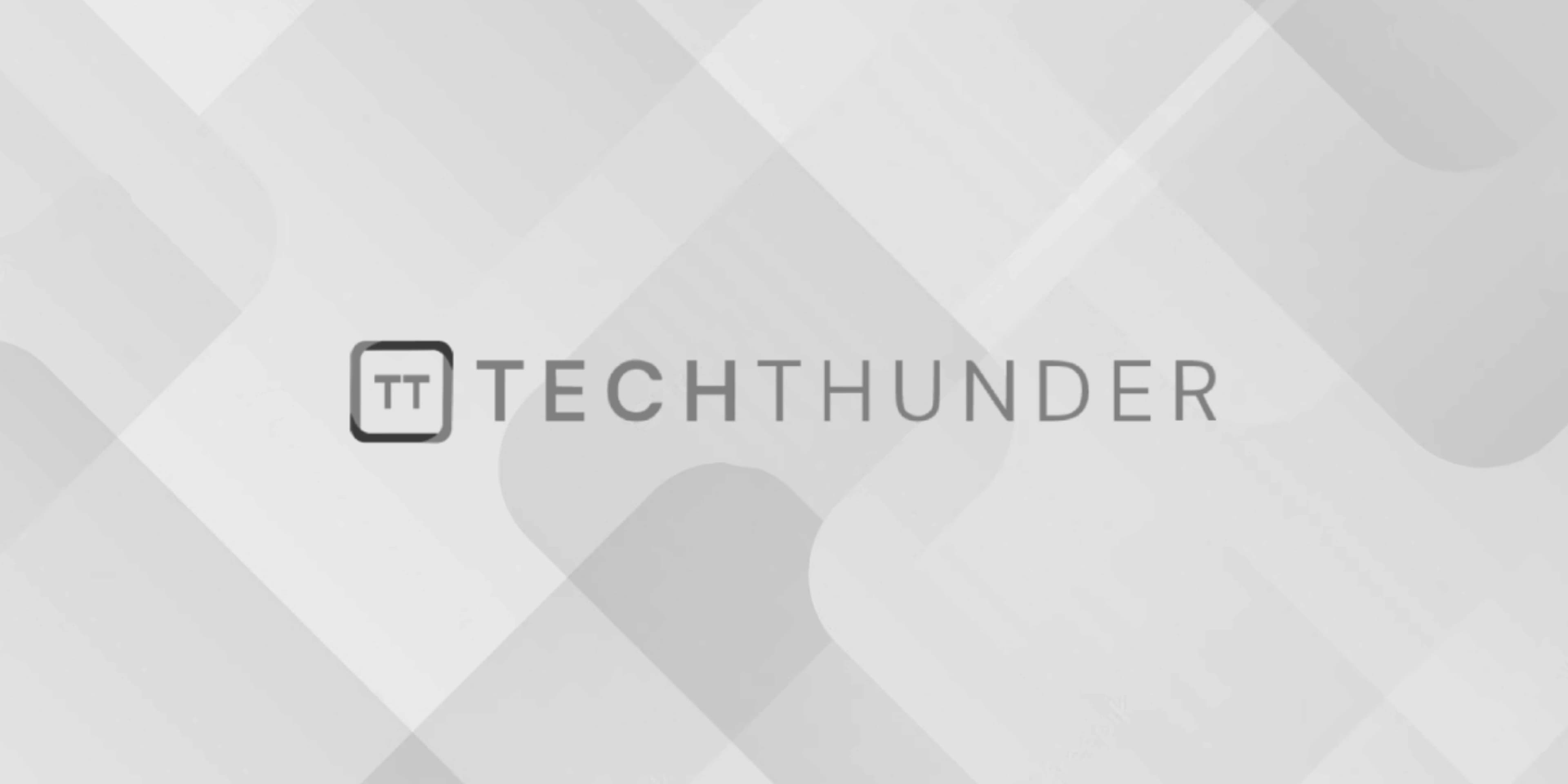
jQuery ajaxError() method
The jQuery ajaxError()
method is an event handler that is triggered when an Ajax request encounters an error. It allows you to attach a function that will be executed whenever an Ajax request fails or encounters an error, such as a network issue, server error, or invalid response.
Here’s the basic syntax of the ajaxError()
method:
$(document).ajaxError(function(event, jqxhr, settings, thrownError) {
// Code to be executed when an Ajax request encounters an error
});
Parameters:
event
: The jQuery event object.jqxhr
: The jQuery XHR (XMLHttpRequest) object representing the failed Ajax request.settings
: The settings object used for the Ajax request (e.g., URL, type, data, etc.).thrownError
: The textual portion of the HTTP status, or an error string representing the error if the request failed due to an error on the server.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery ajaxError() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="sendRequest">Send Ajax Request</button>
<script>
$(document).ready(function() {
// Attach ajaxError event handler
$(document).ajaxError(function(event, jqxhr, settings, thrownError) {
console.log("Ajax request failed with error: " + thrownError);
});
// Click event handler for the button
$("#sendRequest").on("click", function() {
// Simulate an Ajax request that will encounter an error
$.ajax({
url: "https://nonexistentapi.example.com/data",
method: "GET",
success: function(data) {
console.log("Ajax request completed successfully.");
},
error: function(jqxhr, textStatus, errorThrown) {
console.log("Ajax request failed with error: " + textStatus);
}
});
});
});
</script>
</body>
</html>
In this example, we have a button with the ID “sendRequest.” When the button is clicked, an Ajax request is made using $.ajax()
. The request is intentionally made to a non-existent URL to trigger an error. When the request fails, the ajaxError()
event handler is triggered, and the error message is logged to the console.
Note: The ajaxError()
event is triggered for any Ajax request that encounters an error, whether it is a network error, a server error (e.g., HTTP status codes in the 4xx or 5xx range), or any other error. The event handler is executed globally for all Ajax errors on the page.
The ajaxError()
method is useful when you want to handle errors that occur during Ajax requests globally and perform consistent error handling or logging for your application. It allows you to react to failed requests in a centralized manner rather than having to handle errors individually for each Ajax call.