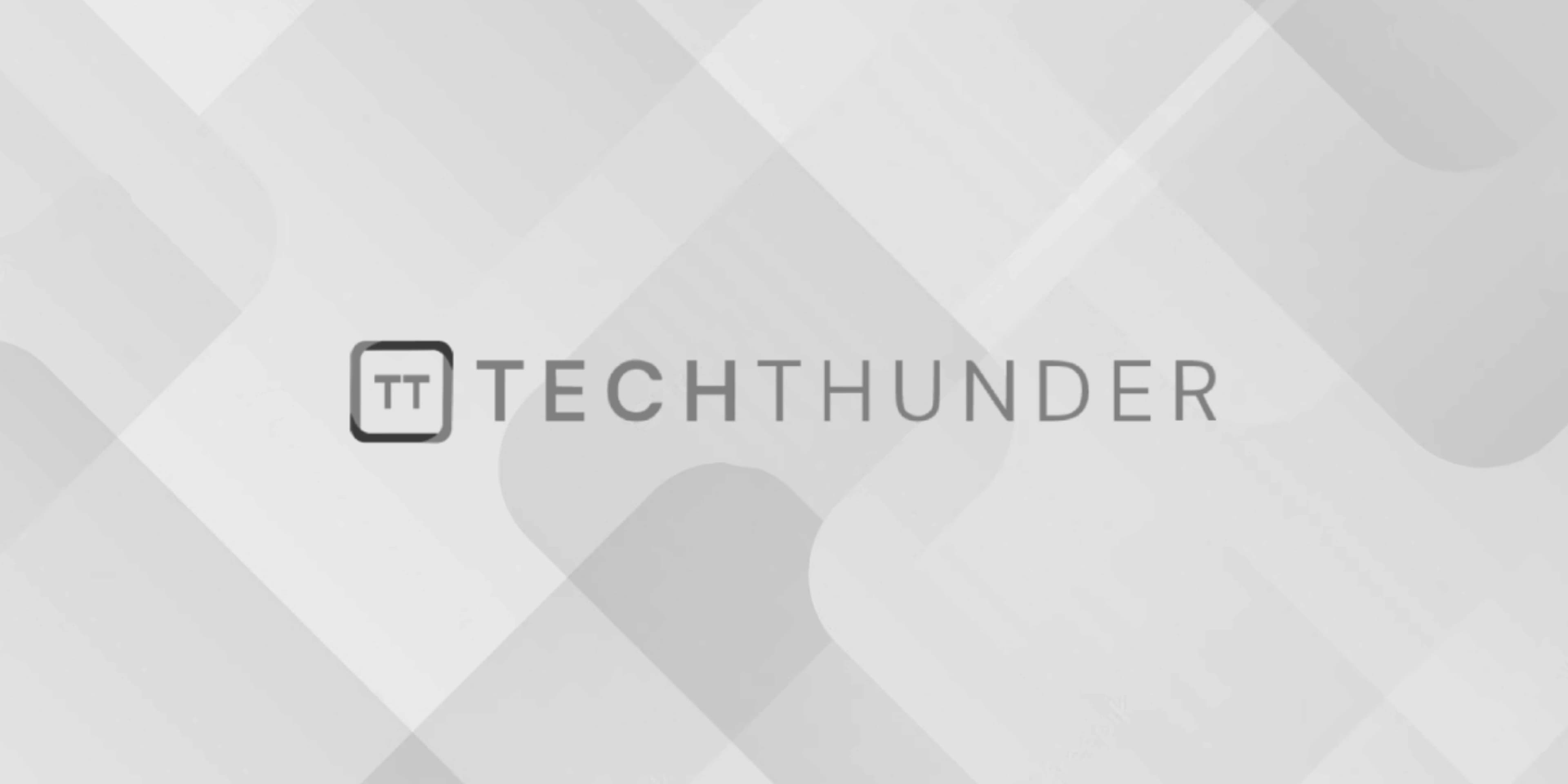
jQuery even() method
The even()
method in jQuery is used to select even-indexed elements from a set of matched elements. It filters the elements in the jQuery object and returns a new jQuery object containing only the elements with even index positions.
Here’s the basic syntax of the even()
method:
$(selector).even()
Parameters:
selector
: The selector for the elements to filter.
Return Value:
The even()
method returns a new jQuery object containing the elements with even index positions from the original set of matched elements. The index positions start from 0.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery even() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
li {
list-style: none;
margin: 5px;
}
.even {
background-color: lightblue;
}
</style>
</head>
<body>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
<script>
$(document).ready(function() {
// Select even-indexed <li> elements and add a class to them
$("li").even().addClass("even");
});
</script>
</body>
</html>
In this example, we have an unordered list with five list items (<li>
elements). We use the even()
method to select the even-indexed <li>
elements (i.e., “Item 1,” “Item 3,” and “Item 5”) and add a class called “even” to them. The “even” class applies a light blue background color to the selected elements.
Please note that the even()
method is zero-based, so it selects elements at index positions 0, 2, 4, and so on. Elements at odd index positions (1, 3, 5, etc.) are excluded from the resulting jQuery object.
As of jQuery version 3.4, the even()
method is marked as deprecated. Instead of using even()
, you can achieve the same result by using the filter()
method with a callback function:
$("li").filter(function(index) {
return index % 2 === 0;
}).addClass("even");
This will have the same effect as using even()
, and it provides a more flexible and extensible way to filter elements based on specific conditions.