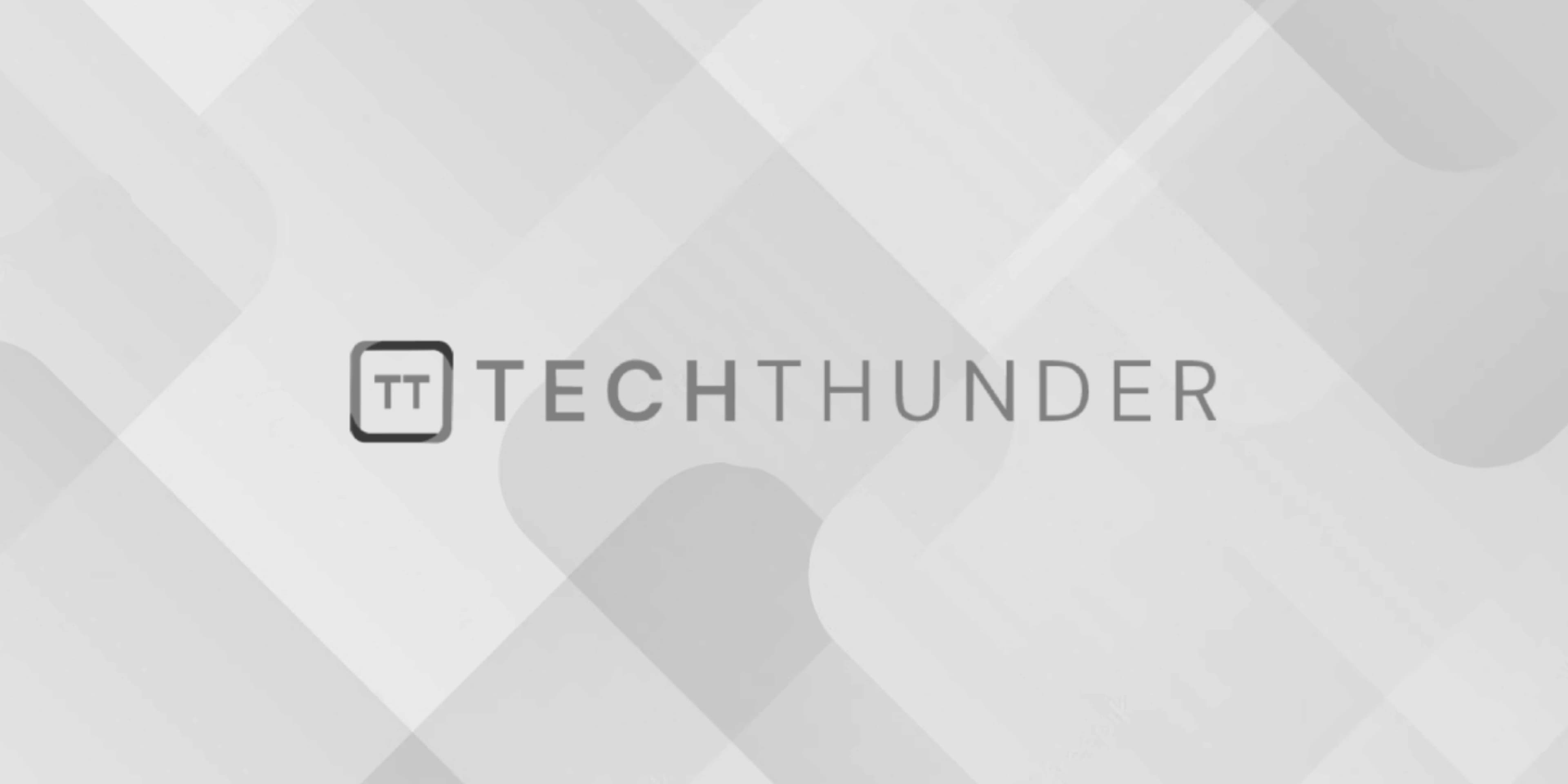
JQuery UI Checkbox Radio
The jQuery UI provides a set of CSS classes for form elements, including checkboxes and radio buttons, that allow you to customize their appearance.
Here’s an example of how to style checkboxes and radio buttons using jQuery UI:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Checkbox and Radio Styling with jQuery UI</title>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="styles.css">
</head>
<body>
<label for="checkbox">Checkbox:</label>
<input type="checkbox" id="checkbox">
<br>
<label for="radio1">Radio 1:</label>
<input type="radio" name="radio" id="radio1">
<label for="radio2">Radio 2:</label>
<input type="radio" name="radio" id="radio2">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</body>
</html>
CSS (styles.css):
/* Customize checkbox and radio styles using jQuery UI classes */
.ui-checkbox .ui-icon {
background-color: #fff; /* Checkbox background color */
border: 1px solid #ccc; /* Checkbox border color */
width: 16px; /* Checkbox width */
height: 16px; /* Checkbox height */
margin-right: 5px; /* Space between checkbox and label */
}
.ui-checkbox.ui-state-active .ui-icon {
background-color: #007bff; /* Checked checkbox background color */
border-color: #007bff; /* Checked checkbox border color */
}
.ui-radio .ui-icon {
background-color: #fff; /* Radio button background color */
border: 2px solid #ccc; /* Radio button border color */
border-radius: 50%; /* Make it circular */
width: 12px; /* Radio button width */
height: 12px; /* Radio button height */
margin-right: 5px; /* Space between radio button and label */
}
.ui-radio.ui-state-active .ui-icon {
background-color: #007bff; /* Selected radio button background color */
border-color: #007bff; /* Selected radio button border color */
}
We have included the necessary jQuery and jQuery UI CSS and JavaScript files. We’ve used the ui-checkbox
and ui-radio
classes provided by jQuery UI for styling the checkboxes and radio buttons. Additionally, we have defined custom CSS to adjust the appearance of the checkboxes and radio buttons.
When you run this code, you’ll see styled checkboxes and radio buttons. The checkboxes will have a square appearance, and the radio buttons will have a circular appearance. When you click on a checkbox or radio button, it will change color to indicate its active state.