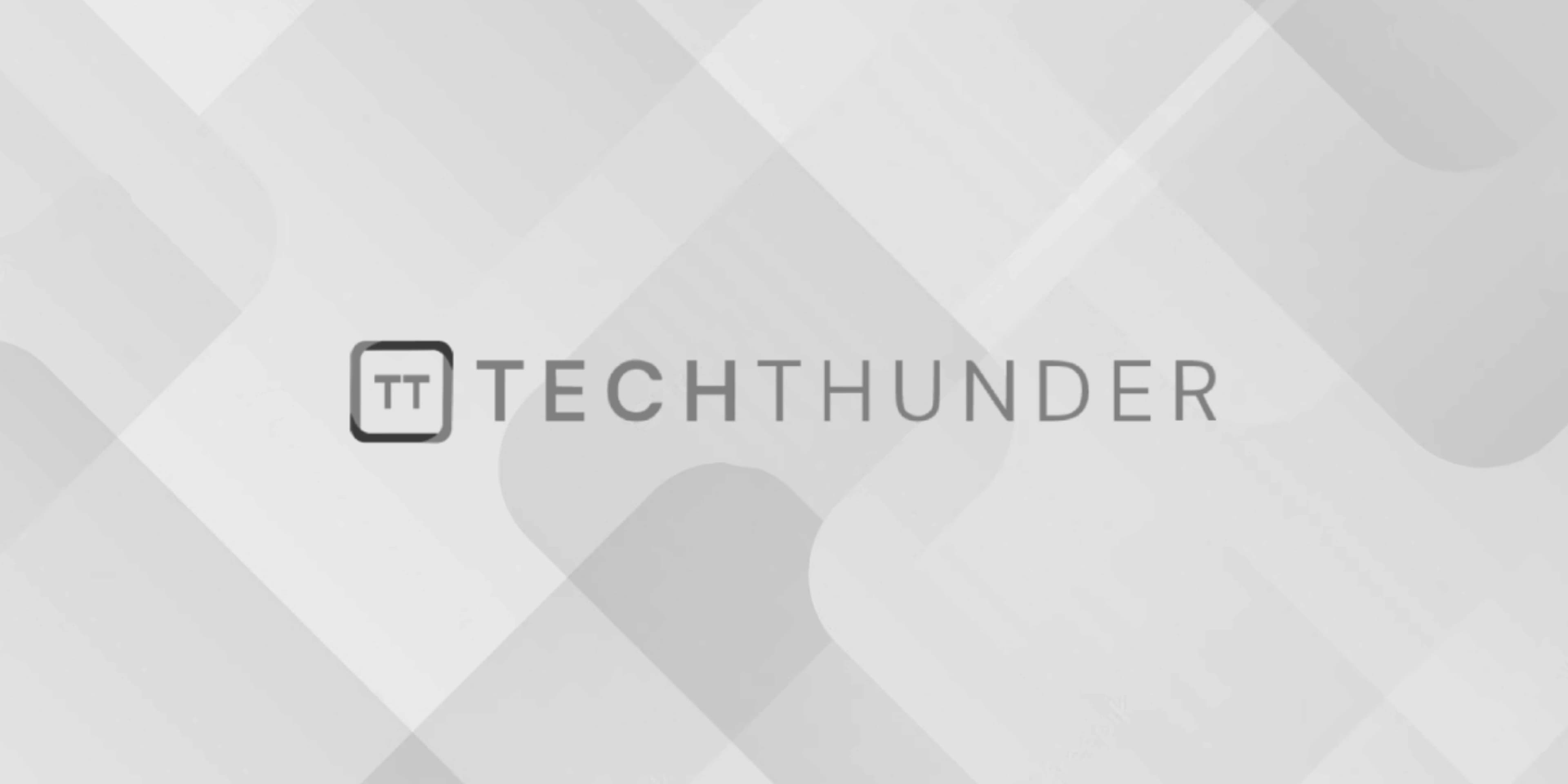
Read more functionality using jQuery
To implement a “Read More” functionality using jQuery, you can show a truncated version of the content with an ellipsis, and then reveal the full content when the “Read More” link is clicked. Here’s a step-by-step guide:
Step 1: Set up your HTML structure.
<!DOCTYPE html>
<html>
<head>
<title>Read More Functionality using jQuery</title>
<style>
.content {
overflow: hidden;
height: 100px; /* Set the initial height for truncated content */
}
.read-more-link {
cursor: pointer;
color: blue;
}
</style>
</head>
<body>
<div class="content">
<!-- Your content goes here -->
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed posuere urna a tellus iaculis, ut interdum mi congue.
Nunc vel ante non ex sagittis facilisis at quis neque. In hac habitasse platea dictumst.
</p>
</div>
<p class="read-more-link">Read More</p>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: JavaScript (script.js)
$(document).ready(function() {
// Read More functionality
$(".read-more-link").click(function() {
var content = $(this).prev(".content");
if (content.hasClass("expanded")) {
// If content is already expanded, truncate it again
content.removeClass("expanded");
content.animate({ height: "100px" }, 300); // Set the initial height
$(this).text("Read More");
} else {
// If content is truncated, expand it
content.addClass("expanded");
var autoHeight = content.css("height", "auto").height();
content.height("100px"); // Set the initial height
content.animate({ height: autoHeight }, 300);
$(this).text("Read Less");
}
});
});
In this example, the content is initially truncated to a fixed height of 100 pixels. When the “Read More” link is clicked, the content expands to show the full content, and the link text changes to “Read Less.” Clicking the link again collapses the content back to the truncated state with the “Read More” link text.
The CSS hides any overflowing content in the .content
container using overflow: hidden
. The JavaScript code uses jQuery to toggle the expansion of the content by adjusting the height property of the .content
element.
With this implementation, you have a basic “Read More” functionality using jQuery. You can customize the styles and animation duration to match your design preferences. Additionally, you can modify the JavaScript code to handle multiple “Read More” sections on the same page or use data attributes to store the full content to avoid content duplication.