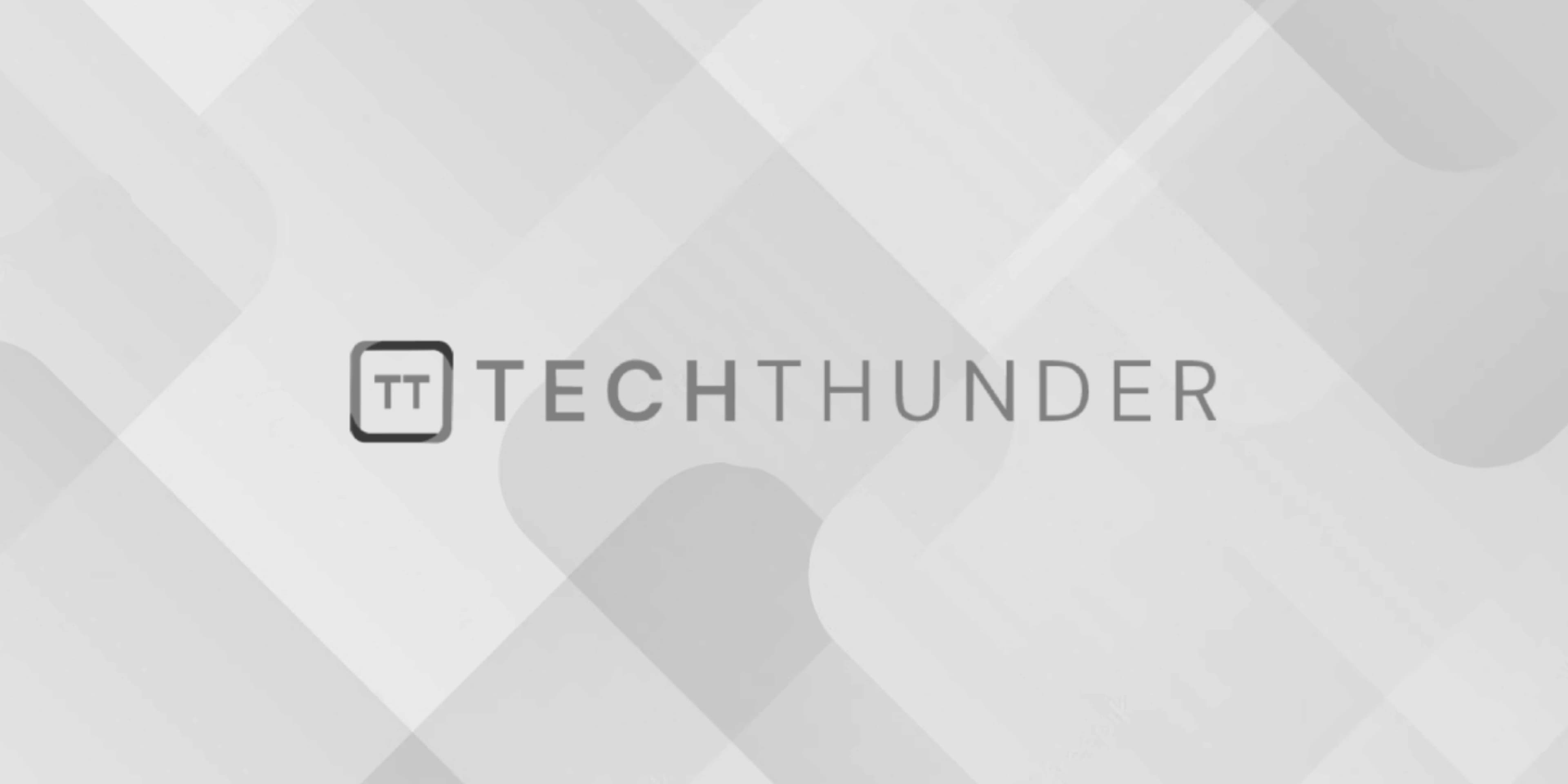
jQuery is() method
The jQuery is()
method is used to check whether a set of matched elements matches a given selector, element, or jQuery object. It returns true
if at least one of the selected elements matches the specified criteria, otherwise, it returns false
.
Here’s the basic syntax of the is()
method:
$(selector).is(filterExpression)
Parameters:
selector
: A selector string representing the elements to be checked.filterExpression
: A selector string, element, or another jQuery object representing the elements that should be checked for matching.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery is() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="box">This is a box</div>
<div class="circle">This is a circle</div>
<script>
$(document).ready(function() {
// Check if the first div has the class "box"
var isFirstDivBox = $('div').first().is('.box');
console.log('Is first div a box?', isFirstDivBox);
// Check if the second div is a circle
var isSecondDivCircle = $('div').eq(1).is('.circle');
console.log('Is second div a circle?', isSecondDivCircle);
});
</script>
</body>
</html>
In this example, we have two <div>
elements with different classes (“box” and “circle”). We use the is()
method to check if the first div has the class “box” and if the second div has the class “circle”. The method returns true
or false
accordingly, and we log the results to the console.
The is()
method is commonly used in conditional statements or when you need to test if a specific element matches certain criteria before performing some actions. It is a helpful method for checking the characteristics of selected elements and making decisions based on their attributes or classes.