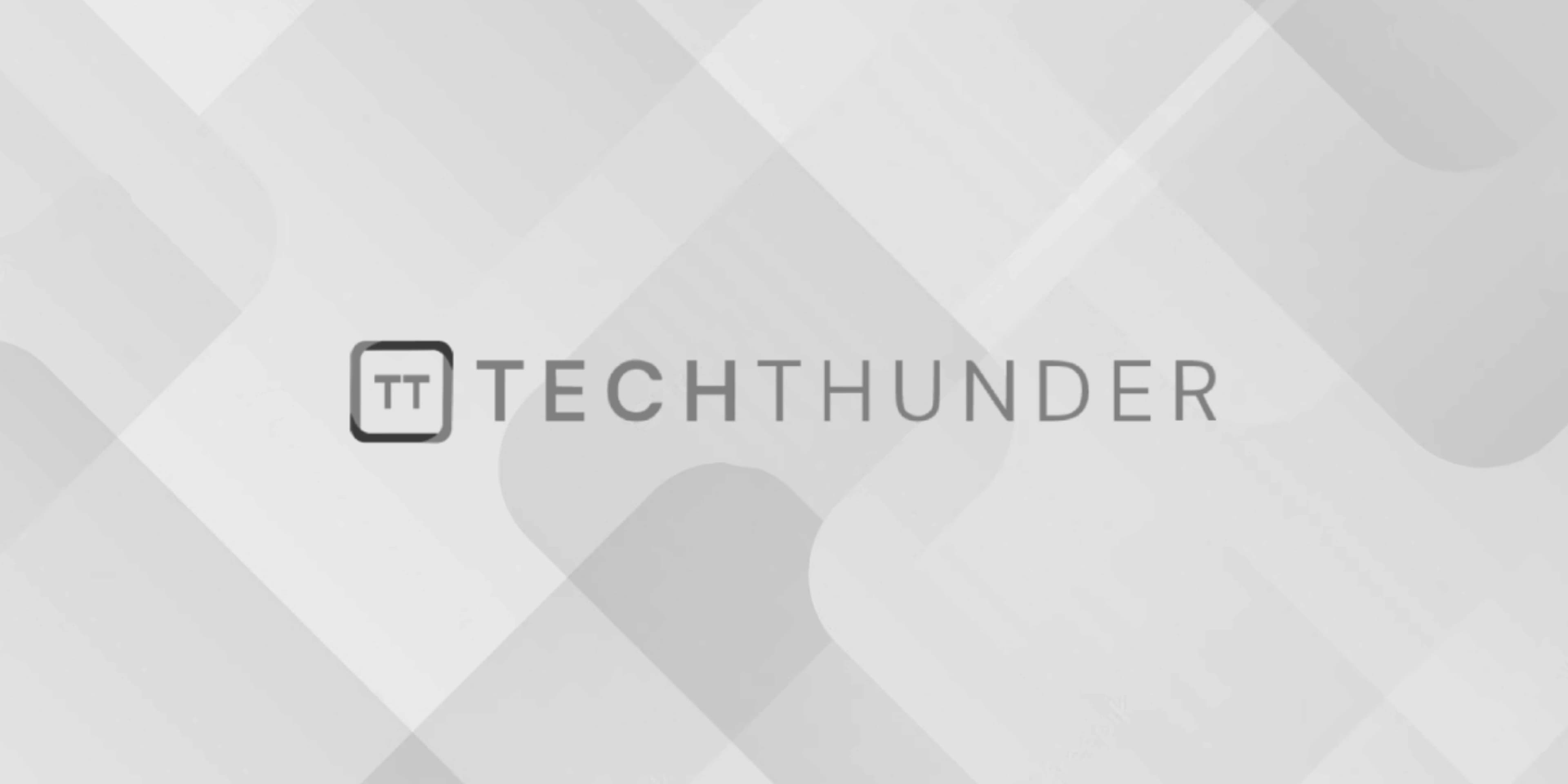
Integrate jQuery Fullcalendar using Bootstrap, PHP and MySQL
To integrate jQuery FullCalendar with Bootstrap, PHP, and MySQL, you can follow these steps:
- Set up the database and table to store events.
- Create the server-side PHP script to fetch events from the database.
- Create the HTML page with FullCalendar and integrate it with the PHP script.
- Use Bootstrap to style the page.
Let’s go through each step in detail:
Step 1: Set up the database and table to store events
Create a database and a table to store events. The table should have columns for the event ID, title, start date, end date, and any other relevant information.
Step 2: Create the server-side PHP script to fetch events from the database
Create a PHP script to retrieve events from the database and return them in JSON format. Here’s an example PHP script named events.php
:
<?php
// Replace with your database credentials
$host = 'localhost';
$username = 'your_username';
$password = 'your_password';
$database = 'your_database';
// Connect to the database
$connection = new mysqli($host, $username, $password, $database);
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
// Fetch events from the database
$query = "SELECT id, title, start, end FROM events";
$result = $connection->query($query);
// Convert events to JSON format
$events = array();
while ($row = $result->fetch_assoc()) {
$events[] = $row;
}
// Close the database connection
$connection->close();
// Return events in JSON format
header('Content-Type: application/json');
echo json_encode($events);
?>
Step 3: Create the HTML page with FullCalendar and integrate it with the PHP script
Create an HTML page and include the necessary CSS and JS files for FullCalendar, Bootstrap, and jQuery. Then, initialize FullCalendar with the PHP script to fetch events dynamically. Here’s an example HTML page:
<!DOCTYPE html>
<html>
<head>
<title>jQuery FullCalendar with Bootstrap, PHP, and MySQL</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/fullcalendar/3.10.2/fullcalendar.min.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/fullcalendar/3.10.2/fullcalendar.min.js"></script>
</head>
<body>
<div class="container mt-5">
<div id="calendar"></div>
</div>
<script>
$(document).ready(function() {
$('#calendar').fullCalendar({
events: 'events.php', // Path to the PHP script to fetch events
});
});
</script>
</body>
</html>
Step 4: Use Bootstrap to style the page
You can use Bootstrap classes to style the FullCalendar and other elements on the page as needed.
Tthe FullCalendar will be displayed on the page, and events will be fetched from the database using the PHP script. The events
option in the FullCalendar initialization specifies the path to the PHP script that returns the events in JSON format.
Remember to create the events.php
script in the same directory as your HTML file to handle event retrieval from the database.
With these steps, you can integrate jQuery FullCalendar with Bootstrap, PHP, and MySQL to create a dynamic calendar application that allows you to manage events stored in the database.