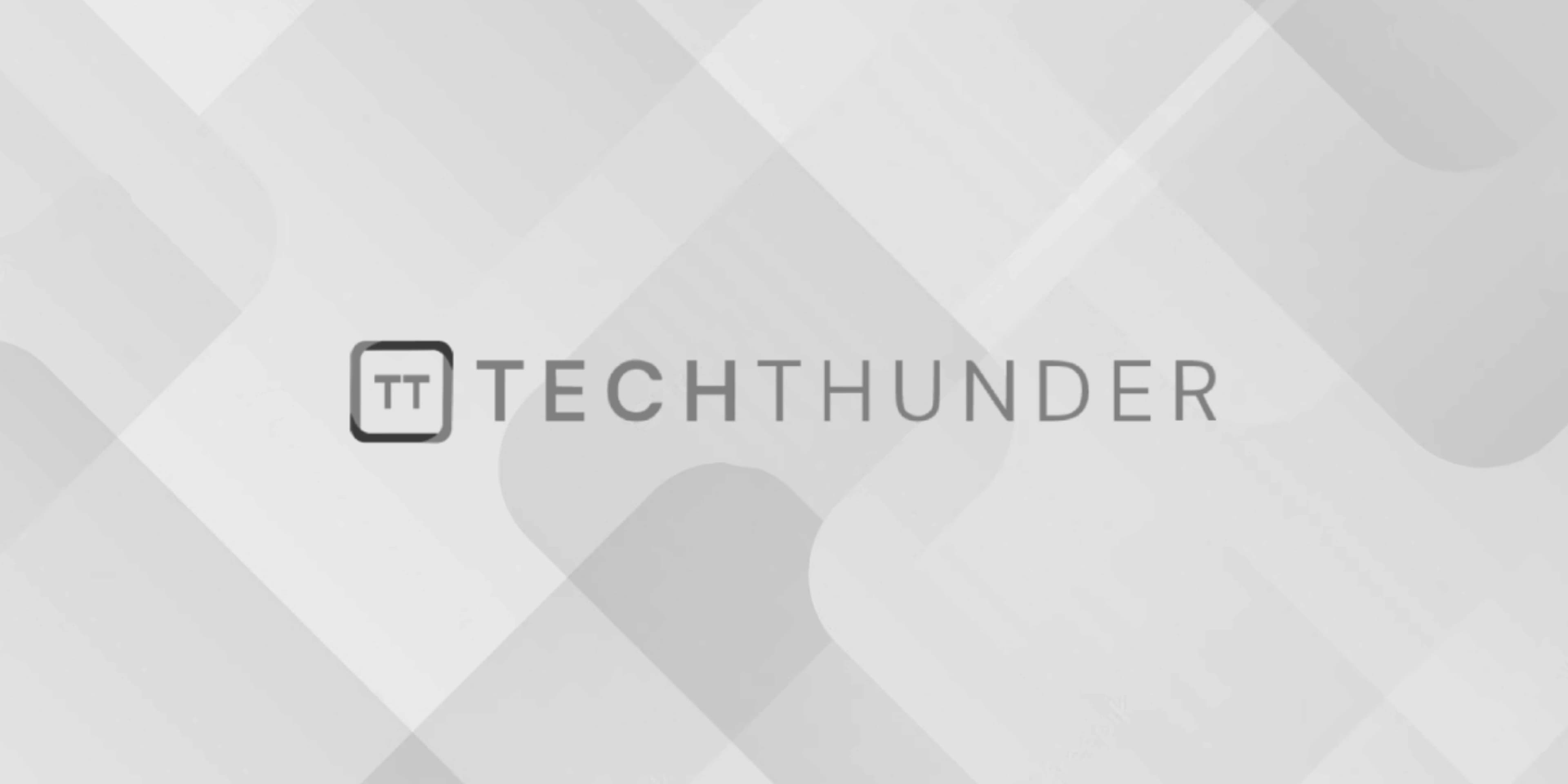
jQuery triggerHandler() method
The jQuery.triggerHandler()
method is used to trigger a specified event on the selected elements without executing the default behavior of the event or invoking any event handlers bound using jQuery.on()
or other event binding methods. This method is particularly useful when you want to manually trigger an event and handle the event logic without triggering any associated behaviors or event handlers.
Here’s the basic syntax of the triggerHandler()
method:
$(selector).triggerHandler(eventType [, extraParameters])
Parameters:
selector
: The selector expression for the elements on which the event will be triggered.eventType
: The type of event to be triggered (e.g., “click”, “mouseover”, etc.).extraParameters
: Optional. An array of additional parameters to pass to the event handler.
Return Value:
The triggerHandler()
method returns the value returned by the last event handler executed during the triggering process. If no event handlers were executed, it returns undefined
.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery triggerHandler() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
$(document).ready(function() {
// Attach a click event handler using .on() method
$("#myButton").on("click", function(event) {
console.log("Button clicked! Default behavior prevented.");
event.preventDefault();
});
// Trigger the click event using triggerHandler()
var returnValue = $("#myButton").triggerHandler("click");
// Output the return value (undefined since the default behavior was prevented)
console.log("Return value:", returnValue);
});
</script>
</body>
</html>
In this example, we attach a click event handler to the button with the ID “myButton” using the jQuery.on()
method. The event handler prevents the default behavior of the click event by calling event.preventDefault()
.
Next, we use the triggerHandler()
method to manually trigger the click event on the button. The event handler is executed, but the default behavior (navigation to a new page in this case) is prevented. The return value of the triggerHandler()
method is undefined
since no other event handlers are executed during the triggering process.
Keep in mind that the triggerHandler()
method only triggers the event on the first matched element in the jQuery object, if multiple elements are selected. If you want to trigger the event on all selected elements, you can use the each()
method to iterate over the elements and call triggerHandler()
for each one.
It’s essential to note that triggerHandler()
is different from trigger()
, which triggers the event and executes all event handlers and default behaviors associated with it. Use triggerHandler()
when you need to manually trigger an event for programmatic purposes and handle the event logic without invoking other event handlers.