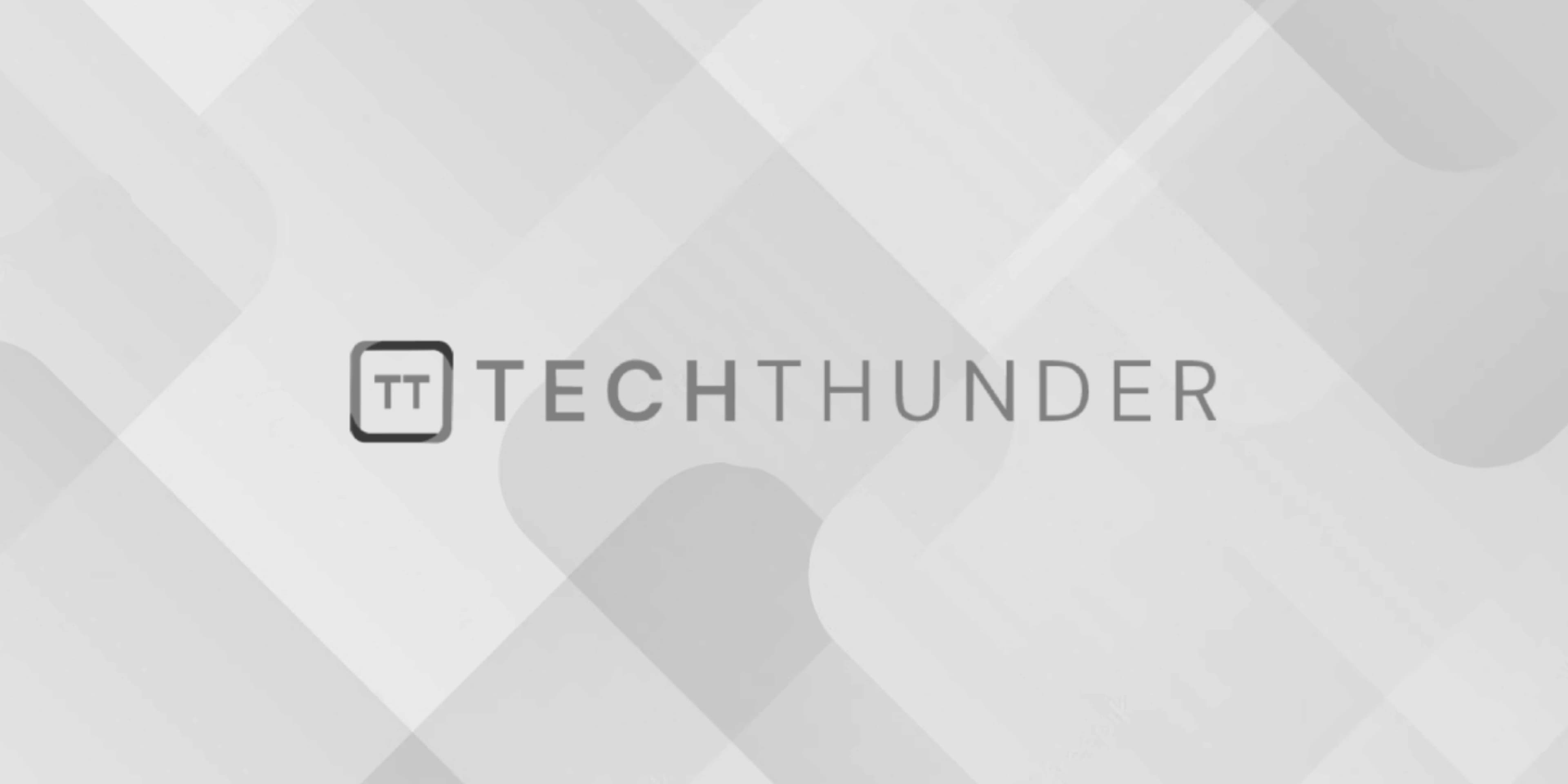
jQuery parentsUntil() method
The jQuery.parentsUntil()
method is used to select all ancestor elements between two specified elements in a DOM hierarchy. It allows you to select a range of ancestors that lie between a starting ancestor (not including the starting ancestor itself) and an ending ancestor (including the ending ancestor).
Here’s the basic syntax of the parentsUntil()
method:
$(selector).parentsUntil(stopSelector, filter)
Parameters:
selector
: The selector expression for the starting ancestor element. Elements that match this selector will not be included in the result.stopSelector
: The selector expression for the ending ancestor element. Elements that match this selector will be included in the result.filter
: Optional. A selector expression used to further filter the selected elements.
Return Value:
The parentsUntil()
method returns a jQuery object containing the selected ancestor elements that lie between the starting and ending ancestors.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery parentsUntil() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div class="grandparent">
<div class="parent">
<div class="child">
Some content here.
</div>
</div>
</div>
<script>
$(document).ready(function() {
// Select all ancestors between .child and .grandparent (inclusive)
var ancestors = $(".child").parentsUntil(".grandparent");
// Log the number of selected ancestors and their HTML content
console.log("Number of ancestors:", ancestors.length);
ancestors.each(function(index) {
console.log("Ancestor " + index + ":", $(this).html());
});
});
</script>
</body>
</html>
In this example, we have a DOM hierarchy with three nested <div>
elements: grandparent, parent, and child. We use the parentsUntil()
method to select all the ancestors of the element with the class “child” that lie between the “child” and “grandparent” (inclusive). The method returns a jQuery object containing these ancestor elements.
The output of the above example will be:
Number of ancestors: 1
Ancestor 0: <div class="parent">
<div class="child">
Some content here.
</div>
</div>
In this output, we see that there is one ancestor element selected, which is the parent of the original element with class “child.” It lies between the starting ancestor (element with class “child”) and the ending ancestor (element with class “grandparent”).
The parentsUntil()
method is useful when you want to select a range of ancestors between two specified elements. It provides a way to traverse the DOM hierarchy selectively and efficiently.