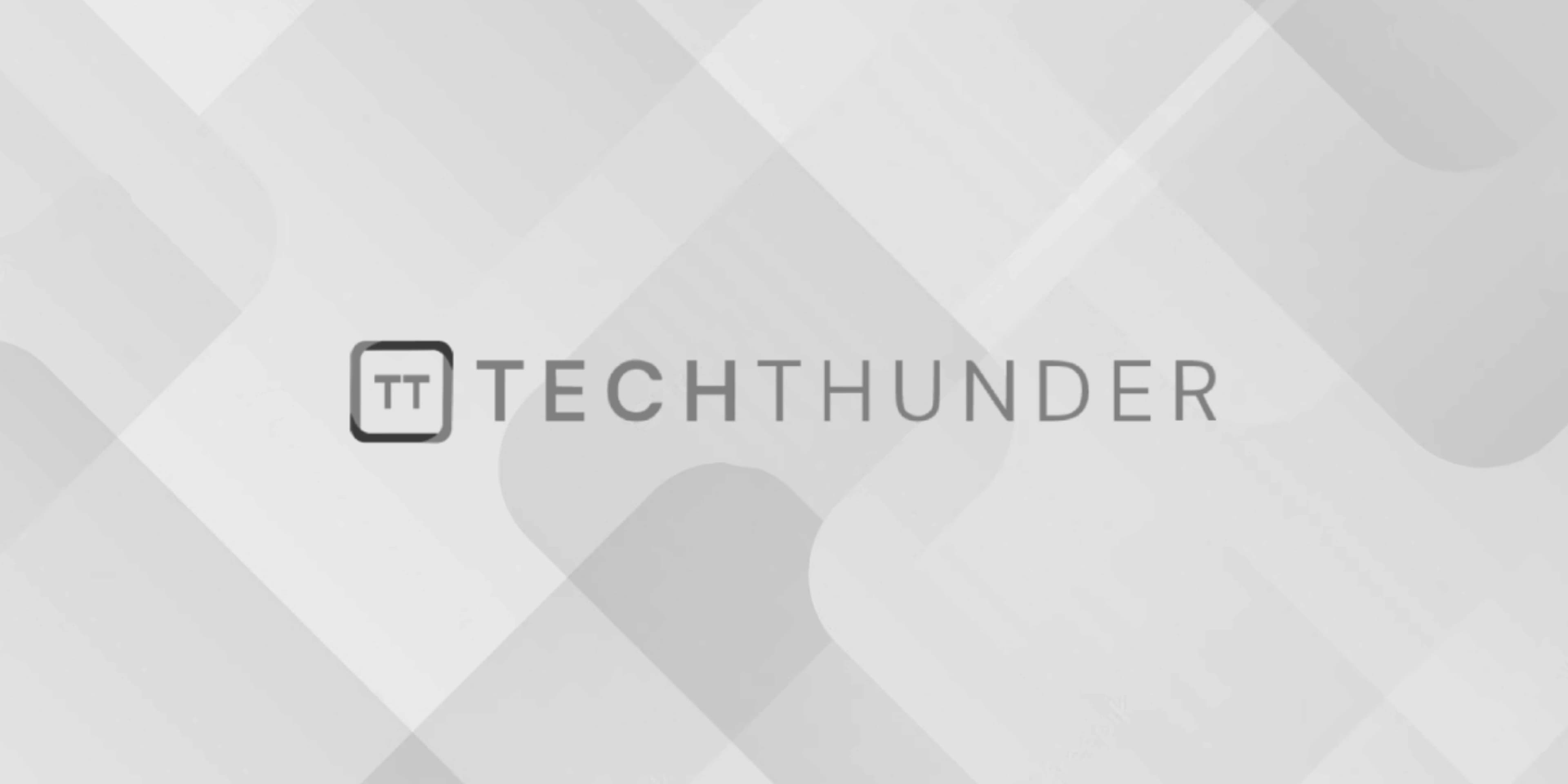
Create menu item animation on hover
To create a menu item animation on hover using jQuery and CSS, you can add a smooth transition effect to the menu items when the user hovers over them. Below is an example of how to achieve this effect:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Menu Item Animation on Hover</title>
<link rel="stylesheet" href="style.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</head>
<body>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</body>
</html>
CSS (style.css):
body {
font-family: Arial, sans-serif;
}
nav {
background-color: #333;
padding: 10px;
}
ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
}
li {
margin: 0 10px;
position: relative;
transition: all 0.3s ease;
}
a {
color: #fff;
text-decoration: none;
}
li::before {
content: '';
position: absolute;
bottom: -3px;
left: 0;
width: 100%;
height: 2px;
background-color: #fff;
transform: scaleX(0);
transition: transform 0.3s ease;
}
li:hover {
transform: translateY(-3px);
}
li:hover::before {
transform: scaleX(1);
}
JavaScript (script.js):
// JavaScript is not needed for the animation in this example.
// You can add JavaScript functionality for other menu interactions if required.
In this example, we create a simple navigation menu with four menu items. When the user hovers over a menu item, it will smoothly move up (transform: translateY(-3px);
) and display a horizontal line at the bottom (::before
pseudo-element with transform: scaleX(1);
) as an animation effect. The transition is achieved using CSS transitions.
Please note that the animation effect is achieved entirely with CSS, and the JavaScript code provided is empty because it is not needed for this particular animation. However, you can add JavaScript functionality for other interactions in your menu if required.