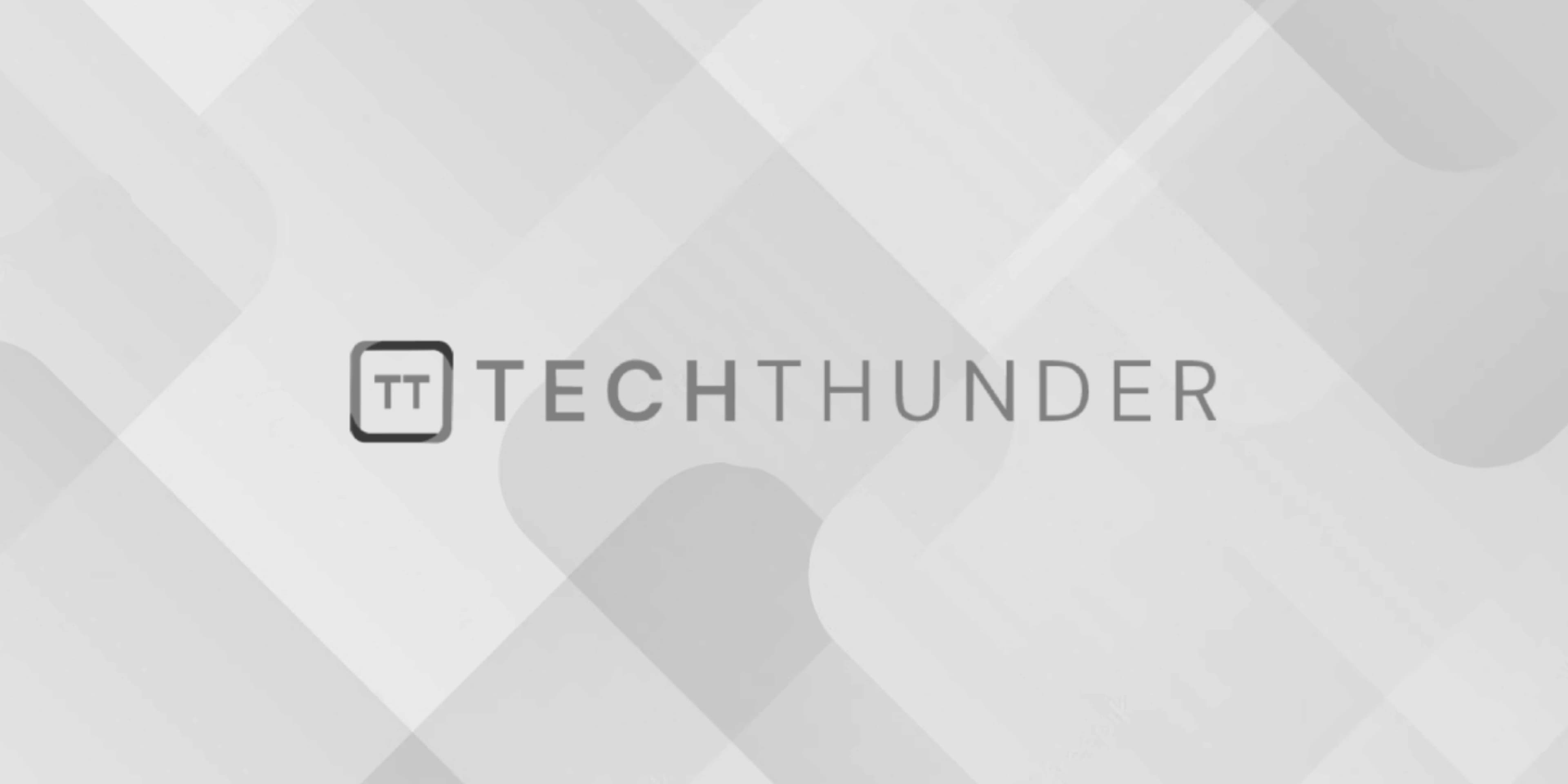
jQuery hover() method
The jQuery hover()
method is a shorthand for attaching mouseenter and mouseleave event handlers to an element. It allows you to specify two functions to be executed when the mouse pointer enters and leaves the area of a selected element. The first function is executed when the mouse pointer enters the element, and the second function is executed when the mouse pointer leaves the element.
The syntax for using the hover()
method is as follows:
$(selector).hover(function() {
// Code to execute when the mouse enters the element
}, function() {
// Code to execute when the mouse leaves the element
});
selector
: It is a string that specifies the elements to be selected.- The first function: The callback function to be executed when the mouse enters the element.
- The second function: The callback function to be executed when the mouse leaves the element.
Here’s an example of how you can use the hover()
method:
HTML:
<div id="myDiv" style="width: 100px; height: 100px; background-color: blue;"></div>
JavaScript:
// Attach hover event handlers to the div element
$('#myDiv').hover(
function() {
$(this).css('background-color', 'red'); // Change background color to red on mouseenter
},
function() {
$(this).css('background-color', 'blue'); // Change background color back to blue on mouseleave
}
);
In the above example, when you move the mouse pointer over the div with the ID “myDiv,” the first function will be executed (background color changes to red), and when you move the mouse pointer out of the div, the second function will be executed (background color changes back to blue).
The hover()
method is a convenient way to add hover effects to elements without having to separately attach mouseenter
and mouseleave
event handlers. However, keep in mind that as of jQuery 3.0, the hover()
method has been removed, and it’s recommended to use separate mouseenter
and mouseleave
event handlers or use the on()
method to handle these events. For example:
// Using separate mouseenter and mouseleave event handlers
$('#myDiv').on('mouseenter', function() {
$(this).css('background-color', 'red');
}).on('mouseleave', function() {
$(this).css('background-color', 'blue');
});