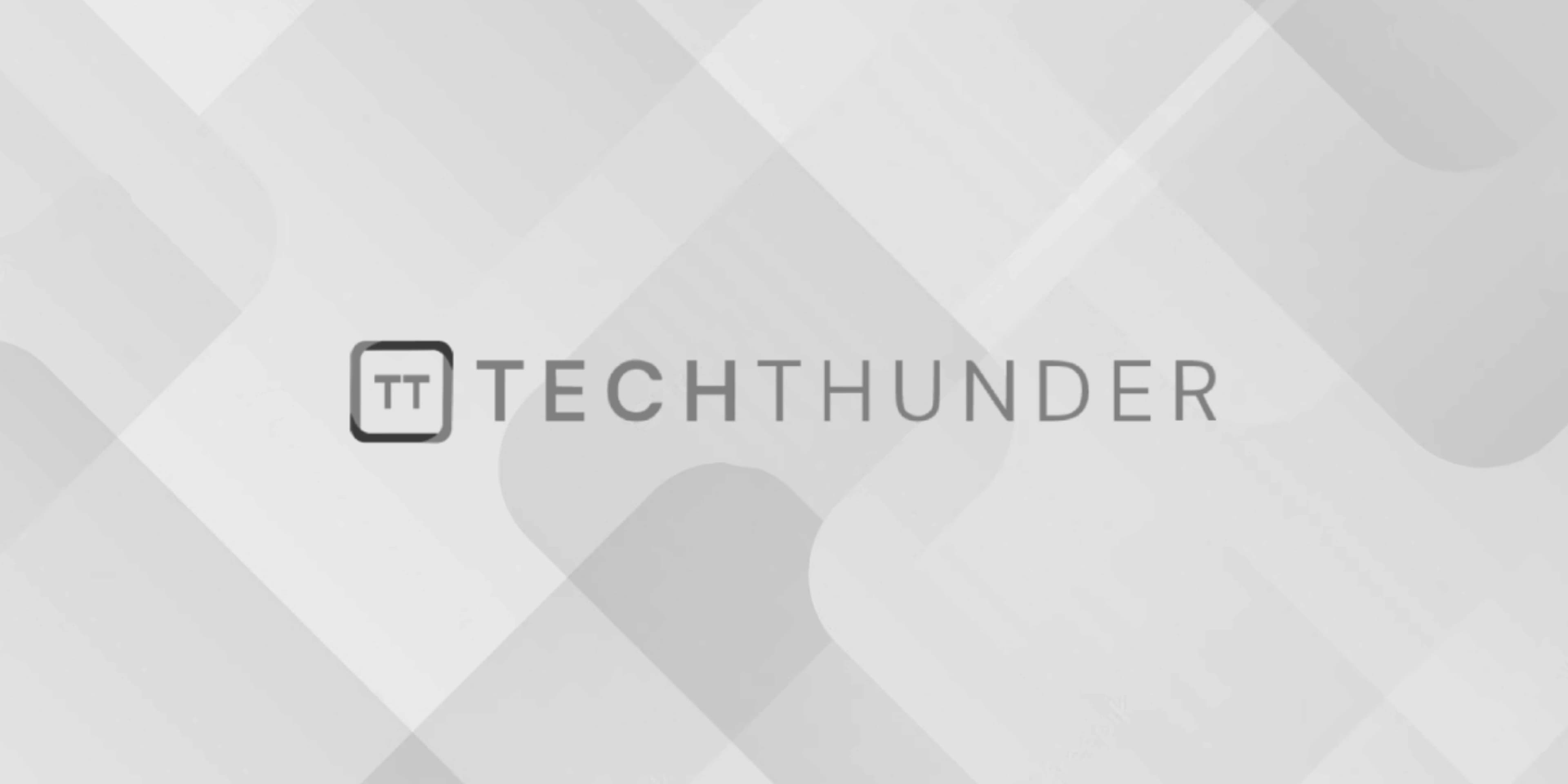
jQuery trigger() method
The jQuery trigger()
method is used to programmatically trigger or simulate a specified event on one or more elements. It can be used to invoke event handlers or execute default behaviors associated with the event. The trigger()
method is especially useful when you need to trigger events in response to certain actions or dynamically trigger events based on specific conditions.
Here’s the basic syntax of the trigger()
method:
$(selector).trigger(eventName)
Parameters:
selector
: A selector string or jQuery object representing the element(s) on which the event will be triggered.eventName
: A string representing the name of the event to be triggered (e.g., “click”, “change”, “mouseover”, etc.).
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery trigger() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
$(document).ready(function() {
// Attach a click event handler to the button
$("#myButton").on("click", function() {
alert("Button clicked!");
});
// Trigger the click event on the button programmatically
$("#myButton").trigger("click");
});
</script>
</body>
</html>
In this example, we have a button with the ID “myButton”. We attach a click event handler to the button using the on()
method. We then use the trigger()
method to programmatically trigger the click event on the button. When the page loads, the click event is triggered immediately, and an alert with the message “Button clicked!” will be displayed.
The trigger()
method can also be used to pass additional data to event handlers using the optional second parameter:
$(selector).trigger(eventName, data)
Here, data
is an object containing the additional data you want to pass to the event handler.
// Example with passing data to the event handler
$("#myButton").on("customEvent", function(event, data) {
alert("Custom event triggered with data: " + data);
});
$("#myButton").trigger("customEvent", "Hello, this is custom data");
The trigger()
method is a powerful tool in jQuery that enables you to simulate events and control the flow of your application based on user interactions or other conditions.