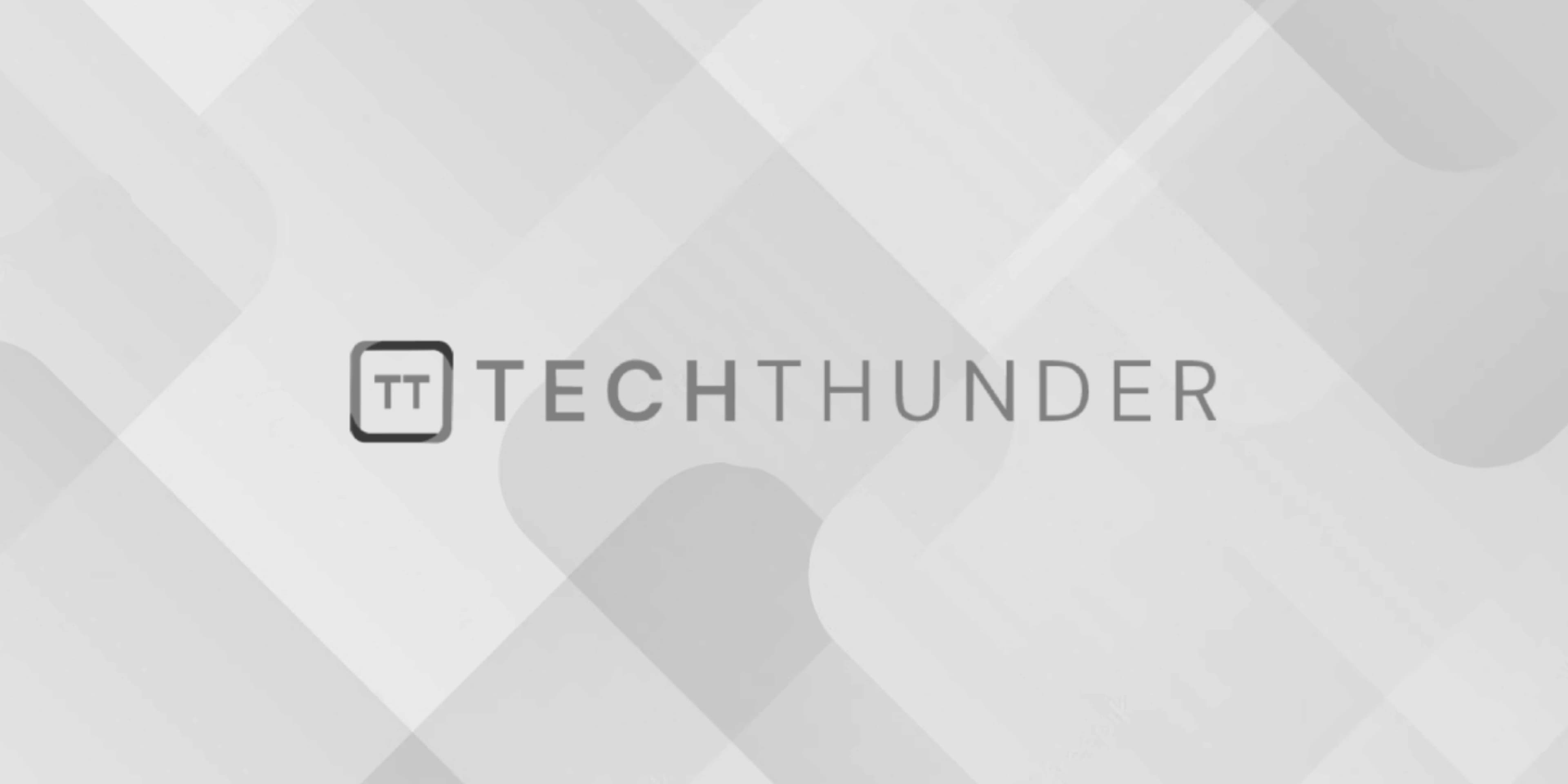
jQuery add() method
The add()
method in jQuery is used to add elements, sets of elements, or jQuery objects to an existing jQuery selection. This method does not modify the original selection but returns a new jQuery object containing the combined elements.
Here’s the basic syntax of the add()
method:
$(selector1).add(selector2)
Parameters:
selector1
: The initial jQuery selection.selector2
: The selector or DOM element(s) to be added to the selection.
Return Value:
The add()
method returns a new jQuery object that includes the elements from both selector1
and selector2
.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery add() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
.selected {
background-color: yellow;
}
</style>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
$(document).ready(function() {
var $selectedItems = $("li").eq(0); // Select the first <li> element
// Use add() to add the third <li> element to the selection
$selectedItems = $selectedItems.add($("li").eq(2));
// Add a class to the selected items
$selectedItems.addClass("selected");
});
</script>
</body>
</html>
In this example, we have an unordered list with three <li>
elements. We use the add()
method to select the first <li>
element and then add the third <li>
element to the selection. The selected items are given a yellow background by adding the selected
class to them.
Please note that the add()
method does not modify the original jQuery selection ($selectedItems
in this case); instead, it returns a new jQuery object that includes the elements from both the original selection and the added elements. This is useful for combining multiple selections or adding elements to an existing selection without affecting the original one.