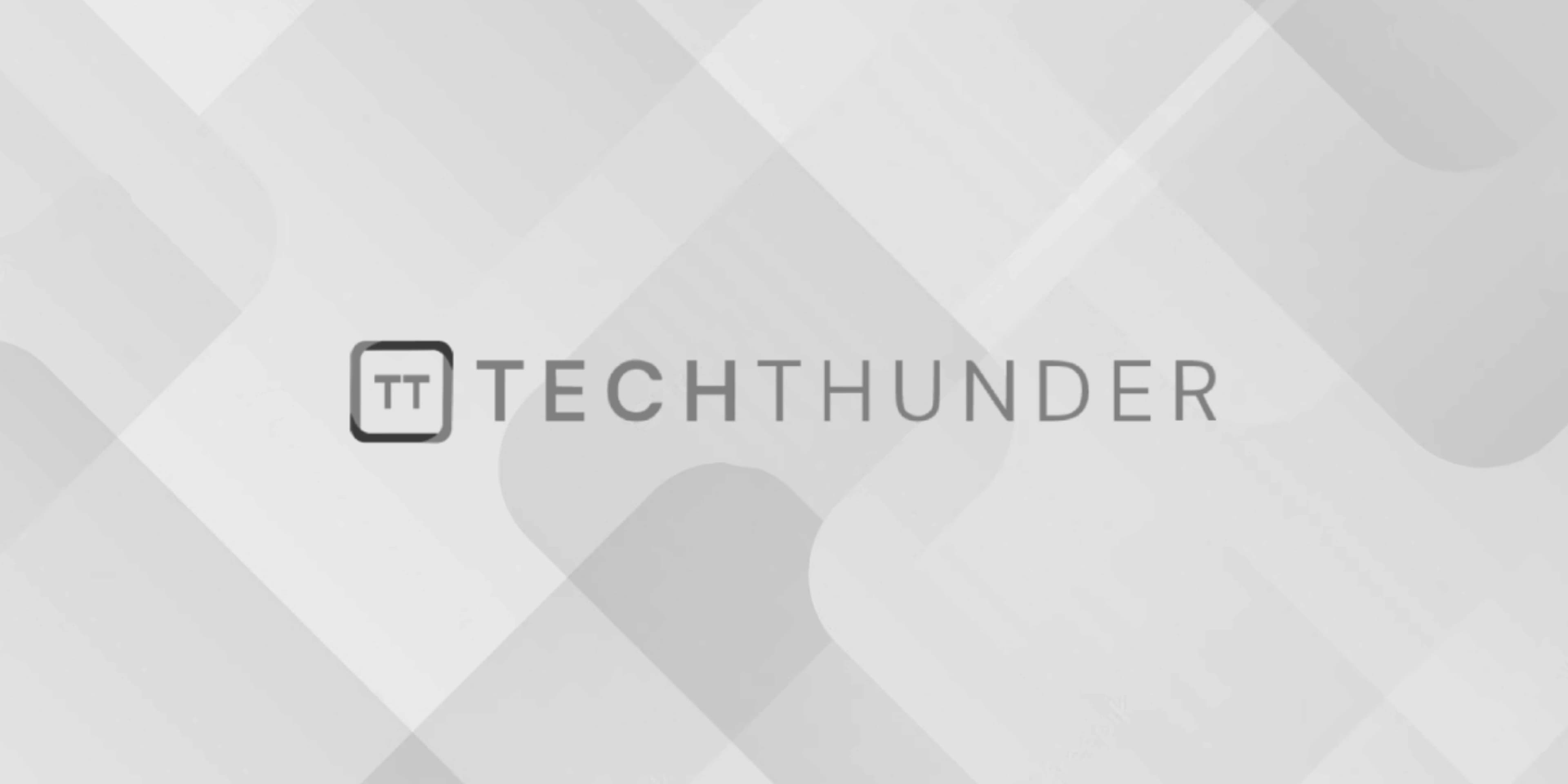
210 views
Get Selected Value in Drop-down in jQuery
To get the selected value from a drop-down list using jQuery, you can use the .val()
method. Here’s a step-by-step guide on how to do this:
Assuming you have the following HTML for your drop-down list:
<select id="myDropdown">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
You can use the following jQuery code to get the selected value:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
// Select the drop-down element by its ID
var selectedValue = $("#myDropdown").val();
// Log the selected value to the console
console.log("Selected value: " + selectedValue);
});
</script>
In this example, we:
- Include the jQuery library.
- Use
$(document).ready()
to ensure that the script is executed after the document is fully loaded. - Select the drop-down element using its ID, which is “myDropdown.”
- Use the
.val()
method to get the selected value and store it in theselectedValue
variable. - Log the selected value to the console using
console.log()
.
It will log the selected value from the drop-down list to the browser’s console. You can then use the selectedValue
variable as needed in your JavaScript code.