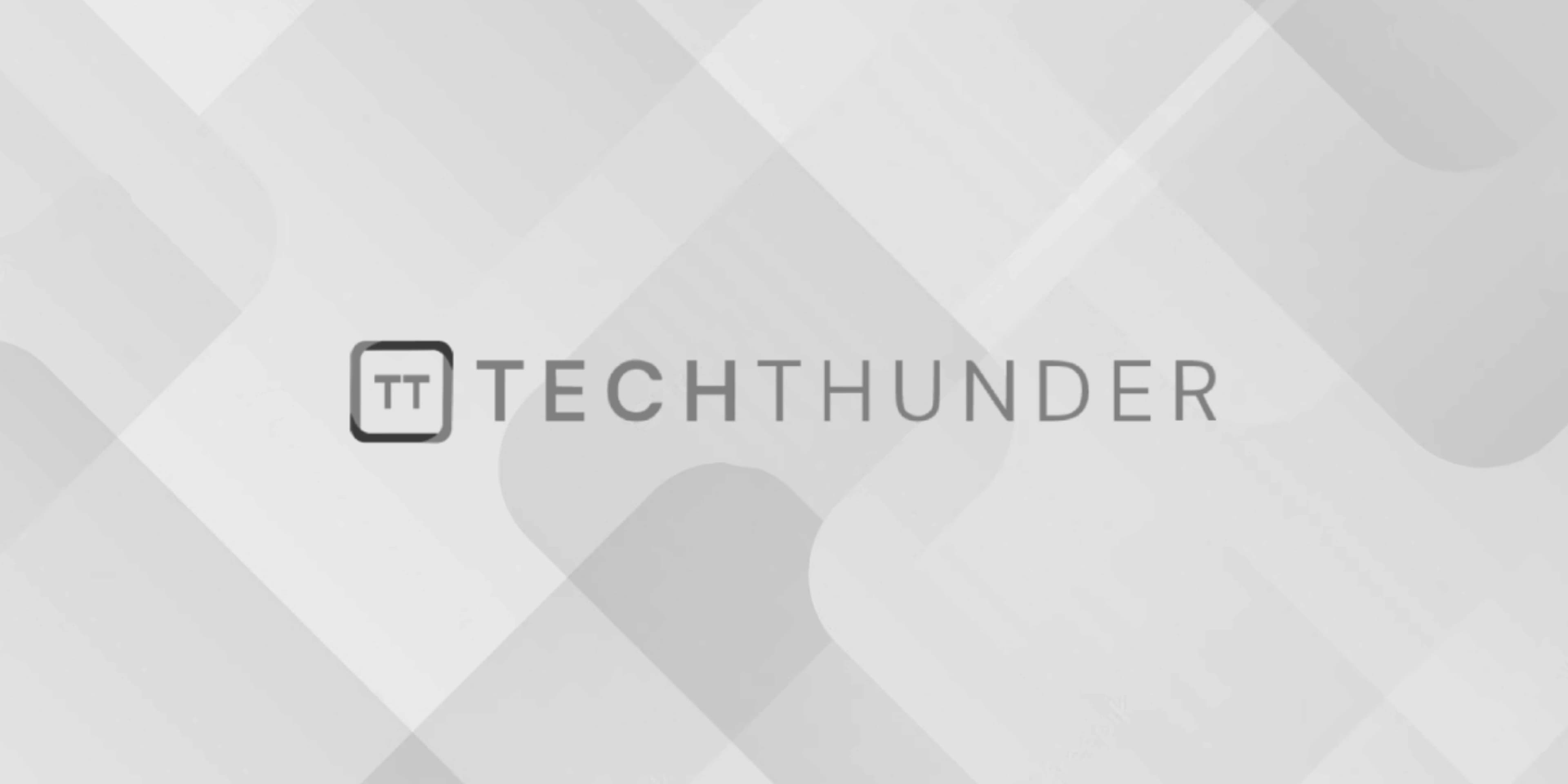
Upload image with progress bar using jQuery
To upload an image with a progress bar using jQuery, you can use the HTML5 File API along with jQuery to handle the file input and display the progress. Below is a step-by-step guide on how to achieve this:
Step 1: Set Up HTML Structure
Create an HTML form with a file input for image selection and a progress bar to display the upload progress.
<!DOCTYPE html>
<html>
<head>
<title>Upload Image with Progress Bar</title>
</head>
<body>
<form id="uploadForm">
<input type="file" name="imageFile" id="imageFile">
<button type="submit">Upload</button>
</form>
<progress id="uploadProgress" value="0" max="100"></progress>
</body>
</html>
Step 2: Include jQuery
Include the jQuery library in your HTML file using a CDN or download and link it locally.
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 3: Handle the Form Submission
Use jQuery to handle the form submission and initiate the image upload. We’ll also update the progress bar as the upload progresses.
$(document).ready(function() {
// Handle form submission
$('#uploadForm').submit(function(e) {
e.preventDefault(); // Prevent default form submission
var formData = new FormData(this);
$.ajax({
url: 'upload.php', // Replace with the URL to your server-side upload script
type: 'POST',
data: formData,
processData: false,
contentType: false,
xhr: function() {
var xhr = new window.XMLHttpRequest();
xhr.upload.addEventListener('progress', function(evt) {
if (evt.lengthComputable) {
var percentComplete = (evt.loaded / evt.total) * 100;
$('#uploadProgress').val(percentComplete);
}
}, false);
return xhr;
},
success: function(response) {
// Handle the server response after the upload is complete
console.log(response);
},
error: function(xhr) {
// Handle error
console.error('Error occurred during upload:', xhr.status);
}
});
});
});
Step 4: Handle the Server-Side Upload (PHP example)
On the server-side, you need to handle the actual file upload. In this example, we’ll use PHP to handle the image upload. Create a PHP script (e.g., upload.php
) that handles the image upload and returns any relevant response.
<?php
if ($_FILES['imageFile']['error'] === UPLOAD_ERR_OK) {
$targetDir = 'uploads/';
$targetFile = $targetDir . basename($_FILES['imageFile']['name']);
if (move_uploaded_file($_FILES['imageFile']['tmp_name'], $targetFile)) {
// File upload successful
echo 'File uploaded successfully.';
} else {
// File upload failed
echo 'Error occurred during file upload.';
}
} else {
// File upload error
echo 'Error: ' . $_FILES['imageFile']['error'];
}
?>
Remember to replace 'upload.php'
with the actual URL to your server-side upload script and adjust the server-side code accordingly based on your server’s setup and requirements.
Now, when you select an image using the file input and click the “Upload” button, the image will be uploaded to the server, and the progress bar will show the upload progress. The server-side script (upload.php) will handle the actual upload and respond with a success or error message.
Always ensure that your server-side upload script properly handles file uploads and performs necessary security checks to prevent unauthorized access or malicious file uploads.