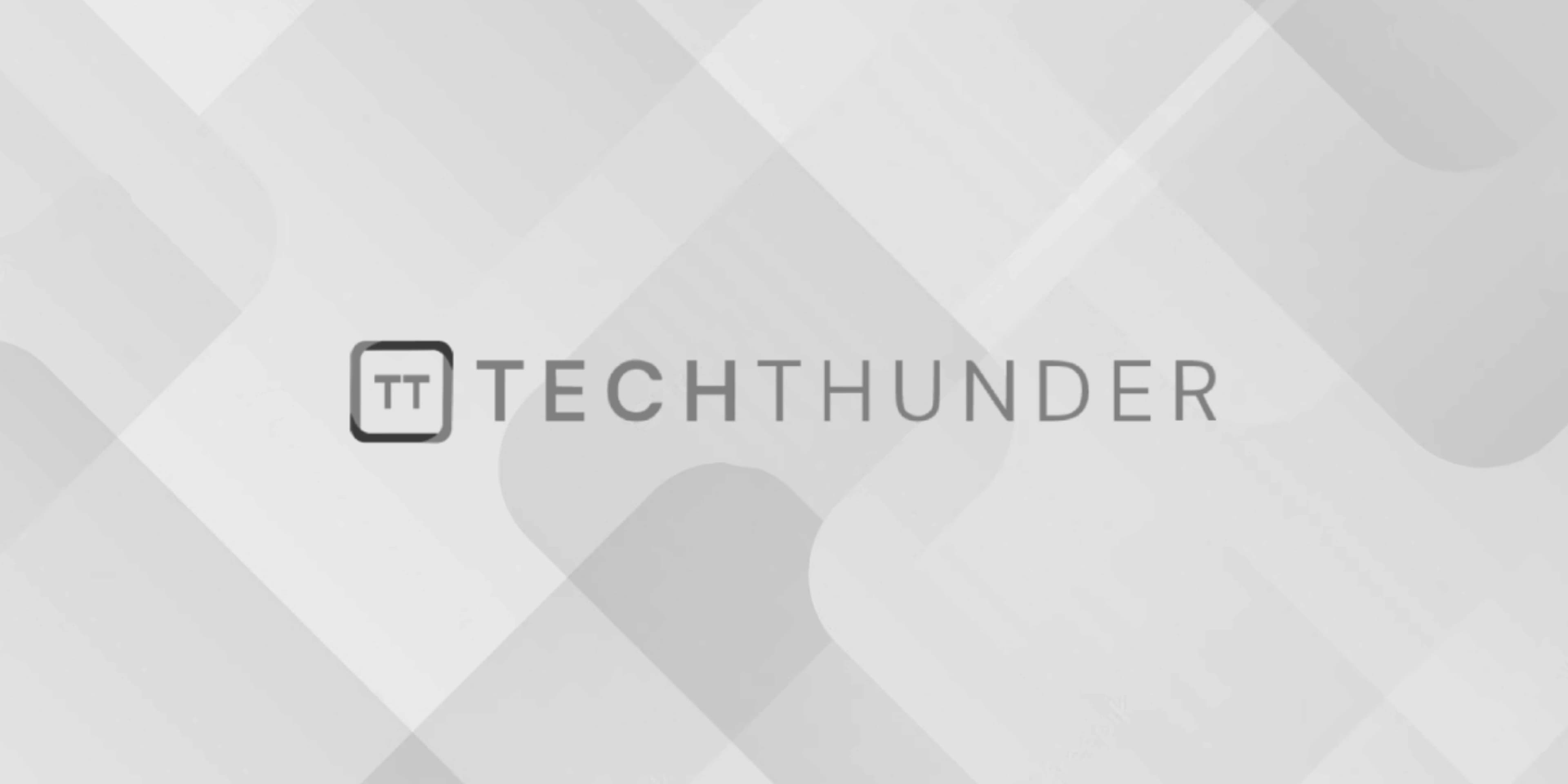
jQuery submit() method
The submit()
method in jQuery is used to trigger the submission of a form or to attach a function that will be executed when a form is submitted. It allows you to handle form submissions and perform actions or validations before the form is sent to the server.
The syntax for using the submit()
method is as follows:
$(selector).submit(function(event) {
// Function body
});
selector
: It is a string that specifies the form element to be selected.function(event)
: The callback function that will be executed when the form is submitted. It takes one argument:event
: The event object containing information about the form submission event. It provides details about the event, such as the form element, form data, and other related information.
Here’s an example of how you can use the submit()
method:
HTML:
<form id="myForm" action="submit.php" method="post">
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<button type="submit">Submit</button>
</form>
JavaScript:
// Attach a submit event handler to the form element
$('#myForm').submit(function(event) {
// Prevent the default form submission
event.preventDefault();
// Perform form validation
var username = $(this).find('input[name="username"]').val();
var password = $(this).find('input[name="password"]').val();
if (username === '' || password === '') {
alert('Please fill in all fields.');
return;
}
// If the form is valid, proceed with form submission
$(this).submit();
});
In the above example, when the user clicks the “Submit” button, the submit()
event handler will be triggered. It first prevents the default form submission using event.preventDefault()
, then performs form validation by checking if the username and password fields are filled in. If the form is valid, it proceeds with the form submission using $(this).submit()
. Otherwise, it displays an alert indicating that all fields must be filled.
The submit()
method is commonly used to handle form submissions, perform client-side validations, and manipulate form data before sending it to the server. It is essential to perform necessary client-side validations, but always remember to perform server-side validations as well for security and data integrity.