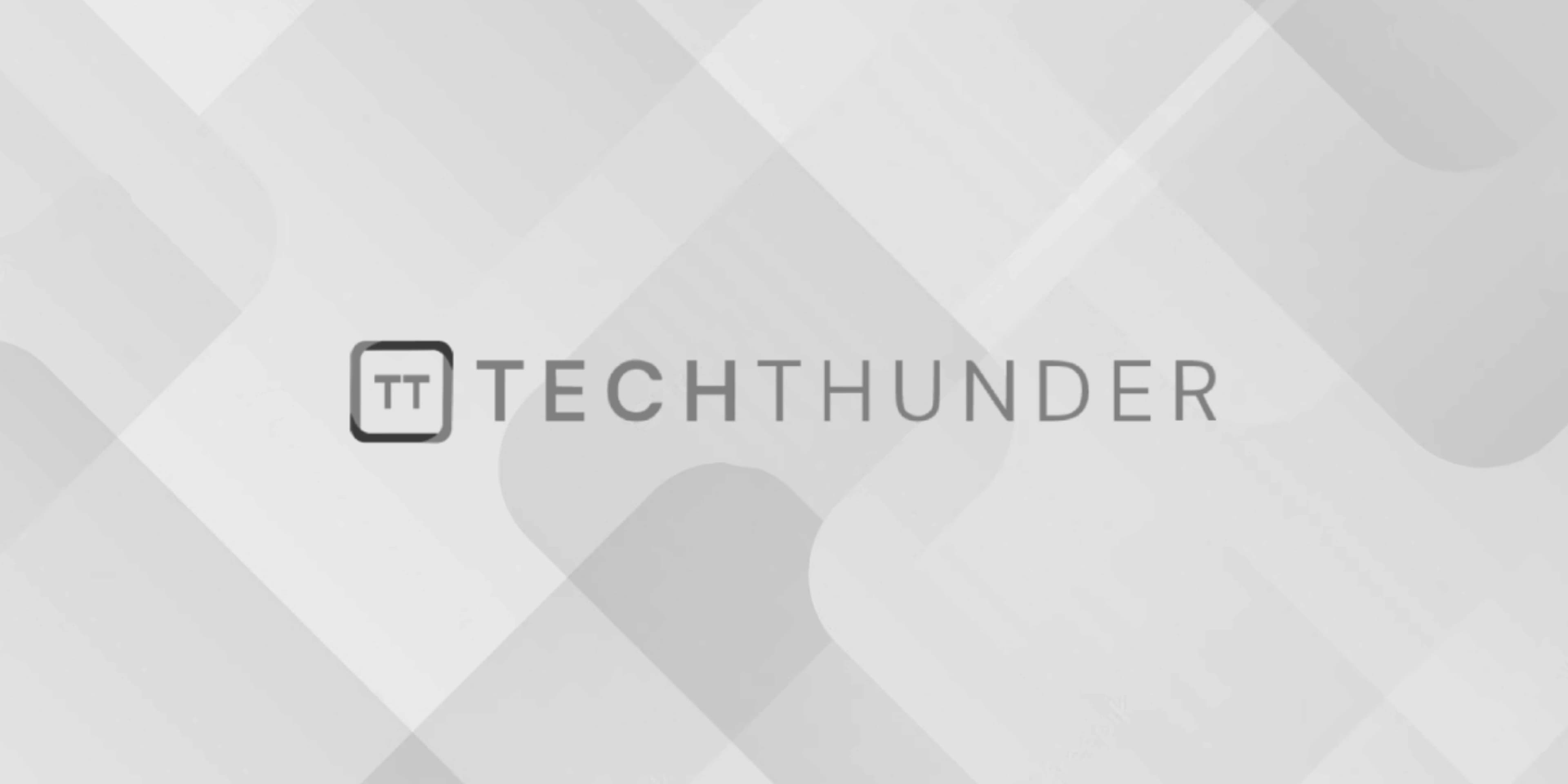
jQuery isWindow() method
As of my last update in September 2021, the jQuery.isWindow()
method is a utility method in jQuery used to check if an object is a window object.
Here’s the basic syntax of the jQuery.isWindow()
method:
jQuery.isWindow(obj)
Parameters:
obj
: The object to be tested.
Return Value:
The jQuery.isWindow()
method returns true
if the given object is a window object, otherwise it returns false
.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery isWindow() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="myDiv">This is a div element</div>
<script>
$(document).ready(function() {
var divElement = document.getElementById("myDiv");
var isDivWindow = jQuery.isWindow(divElement);
console.log(isDivWindow); // Output: false
var windowObj = window;
var isWindow = jQuery.isWindow(windowObj);
console.log(isWindow); // Output: true
});
</script>
</body>
</html>
In this example, we test two objects using the jQuery.isWindow()
method. The first object divElement
is a DOM element, and it returns false
because it is not a window object. The second object windowObj
is the window
object itself, and it returns true
because it is a window object.
Please note that jQuery.isWindow()
is particularly useful when you have a variable that may refer to either a window object or something else, and you want to differentiate between them. The method internally checks if the object has properties typical of a window object, such as window
, document
, and others.
As a side note, since version 3.0 of jQuery, this method has been removed from the core library and is only available in the jQuery Migrate plugin for backward compatibility. If you are using a newer version of jQuery, consider using the native instanceof
operator to check if an object is a window object:
var isWindow = (obj instanceof Window);
This will return true
if the obj
is a window object and false
otherwise.