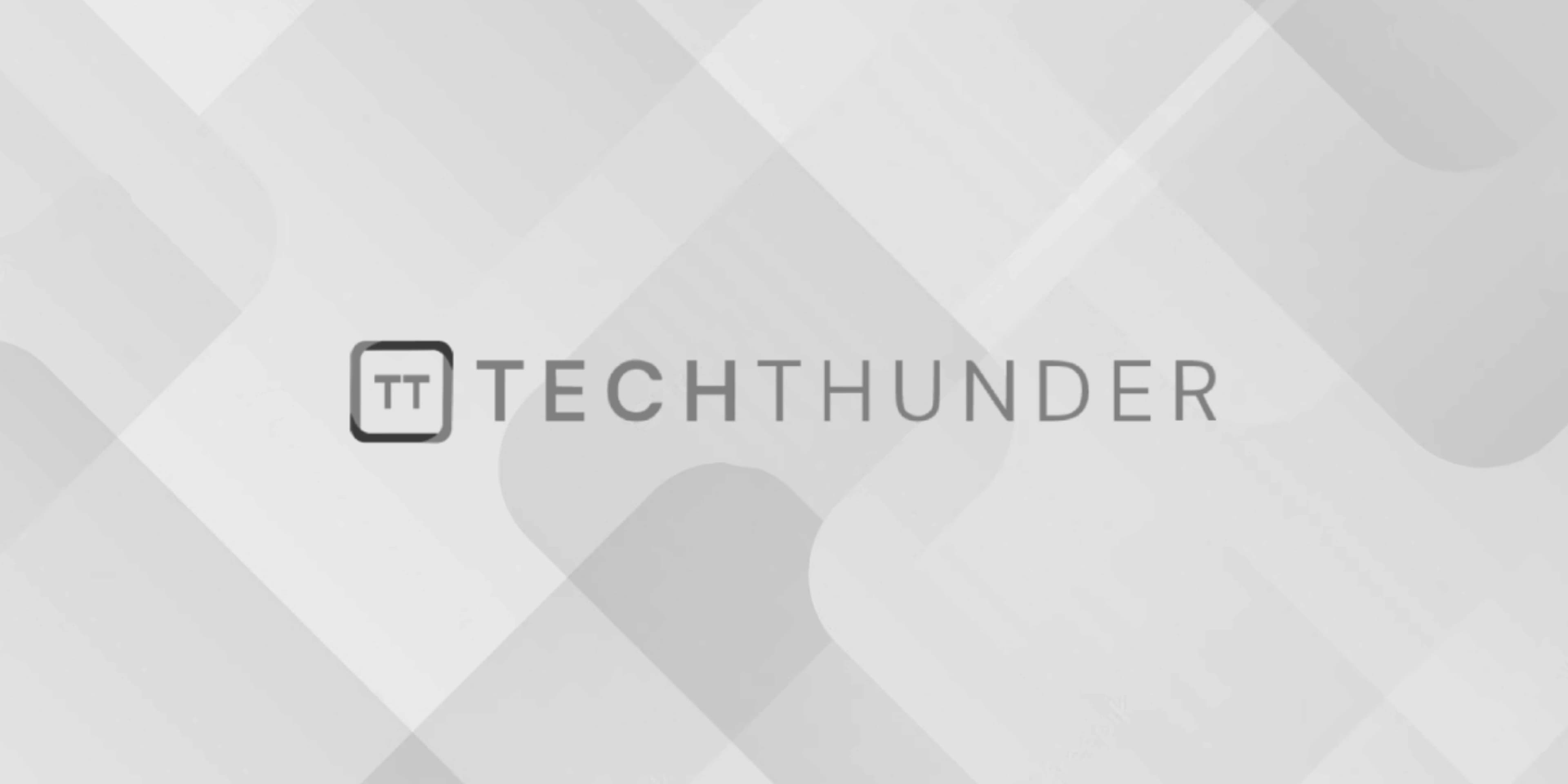
JQuery UI Control groups
The jQuery UI control groups provide a way to visually group related form elements together, such as checkboxes, radio buttons, or buttons, into a single visually connected unit. This grouping can improve the user experience and make it clear that these elements are related.
To create control groups in jQuery UI, you’ll need to include the jQuery UI library along with the jQuery library in your HTML file. Then, you can use the controlgroup()
method to create control groups for your form elements.
Here’s a basic example of creating control groups for checkboxes and radio buttons:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>jQuery UI Control Groups</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="control-group">
<label>Checkbox Group:</label>
<div>
<input type="checkbox" id="checkbox1">
<label for="checkbox1">Option 1</label>
<input type="checkbox" id="checkbox2">
<label for="checkbox2">Option 2</label>
<input type="checkbox" id="checkbox3">
<label for="checkbox3">Option 3</label>
</div>
</div>
<div class="control-group">
<label>Radio Button Group:</label>
<div>
<input type="radio" name="radio-group" id="radio1">
<label for="radio1">Option 1</label>
<input type="radio" name="radio-group" id="radio2">
<label for="radio2">Option 2</label>
<input type="radio" name="radio-group" id="radio3">
<label for="radio3">Option 3</label>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 0;
}
.control-group {
max-width: 400px;
margin: 50px auto;
padding: 20px;
border: 2px solid #ccc;
border-radius: 8px;
}
label {
font-weight: bold;
display: block;
margin-bottom: 10px;
}
input[type="checkbox"],
input[type="radio"] {
margin-right: 5px;
}
In this example, we have two control groups: one for checkboxes and one for radio buttons. We use the controlgroup()
method provided by jQuery UI to style and group these form elements.
JavaScript (script.js):
$(document).ready(function() {
$('.control-group div').controlgroup();
});
The controlgroup()
method is called on the div
elements inside each control group to apply the control group styling. This method formats the form elements and their labels to make them visually connected.
When you open the HTML file in your browser, you will see the checkboxes and radio buttons organized in control groups with labels. The control group styling enhances the visual appearance and layout of the form elements.