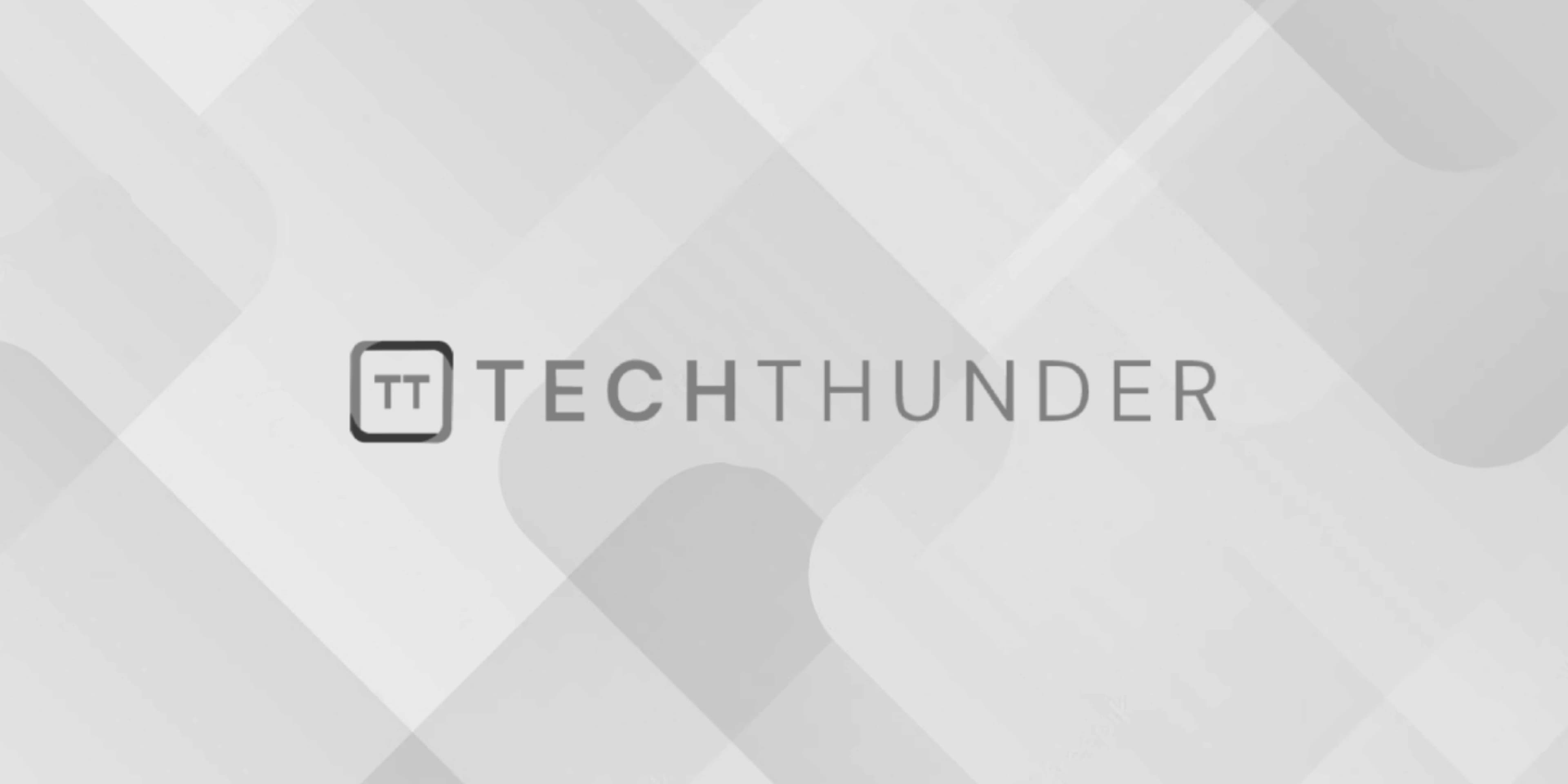
Automatically Format your Numbers and Currencies using jquery
The automatically format numbers and currencies using jQuery, you can use a third-party library like “jQuery Number and Currency Formatter Plugin.” This plugin allows you to easily format numbers, currencies, and other numeric values with options for precision, separators, and currency symbols.
Here’s how you can use the “jQuery Number and Currency Formatter Plugin”:
Step 1: Include Dependencies
First, include the required jQuery library and the “jQuery Number and Currency Formatter Plugin” in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Number and Currency Formatting</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-number/2.1.6/jquery.number.min.js"></script>
</head>
<body>
<!-- Your content goes here -->
<script src="script.js"></script>
</body>
</html>
Step 2: Format Numbers and Currencies
In your JavaScript file (e.g., script.js
), you can use the “jQuery Number and Currency Formatter Plugin” to format numbers and currencies:
$(document).ready(function() {
// Format a number with default options
var number = 1234567.89;
var formattedNumber = $.number(number);
console.log(formattedNumber); // Output: "1,234,567.89"
// Format a currency with custom options
var currency = 1234567.89;
var formattedCurrency = $.number(currency, 2, '.', ',');
console.log(formattedCurrency); // Output: "1,234,567.89"
// Format a currency with currency symbol
var currencyWithSymbol = 1234567.89;
var formattedCurrencyWithSymbol = $.number(currencyWithSymbol, 2, '.', ',', '$');
console.log(formattedCurrencyWithSymbol); // Output: "$1,234,567.89"
});
In this example, we demonstrate formatting a number and currency using the “jQuery Number and Currency Formatter Plugin.” The $.number()
function is used to format the numeric values. You can specify the precision (number of decimal places), the decimal separator, and the thousands separator as arguments. Additionally, you can include the currency symbol if formatting a currency value.
The plugin provides flexibility in formatting numeric values to suit different regional and currency formats.
Please note that there are other jQuery-based formatting plugins available as well. If the “jQuery Number and Currency Formatter Plugin” doesn’t meet your specific requirements, you can explore other alternatives that offer different features and customization options. Always ensure to use plugins from reputable sources and check their documentation for usage instructions and supported options.