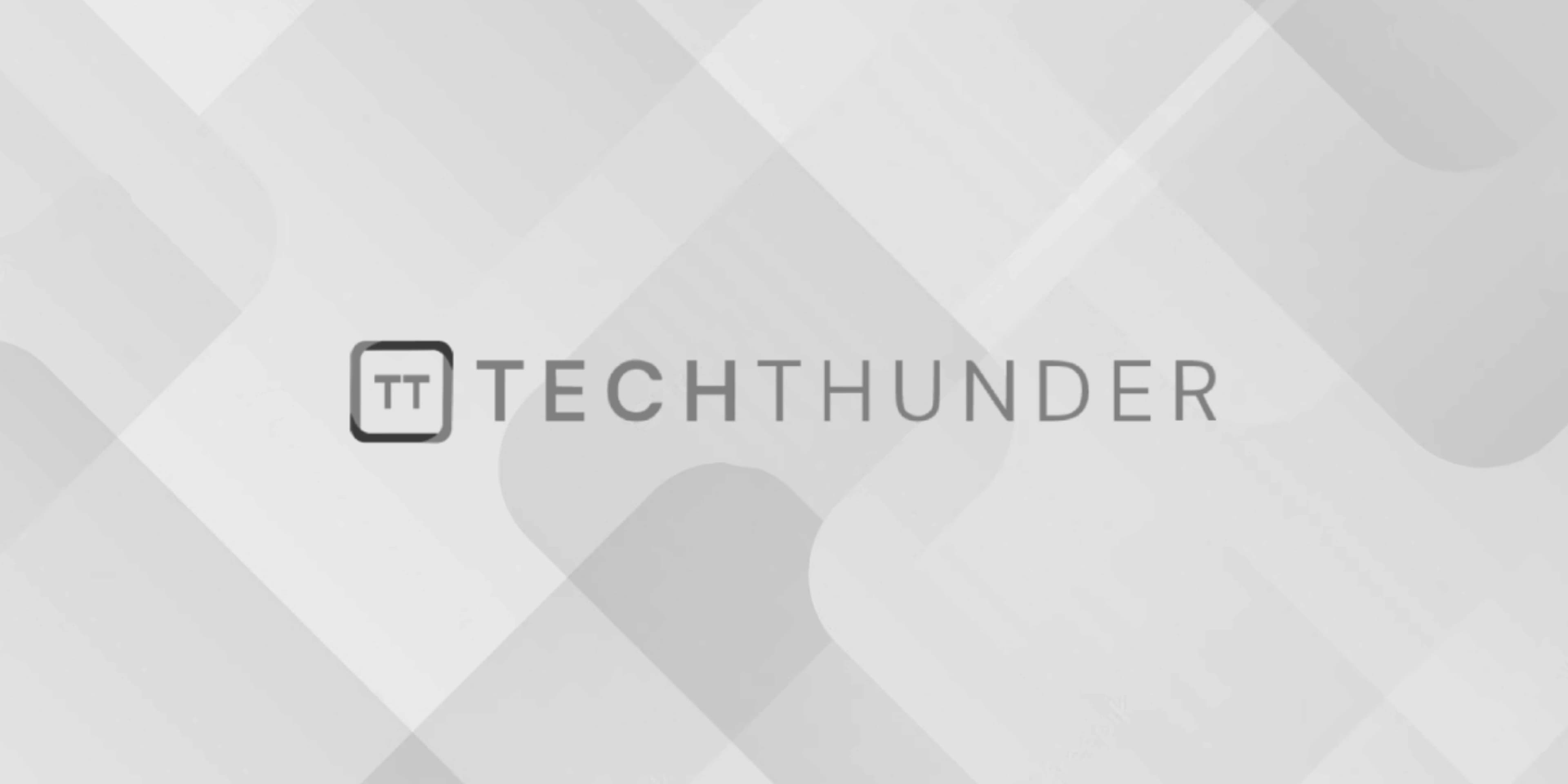
jQuery star rating
To create a star rating using jQuery, you can use HTML, CSS, and JavaScript/jQuery. Here’s a simple example of how you can implement a star rating system:
HTML:
<div class="rating">
<span class="star" data-rating="1"></span>
<span class="star" data-rating="2"></span>
<span class="star" data-rating="3"></span>
<span class="star" data-rating="4"></span>
<span class="star" data-rating="5"></span>
</div>
CSS:
.rating {
display: inline-block;
font-size: 0; /* remove white space between inline-block elements */
}
.star {
display: inline-block;
width: 30px;
height: 30px;
background: url('path_to_star_icon.png') 0 0; /* use your star icon */
background-size: 100%;
cursor: pointer;
}
.star.active {
background-position: 0 -30px; /* use your active star icon position */
}
JavaScript/jQuery:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
// Initial rating value
var currentRating = 0;
// Add click event to stars
$('.star').on('click', function() {
// Get the rating value from the data attribute
var rating = $(this).data('rating');
// Update the rating and display the active stars
updateRating(rating);
});
// Function to update the rating
function updateRating(rating) {
currentRating = rating;
$('.star').removeClass('active');
$('.star').slice(0, currentRating).addClass('active');
}
});
</script>
In this example, each star is represented by a <span>
element with a star
class, and the rating value is stored in the data-rating
attribute. The CSS styles the stars with an icon and handles the active state when a star is clicked. The JavaScript/jQuery code handles the click event and updates the rating accordingly.
Make sure to replace 'path_to_star_icon.png'
with the actual path to your star icon image. You can customize the star icon and its active state by modifying the CSS styles.
This is a basic implementation of a star rating system. Depending on your specific requirements, you can enhance the functionality by saving the rating value, submitting it to the server, or displaying additional information based on the rating.