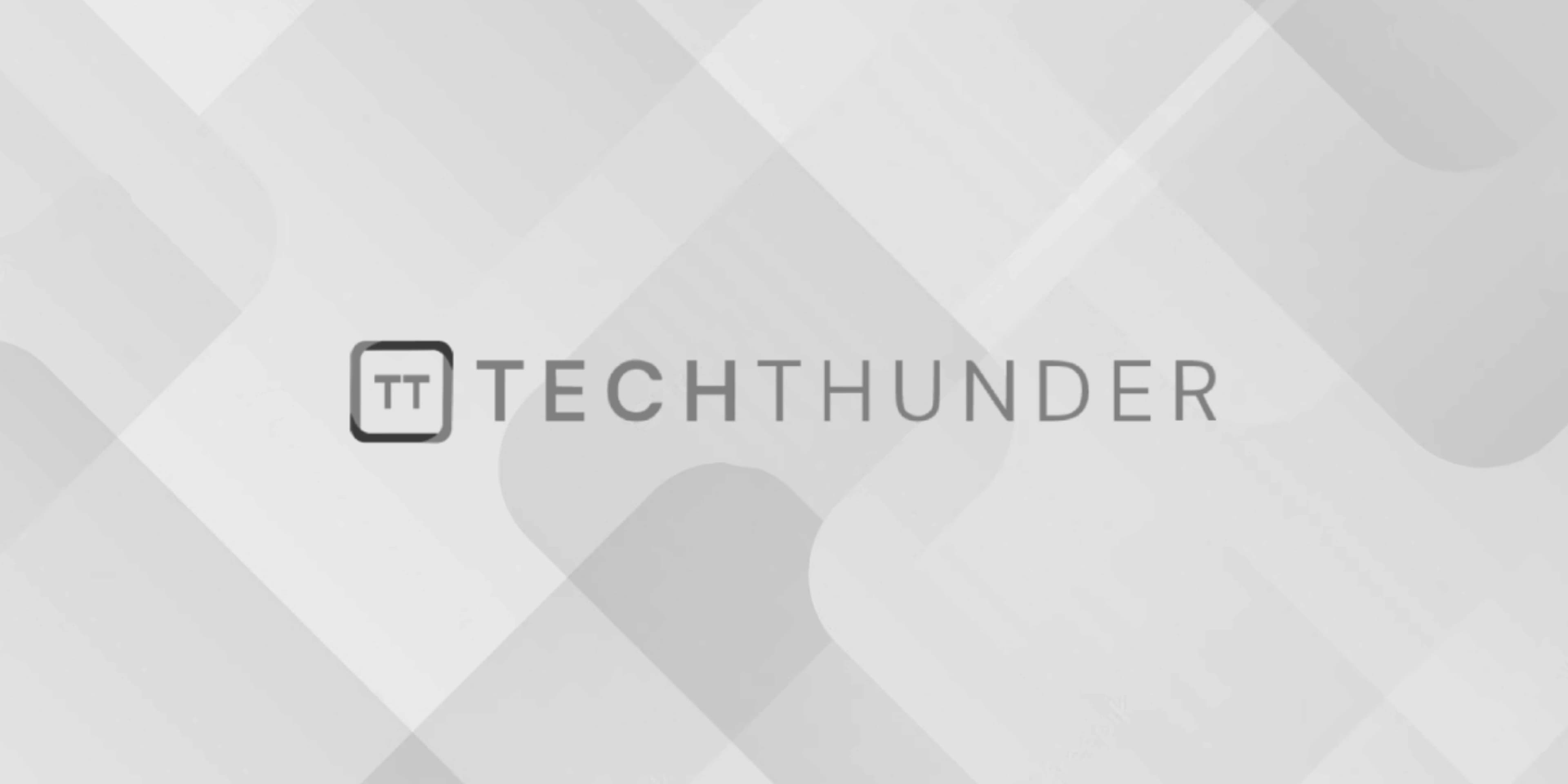
jQuery delegate() method
The jQuery delegate()
method was used for event delegation in earlier versions of jQuery. However, it has been deprecated since jQuery version 3.0, and the recommended way to achieve event delegation now is by using the on()
method with event delegation syntax.
In versions of jQuery prior to 3.0, the delegate()
method allowed you to attach event handlers to elements that are not yet present in the DOM at the time the handler is bound. It was particularly useful for dynamically added elements or elements that might be added in the future.
Here’s the syntax of the delegate()
method:
$(selector).delegate(childSelector, eventType, handler);
Parameters:
childSelector
: A selector string that specifies the child elements to which the event will be delegated.eventType
: The type of event (e.g., “click”, “mouseenter”, “keydown”, etc.).handler
: The function that will be executed when the event is triggered.
However, as mentioned earlier, the delegate()
method has been deprecated and replaced with the on()
method using the event delegation syntax. Here’s how you can achieve event delegation with the on()
method:
$(document).on(eventType, selector, handler);
Parameters:
eventType
: The type of event (e.g., “click”, “mouseenter”, “keydown”, etc.).selector
: A selector string that specifies the child elements to which the event will be delegated. It can be any valid CSS selector.handler
: The function that will be executed when the event is triggered.
By using the on()
method with event delegation, you can achieve the same functionality as the delegate()
method in a more consistent and recommended way.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Event Delegation with on() Method</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
// Deprecated delegate() method (jQuery < 3.0)
// $('#list').delegate('li', 'click', function() {
// alert($(this).text());
// });
// Recommended event delegation with on() method (jQuery >= 3.0)
$(document).on('click', '#list li', function() {
alert($(this).text());
});
</script>
</body>
</html>
In this example, we have a list (<ul>
) with three list items (<li>
). We use event delegation to attach a click event handler to the list items, even though they are not present in the DOM when the event handler is bound. When a list item is clicked, an alert will display its text.
Remember to use the on()
method with event delegation syntax for new projects or when updating your jQuery code to the latest version.