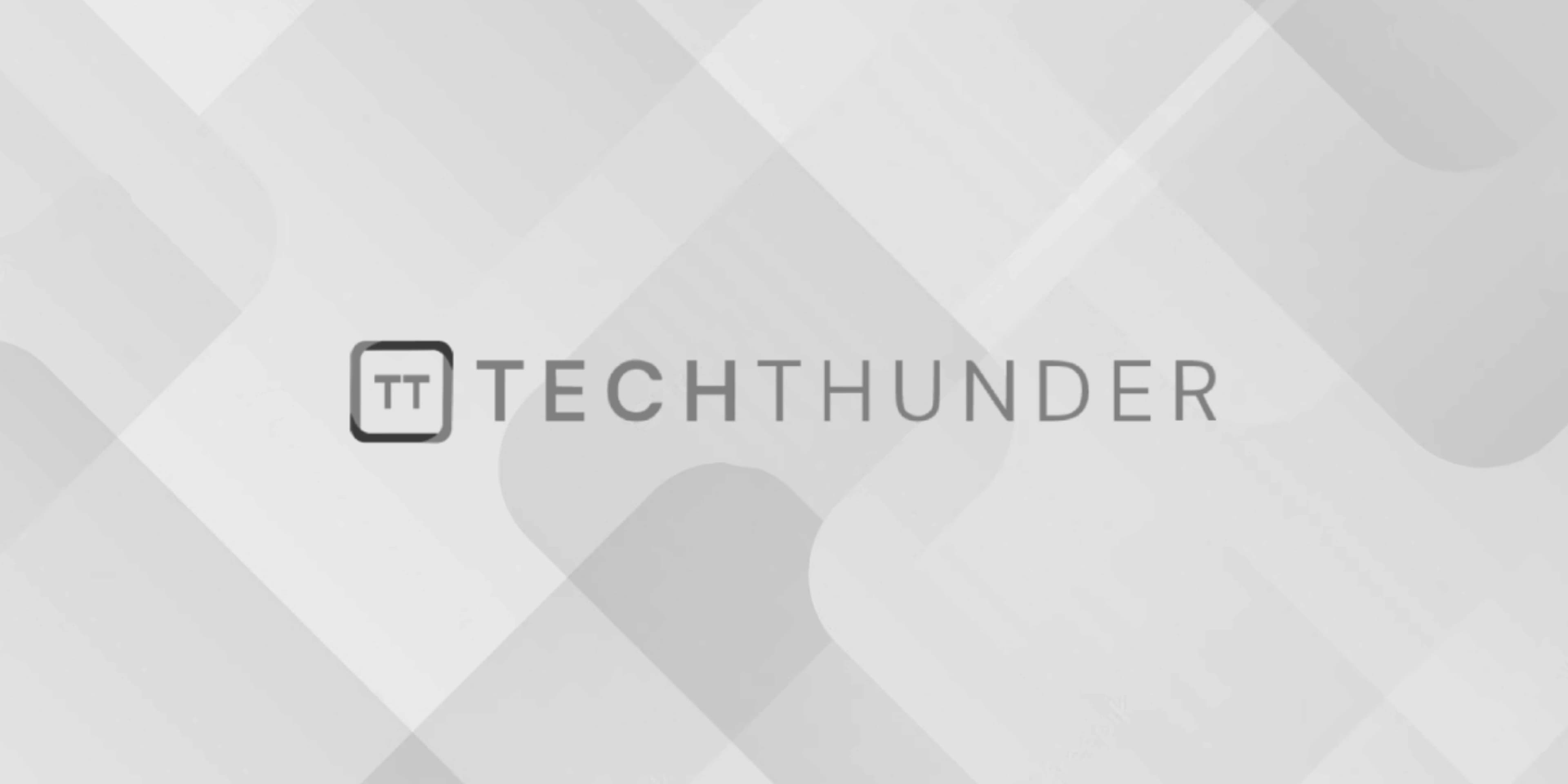
jQuery andBack() method
The addBack()
method is used to add the previous set of elements to the current set of matched elements in a jQuery object. This method allows you to include both the original set of elements and the elements from the previous step in the method chaining.
Here’s the basic syntax of the addBack()
method:
$(selector).addBack([filter])
Parameters:
selector
: The selector for the elements to add to the current set.filter
(optional): A string representing a filter expression to further narrow down the selection.
Return Value:
The addBack()
method returns a new jQuery object containing the combined set of elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery addBack() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
div {
width: 100px;
height: 100px;
background-color: red;
margin: 10px;
}
.highlight {
border: 2px solid blue;
}
</style>
</head>
<body>
<div class="box"></div>
<div class="box"></div>
<div class="box"></div>
<button id="highlightFirst">Highlight First Box</button>
<button id="highlightAll">Highlight All Boxes</button>
<script>
$(document).ready(function() {
function highlightFirstBox() {
$(".box").first().addClass("highlight");
}
function highlightAllBoxes() {
$(".box").addBack().addClass("highlight");
}
$("#highlightFirst").click(function() {
highlightFirstBox();
});
$("#highlightAll").click(function() {
highlightAllBoxes();
});
});
</script>
</body>
</html>
In this example, we have three red squares (<div>
elements) with the class “box.” There are two buttons: “Highlight First Box” and “Highlight All Boxes.” When the “Highlight First Box” button is clicked, the highlightFirstBox()
function is called, which uses the first()
method to select the first box and adds the class “highlight” to it, giving it a blue border. When the “Highlight All Boxes” button is clicked, the highlightAllBoxes()
function is called, which uses the addBack()
method to add the original set of boxes back to the selection and adds the class “highlight” to all the boxes, giving them all blue borders.
As shown in the example, addBack()
can be useful when you want to include the previously selected elements back into the current selection, allowing you to apply changes to both the new and original sets of elements.