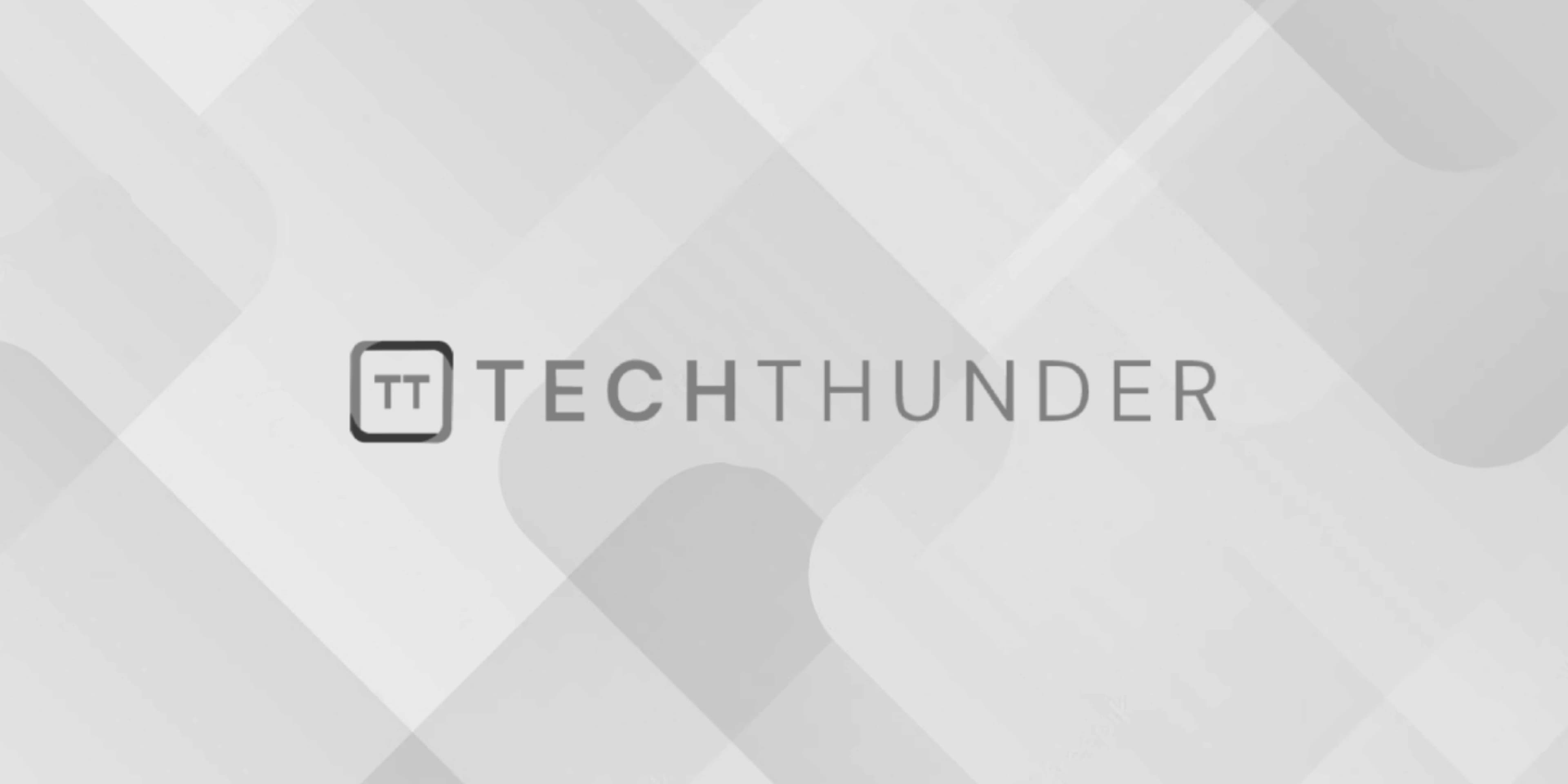
jQuery has() method
The jQuery.has()
method in jQuery, also known as the .has()
selector. This method is used to filter elements based on whether they contain any descendants that match the specified selector.
Here’s the basic syntax of the has()
method:
$(selector).has(descendantSelector)
Parameters:
selector
: A selector expression used to select elements from which you want to filter out those that contain descendants matching thedescendantSelector
.descendantSelector
: A selector expression used to select the descendants you want to check for existence within the elements matched by theselector
.
Return Value:
The has()
method returns a new jQuery object containing only the elements from the original selection that have descendants matching the descendantSelector
.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery has() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div>
<p>Paragraph 1</p>
</div>
<div>
<span>Span 1</span>
</div>
<div>
<p>Paragraph 2</p>
<span>Span 2</span>
</div>
<script>
$(document).ready(function() {
// Select div elements that contain a <p> element
var divsWithParagraph = $("div").has("p");
// Log the selected div elements
console.log(divsWithParagraph);
});
</script>
</body>
</html>
In this example, we use the has()
method to select the <div>
elements that contain a <p>
element. The method returns a jQuery object containing the first and third <div>
elements since they are the ones that have <p>
elements as descendants.
The output of the above example will be:
[<div>...</div>, <div>...</div>]
In this output, we see that the has()
method successfully selected the <div>
elements that contain <p>
elements.
The has()
method is useful when you want to filter elements based on the existence of certain descendants within them. It allows you to easily find parent elements that have specific child elements matching a particular selector.