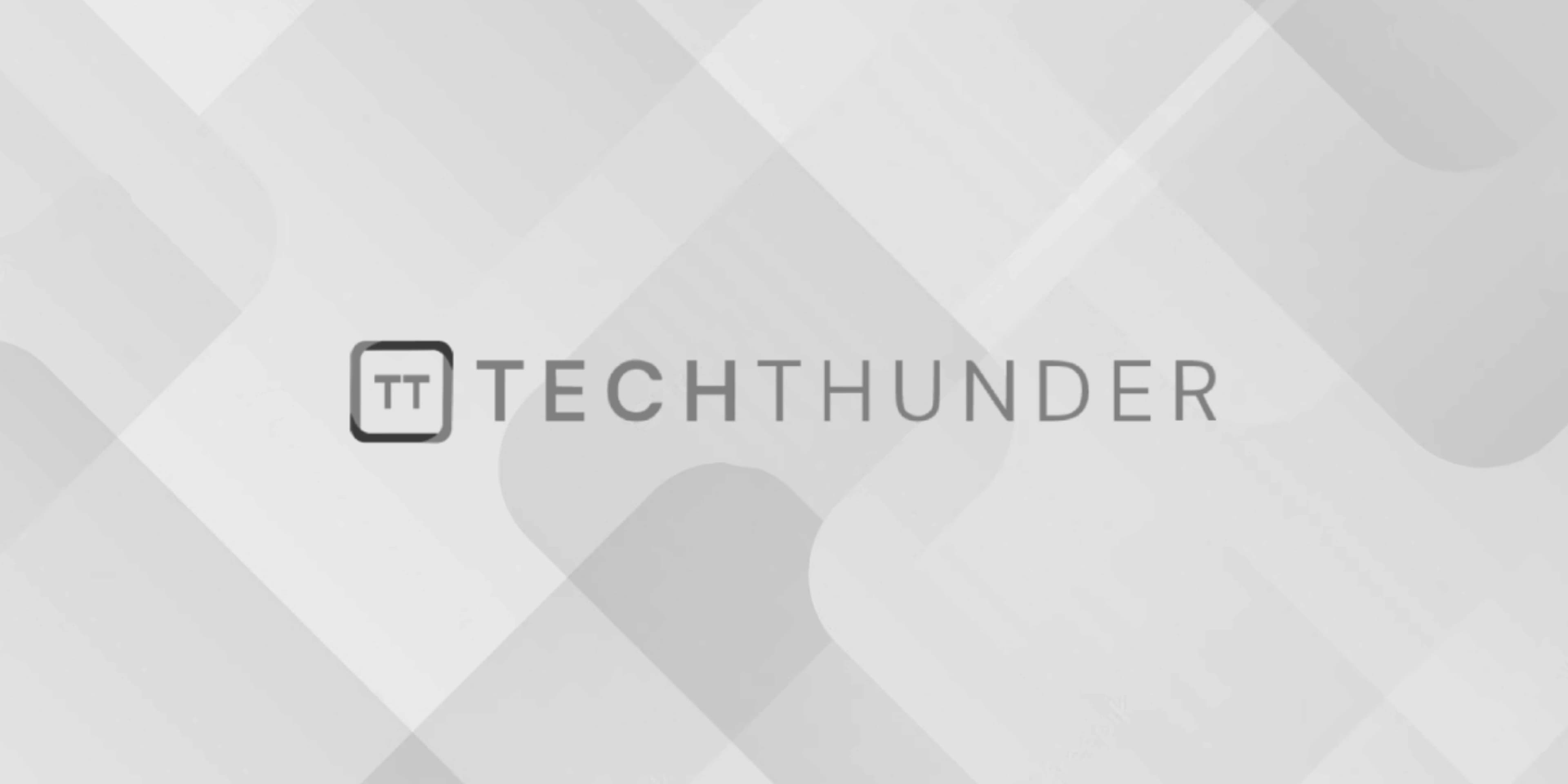
163 views
offset vs position in jQuery
In jQuery, offset()
and position()
are two methods used to retrieve the position of an element relative to its parent or to the document.
offset()
method:
Theoffset()
method returns an object that contains the propertiestop
andleft
, representing the distance of the element’s top-left corner from the top-left corner of the document. It takes into account the element’s margin, padding, border, and scroll position.
Example:
<div id="myElement" style="position: relative; top: 100px; left: 50px;"></div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
var elementOffset = $('#myElement').offset();
console.log('Top offset:', elementOffset.top);
console.log('Left offset:', elementOffset.left);
});
</script>
In this example, the offset()
method is used to retrieve the top and left offset of the myElement
div relative to the document.
position()
method:
Theposition()
method, on the other hand, returns an object withtop
andleft
properties representing the position of the element relative to its offset parent. An offset parent is the closest ancestor element with a CSS property ofposition
other thanstatic
(i.e.,relative
,absolute
,fixed
, orsticky
).
Example:
<div style="position: relative;">
<div id="myElement" style="position: absolute; top: 100px; left: 50px;"></div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
var elementPosition = $('#myElement').position();
console.log('Top position:', elementPosition.top);
console.log('Left position:', elementPosition.left);
});
</script>
In this example, the position()
method is used to retrieve the top and left position of the myElement
div relative to its offset parent, which is the outer div
with position: relative
.
In summary:
offset()
gives the position of the element relative to the document, considering margins, padding, borders, and scroll position.position()
gives the position of the element relative to its offset parent, which is the closest ancestor element with a CSS position other thanstatic
.
Use offset()
when you want to get the absolute position of an element on the entire document, and use position()
when you want to get the position of an element relative to its immediate offset parent.