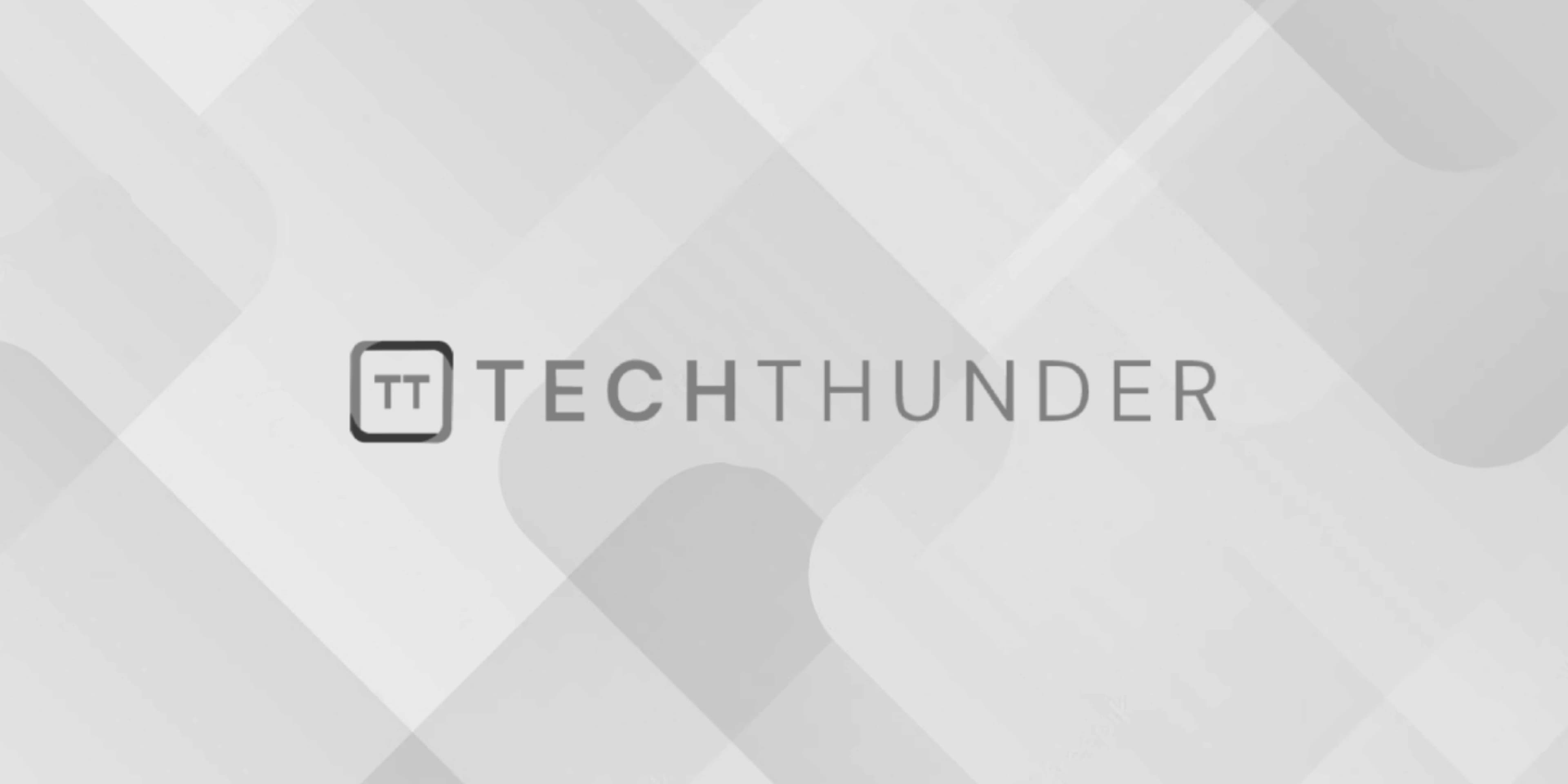
jQuery isPlainObject() method
The jQuery.isPlainObject()
method in jQuery is a utility function used to check whether an object is a plain JavaScript object or not. A plain JavaScript object is an object that is created with the object literal syntax {}
or with the new Object()
constructor and does not inherit from a custom prototype or other non-native objects.
Here’s the basic syntax of the jQuery.isPlainObject()
method:
jQuery.isPlainObject(obj)
Parameters:
obj
: The object to be tested.
Return Value:
The jQuery.isPlainObject()
method returns true
if the specified object is a plain JavaScript object, and false
if the object is not a plain JavaScript object.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery isPlainObject() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<script>
$(document).ready(function() {
var plainObject = { name: "John", age: 30 };
var nonPlainObject = Object.create({});
// Check if the objects are plain JavaScript objects
var isPlain1 = jQuery.isPlainObject(plainObject);
var isPlain2 = jQuery.isPlainObject(nonPlainObject);
// Log the results to the console
console.log("plainObject is a plain object:", isPlain1); // true
console.log("nonPlainObject is a plain object:", isPlain2); // false
});
</script>
</body>
</html>
In this example, we have two objects: plainObject
and nonPlainObject
. The plainObject
is a plain JavaScript object created with the object literal syntax {}
. The nonPlainObject
, on the other hand, is created using Object.create({})
, which inherits from an empty object and is not a plain JavaScript object.
We use the jQuery.isPlainObject()
method to check whether each of these objects is a plain JavaScript object or not. The method returns true
for the plainObject
because it is a plain JavaScript object, and false
for the nonPlainObject
because it is not a plain JavaScript object.
Keep in mind that the jQuery.isPlainObject()
method is useful in cases where you want to differentiate between plain JavaScript objects and objects that may inherit from custom prototypes or non-native objects. If you only want to check if an object is a plain object without considering its prototype chain, you can use the following modern JavaScript approach:
Object.prototype.toString.call(obj) === '[object Object]'
Using Object.prototype.toString.call(obj) === '[object Object]'
provides a reliable way to identify plain objects in modern JavaScript environments without relying on the jQuery utility.