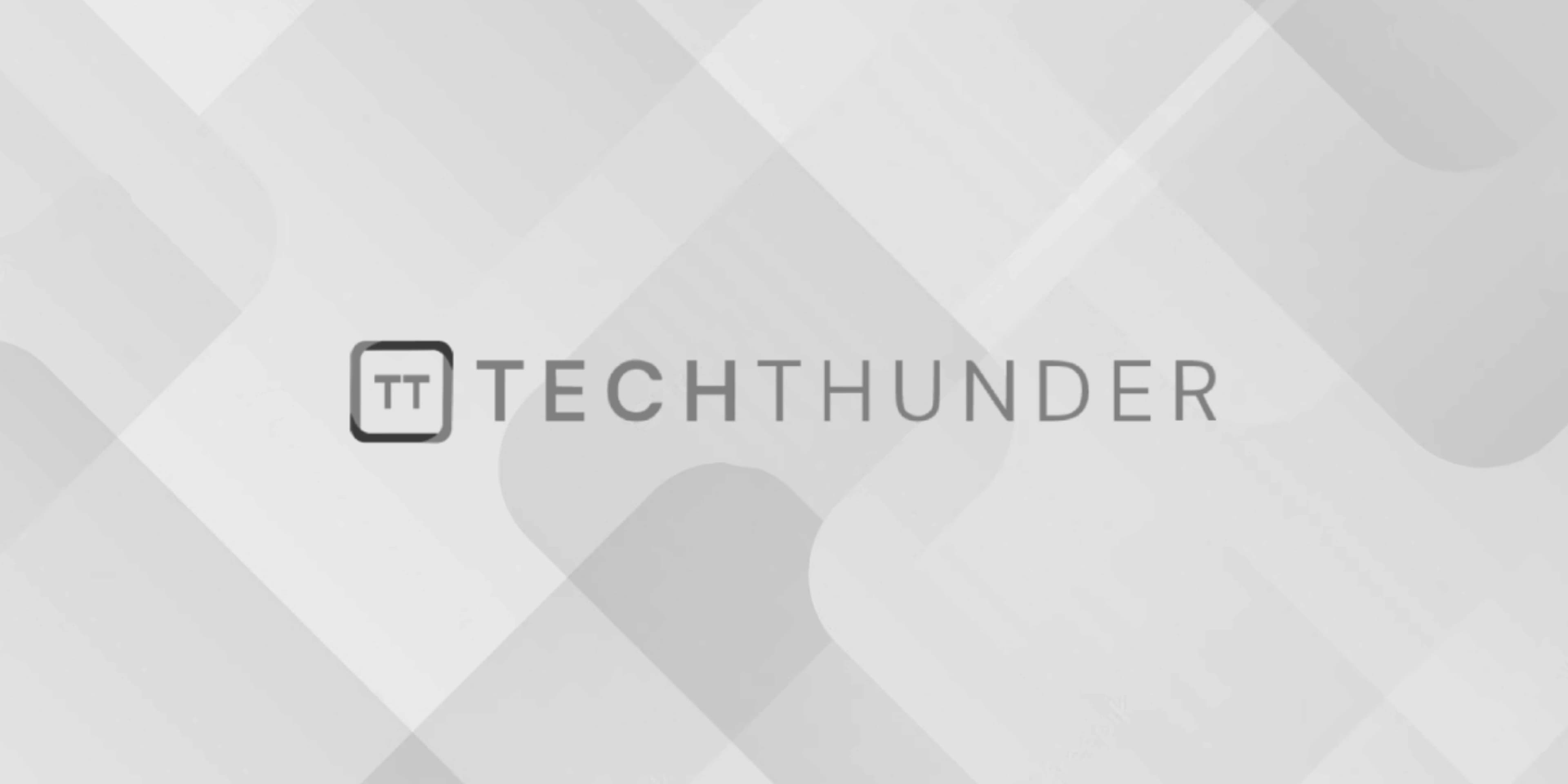
Add remove input fields dynamically using jQuery with Bootstrap
To dynamically add and remove input fields using jQuery and Bootstrap, you can combine the power of jQuery for dynamic manipulation and Bootstrap for styling and layout. Here’s a step-by-step guide:
Step 1: Set up your HTML structure with Bootstrap classes.
Step 2: Implement the “Add” functionality to add new input fields.
Step 3: Implement the “Remove” functionality to remove input fields.
Here’s an example of how to achieve this:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Add/Remove Input Fields with Bootstrap</title>
<!-- Link to Bootstrap CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.3.0/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h2>Add or Remove Input Fields</h2>
<div id="inputContainer">
<!-- Input fields will be dynamically added here -->
</div>
<button type="button" class="btn btn-primary mt-2" id="addButton">Add Field</button>
</div>
<!-- Link to jQuery -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
var maxFields = 5; // Set the maximum number of input fields
var addButton = $('#addButton');
var inputContainer = $('#inputContainer');
var fieldIndex = 1;
// Add Functionality
addButton.click(function() {
if (fieldIndex < maxFields) {
fieldIndex++;
var newField = '<div class="input-group mb-2"><input type="text" class="form-control" name="field' + fieldIndex + '">\
<button type="button" class="btn btn-danger removeButton">Remove</button></div>';
inputContainer.append(newField);
}
});
// Remove Functionality
$(document).on('click', '.removeButton', function() {
$(this).parent().remove();
fieldIndex--;
});
});
In this example, the JavaScript code uses jQuery for the dynamic addition and removal of input fields. Bootstrap classes are used for styling the input fields and the “Add” button.
The “Add” button has the class btn btn-primary
, which gives it the Bootstrap primary button styling. The input fields are wrapped in a div
with the class input-group mb-2
, which applies the Bootstrap input group style and adds some margin between each input field.
The “Remove” buttons have the class btn btn-danger
, which gives them the Bootstrap danger button styling.
With this implementation, you can dynamically add and remove input fields using jQuery and Bootstrap, giving you a clean and user-friendly interface for managing input fields. You can further customize the form and styling based on your specific requirements.