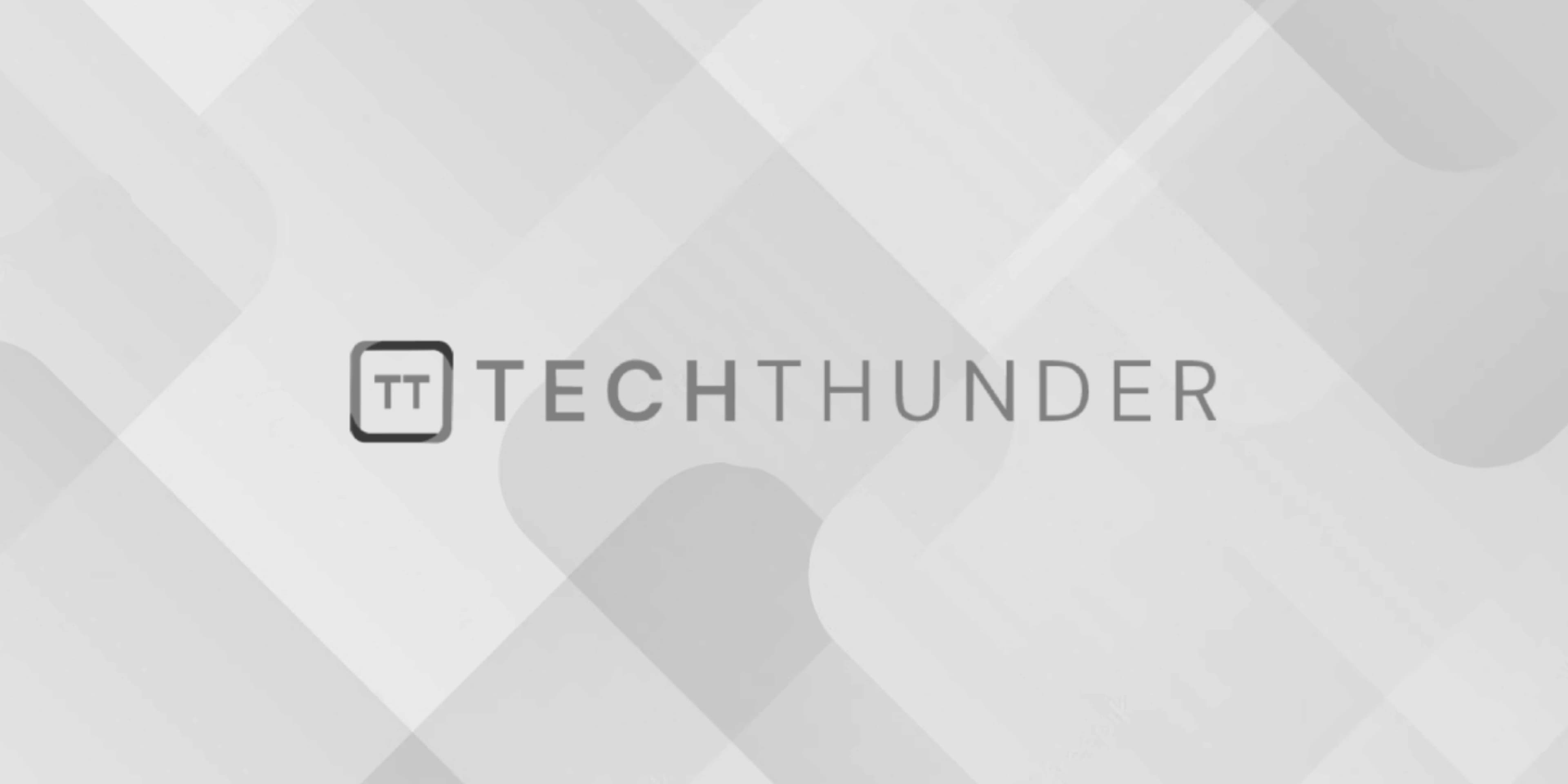
jQuery each() method
The each()
method in jQuery is used to iterate over a collection of elements or an array-like object and execute a function for each element in the collection. It provides a simple way to loop through elements and perform some action or operation on them.
The syntax for using the each()
method is as follows:
$(selector).each(function(index, element) {
// Function body
});
selector
: It is a string that specifies the elements to be selected.function(index, element)
: The callback function that will be executed for each element in the selected collection. It takes two arguments:index
: The index of the current element in the collection. It is a zero-based integer.element
: The DOM element at the current index.
Here’s an example of how you can use the each()
method:
HTML:
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
JavaScript:
// Loop through each <li> element and change the text content
$('li').each(function(index, element) {
$(element).text('New Item ' + (index + 1));
});
In the above example, the each()
method is used to iterate over all <li>
elements and change their text content to “New Item 1”, “New Item 2”, and “New Item 3” respectively.
The each()
method can be applied to any jQuery collection, including selected elements, arrays, and objects. When applied to an object, the callback function is called with two arguments: the key and the value of each property in the object.
// Loop through an object and print key-value pairs
var myObj = { a: 1, b: 2, c: 3 };
$.each(myObj, function(key, value) {
console.log(key + ': ' + value);
});
The each()
method is a convenient way to perform operations on a set of elements or items in an array-like object without the need for explicit looping constructs like for
or forEach
.