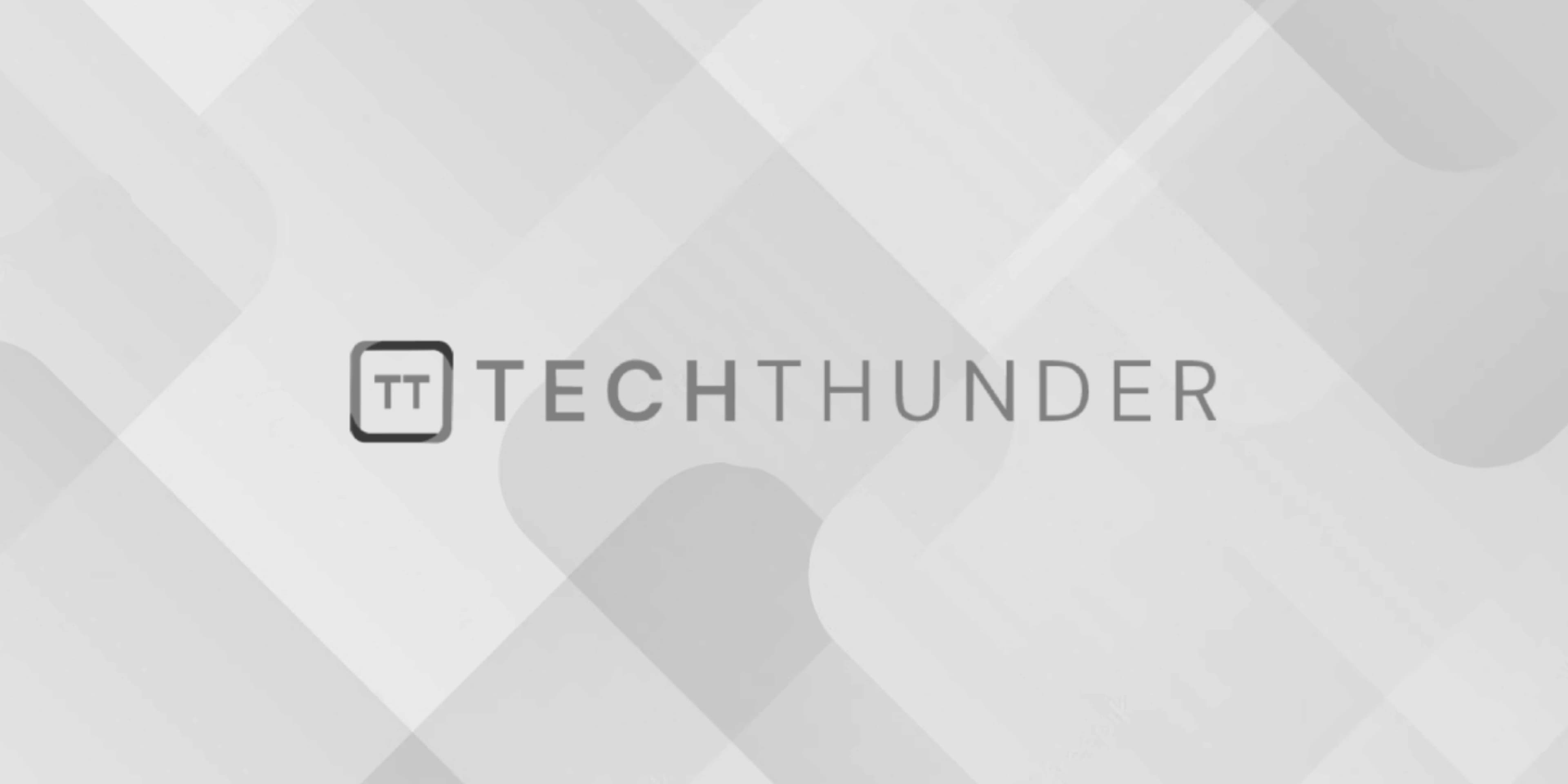
jQuery extend() method
The jQuery.extend()
method in jQuery is used to merge the contents of two or more objects together into the first object. It is primarily used for extending the properties of one object with the properties from another object, allowing you to create a new, combined object.
The syntax for using the jQuery.extend()
method is as follows:
jQuery.extend(target, object1, object2, ...);
target
: The object that will receive the merged properties. It can be an empty object or an existing object to which properties will be added or overwritten.object1
,object2
, …: The objects containing properties to be merged into thetarget
object. You can pass multiple objects separated by commas.
Here’s an example of how you can use the jQuery.extend()
method:
// Create two objects to be merged
var obj1 = { a: 1, b: 2 };
var obj2 = { b: 3, c: 4 };
// Merge obj2 and obj1 into a new empty object
var result1 = jQuery.extend({}, obj1, obj2);
console.log(result1);
// Output: { a: 1, b: 3, c: 4 }
// Merge obj2 and obj1 into obj1 (modifying obj1)
var result2 = jQuery.extend(obj1, obj2);
console.log(result2);
// Output: { a: 1, b: 3, c: 4 } (obj1 is also modified)
// Note that obj1 is modified in the above example. If you want to preserve obj1 and create a new object, use an empty object as the target:
var newObj = jQuery.extend({}, obj1, obj2);
console.log(newObj);
// Output: { a: 1, b: 3, c: 4 } (obj1 is not modified)
The jQuery.extend()
method can be particularly useful when you want to combine the properties of multiple objects into one, such as merging default options with user-provided options, or combining configuration settings for a plugin.
Keep in mind that jQuery.extend()
performs a shallow copy, meaning that it only merges the top-level properties of objects. If the objects contain nested objects or arrays, those will not be deeply merged. For deep merging, you may need to use a specialized deep-merge function or a library that supports deep merging.