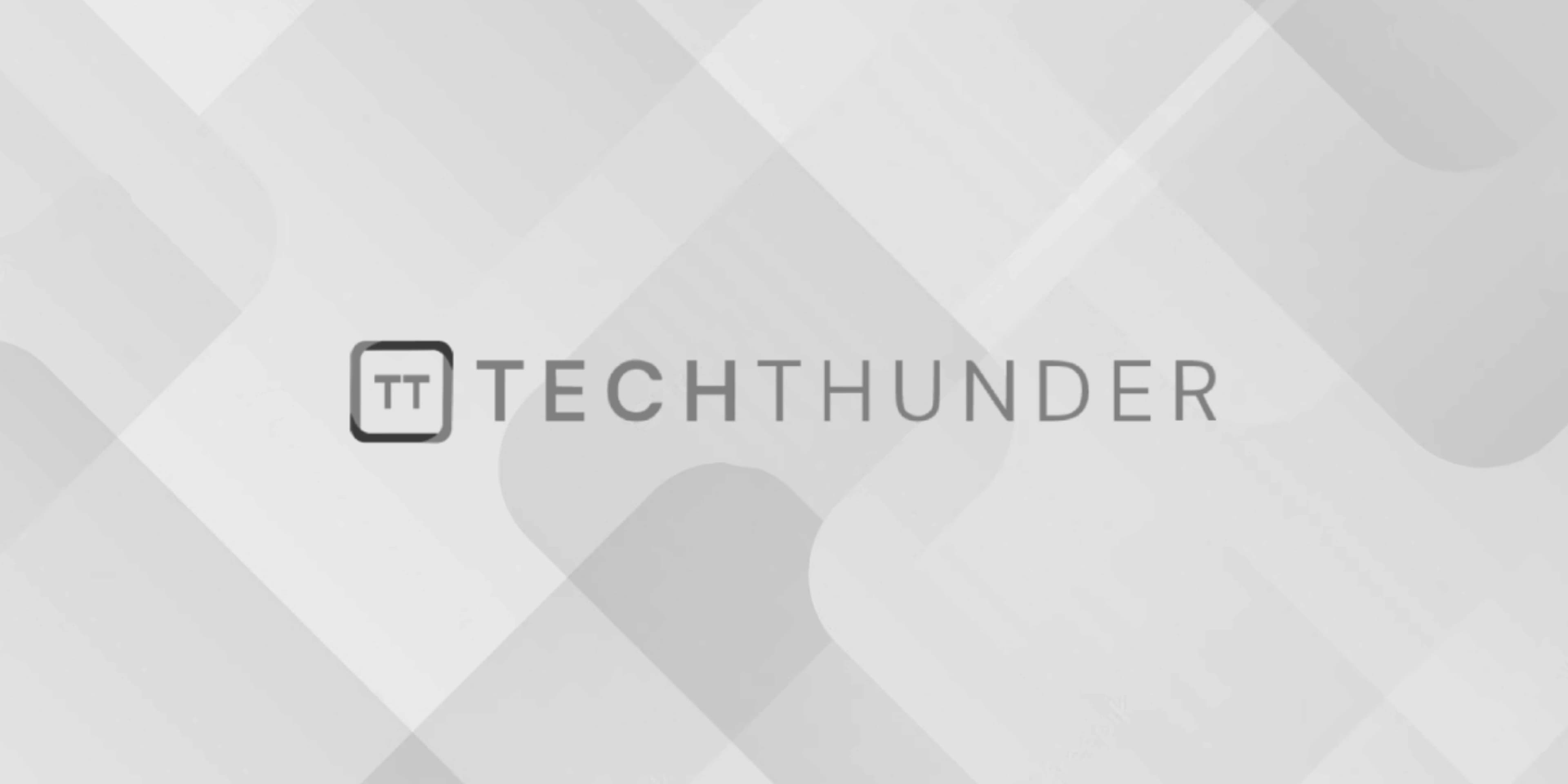
jQuery trim() method
The jQuery trim()
method is used to remove leading and trailing white spaces (including spaces, tabs, and line breaks) from a string. It operates similarly to the native JavaScript trim()
method, but it can be used on jQuery objects as well.
Here’s the basic syntax of the trim()
method:
$.trim(string)
Parameters:
string
: The string to be trimmed.
Return Value:
The trim()
method returns the trimmed version of the input string.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery trim() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p id="text"> Hello, this is a sample text. </p>
<script>
$(document).ready(function() {
var textContent = $("#text").text(); // Get the text content of the paragraph
var trimmedText = $.trim(textContent); // Trim the text content
// Display the original and trimmed text
console.log("Original Text: '" + textContent + "'");
console.log("Trimmed Text: '" + trimmedText + "'");
});
</script>
</body>
</html>
In this example, we have a paragraph (<p>
) element with some leading and trailing white spaces in the text. We use the $.trim()
method to remove those white spaces and obtain the trimmed version of the text. The original and trimmed text are then displayed in the console.
When you run the code, you will see that the leading and trailing white spaces in the original text are removed in the trimmed text.
The trim()
method is useful when you need to clean up user input, especially when handling form data, to ensure that no unwanted spaces are included in the input. It is commonly used to sanitize and normalize strings before further processing.