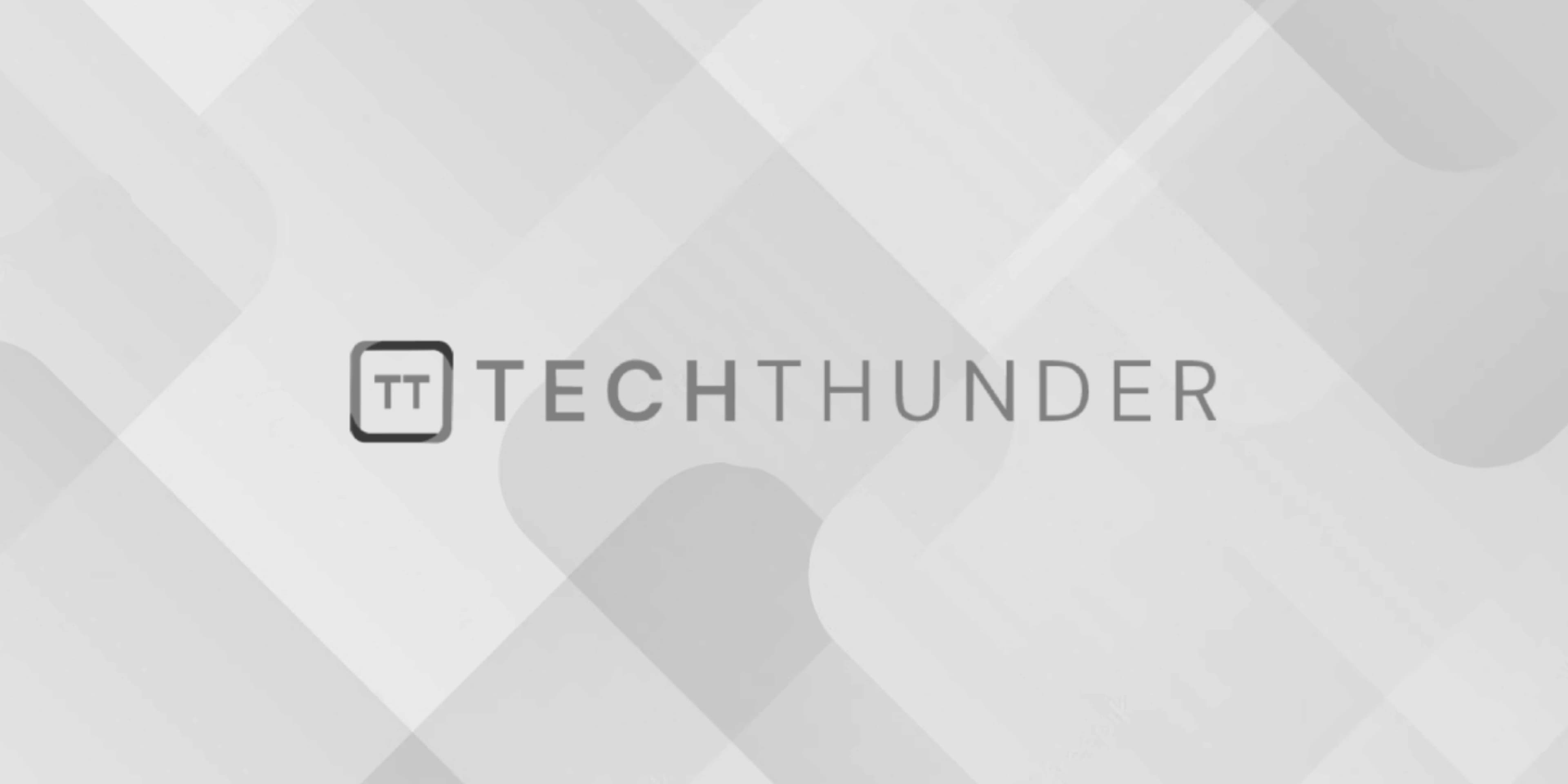
jQuery stop() method
The jQuery stop()
method is used to stop animations or queued animations on selected elements. It allows you to halt the current animation in progress, clear the animation queue, or both.
Here’s the basic syntax of the stop()
method:
$(selector).stop(clearQueue, jumpToEnd)
Parameters:
clearQueue
: A boolean value that determines whether to clear the animation queue. If set totrue
, all remaining animations in the queue for the selected elements will be removed. If set tofalse
or not provided, the queue will not be cleared, and the animations will continue after the current one stops. This parameter is optional.jumpToEnd
: A boolean value that determines whether to jump to the end of the animation. If set totrue
, the current animation will immediately complete and jump to its end state. If set tofalse
or not provided, the animation will stop at its current position. This parameter is optional.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery stop() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="box"></div>
<button id="animateButton">Animate</button>
<button id="stopButton">Stop Animation</button>
<script>
$(document).ready(function() {
var $box = $("#box");
// Function to animate the box
function animateBox() {
$box.animate({ left: "200px" }, 2000);
}
// Animate the box when the "Animate" button is clicked
$("#animateButton").click(function() {
animateBox();
});
// Stop the animation when the "Stop Animation" button is clicked
$("#stopButton").click(function() {
$box.stop();
});
});
</script>
<style>
#box {
width: 50px;
height: 50px;
background-color: red;
position: relative;
}
</style>
</body>
</html>
In this example, we have a red box (<div>
element) that can be animated to move to the right by clicking the “Animate” button. The “Stop Animation” button is used to stop the animation immediately when clicked, or it can be used to clear the animation queue.
When you click the “Animate” button, the box will start moving to the right. If you click the “Stop Animation” button while the box is moving, the animation will stop immediately, and the box will remain at its current position. If you click the “Animate” button multiple times rapidly, the box will move to the right multiple times in a sequence. However, if you click the “Stop Animation” button before the animations complete, the queue will be cleared, and the box will stop at its current position.
The stop()
method is useful when you want to control animations on elements and manage their behavior. It can be helpful to prevent animations from stacking up or to provide more interactive control over animations on user interaction.