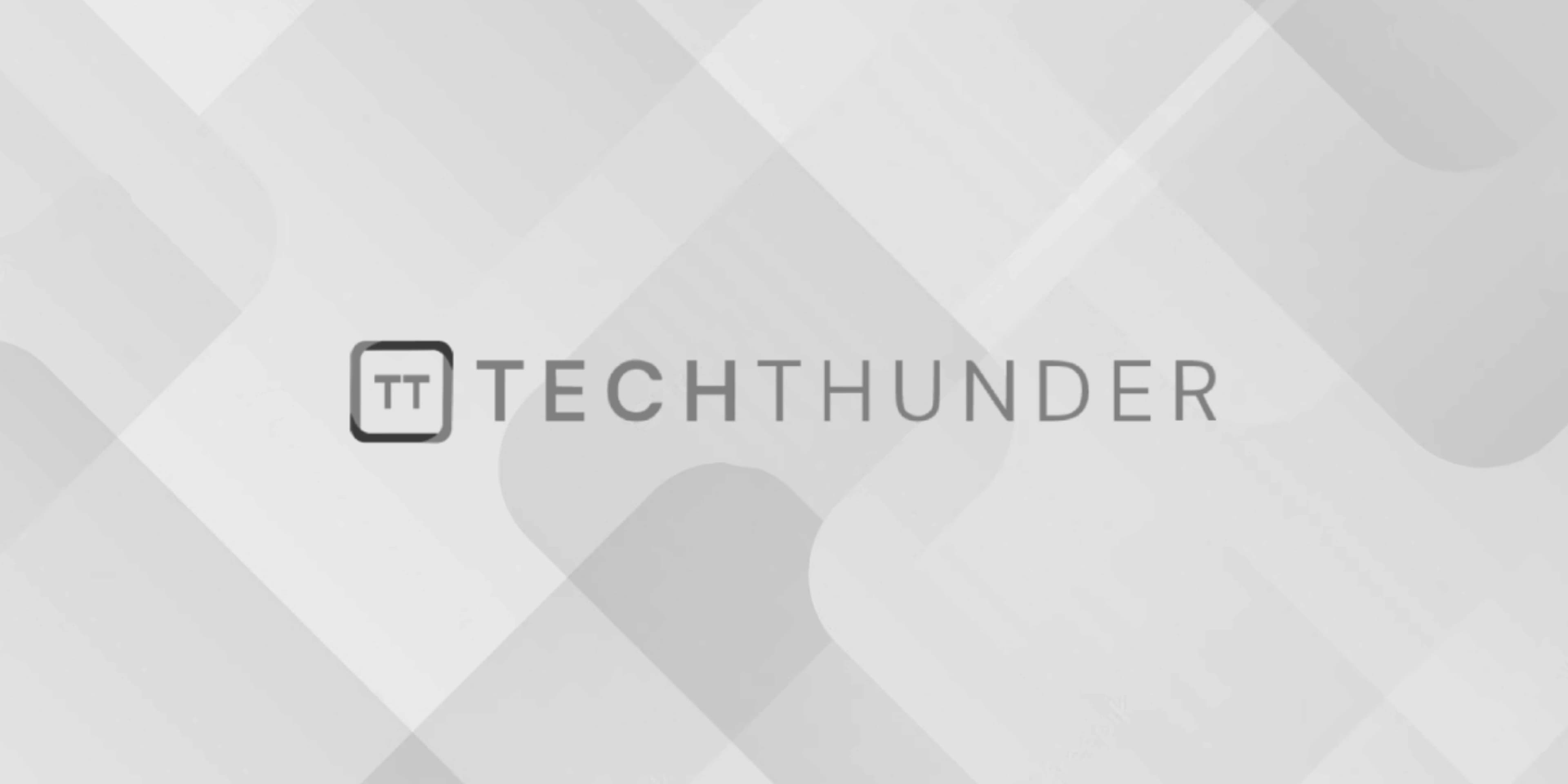
jQuery parents() method
The jQuery.parents()
method is used to select all the ancestor elements of the selected elements in a DOM hierarchy. An ancestor element is a parent, grandparent, great-grandparent, and so on, of the selected elements, moving upwards in the DOM tree.
Here’s the basic syntax of the parents()
method:
$(selector).parents([filter])
Parameters:
selector
: Optional. A selector expression used to filter the ancestor elements. If provided, only the ancestors that match the selector will be selected.filter
: Optional. A selector expression used to further filter the selected ancestors.
Return Value:
The parents()
method returns a jQuery object containing the selected ancestor elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery parents() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="grandparent">
<div class="parent">
<div class="child">
Some content here.
</div>
</div>
</div>
<script>
$(document).ready(function() {
// Select all ancestors of the .child element
var ancestors = $(".child").parents();
// Log the number of selected ancestors and their HTML content
console.log("Number of ancestors:", ancestors.length);
ancestors.each(function(index) {
console.log("Ancestor " + index + ":", $(this).html());
});
});
</script>
</body>
</html>
In this example, we have a DOM hierarchy with three nested <div>
elements: grandparent, parent, and child. We use the parents()
method to select all the ancestors of the element with the class “child” (in this case, the parent and grandparent). The method returns a jQuery object containing these ancestor elements.
The output of the above example will be:
Number of ancestors: 2
Ancestor 0: <div class="parent">
<div class="child">
Some content here.
</div>
</div>
Ancestor 1: <div class="grandparent">
<div class="parent">
<div class="child">
Some content here.
</div>
</div>
</div>
In this output, we see that there are two ancestor elements selected: the parent and the grandparent of the original element with class “child.” The ancestors are listed in the order in which they appear in the DOM tree, starting from the immediate parent and moving up the hierarchy.
If you provide a selector
or filter
argument to the parents()
method, it will only select the ancestors that match the specified selector or filter. For example:
var filteredAncestors = $(".child").parents(".parent");
This will select only the parent of the element with class “child” and exclude the grandparent.