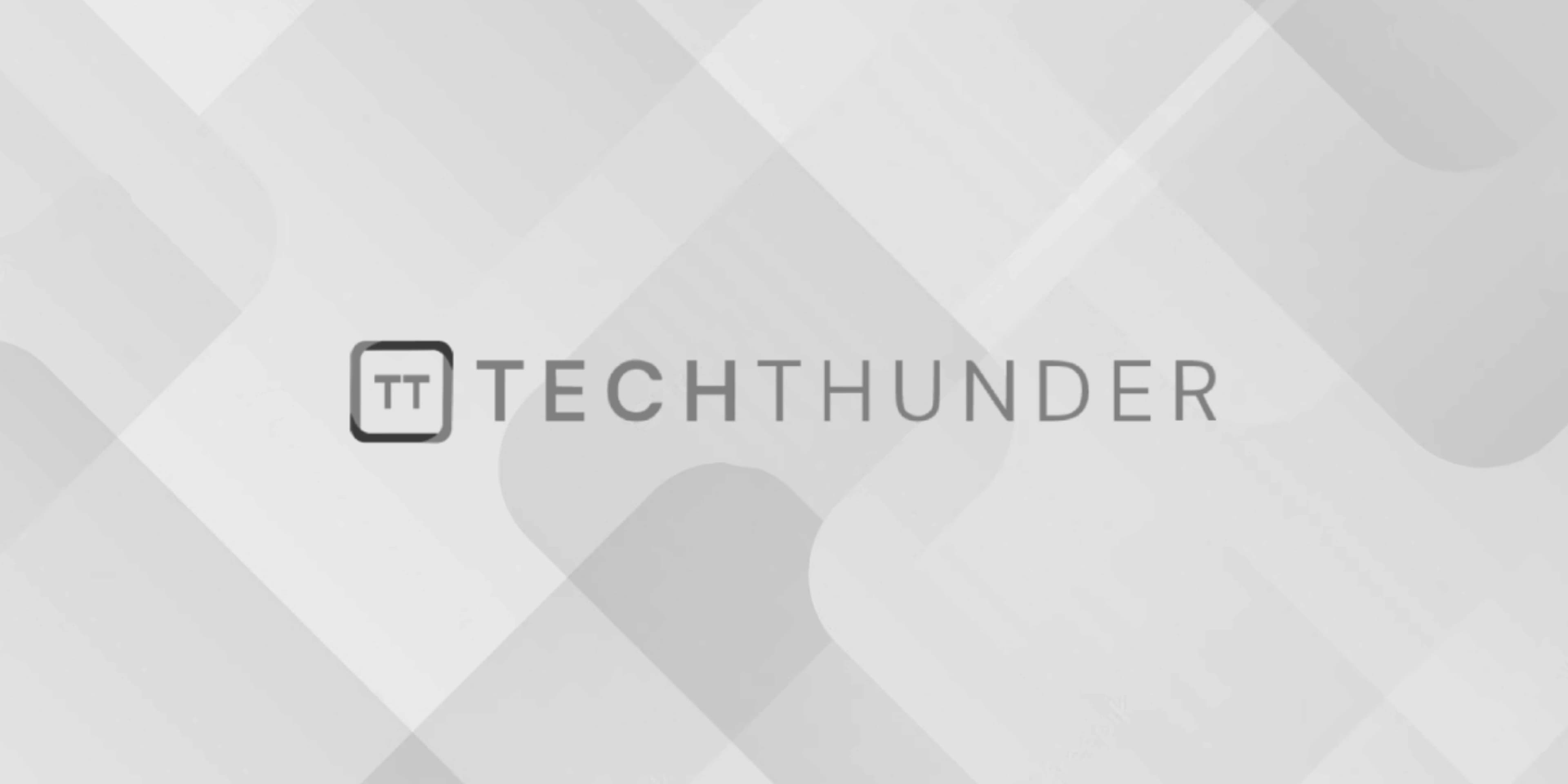
jQuery find() method
The jQuery find()
method is used to search for descendant elements within a matched set of elements. It allows you to locate elements that are nested inside other elements and match a specific selector.
Here’s the basic syntax of the find()
method:
$(selector).find(filterExpression)
Parameters:
selector
: A selector string representing the elements to be found within the matched set.filterExpression
: (Optional) A selector string, element, or another jQuery object representing the elements to be searched for within the matched set.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery find() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="container">
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
</div>
<script>
$(document).ready(function() {
// Find all list items within the div with class "container"
var listItems = $('.container').find('li');
listItems.css('color', 'blue');
});
</script>
</body>
</html>
In this example, we have a <div>
with a class of “container” that contains an unordered list (<ul>
) with three list items (<li>
). We use the find()
method to select all the list items that are descendants of the element with the class “container”. We then apply a blue text color to those list items using the css()
method.
The find()
method is particularly useful when you want to perform operations on specific elements within a nested structure. It allows you to search for elements within a parent element and manipulate them without affecting other elements on the page.