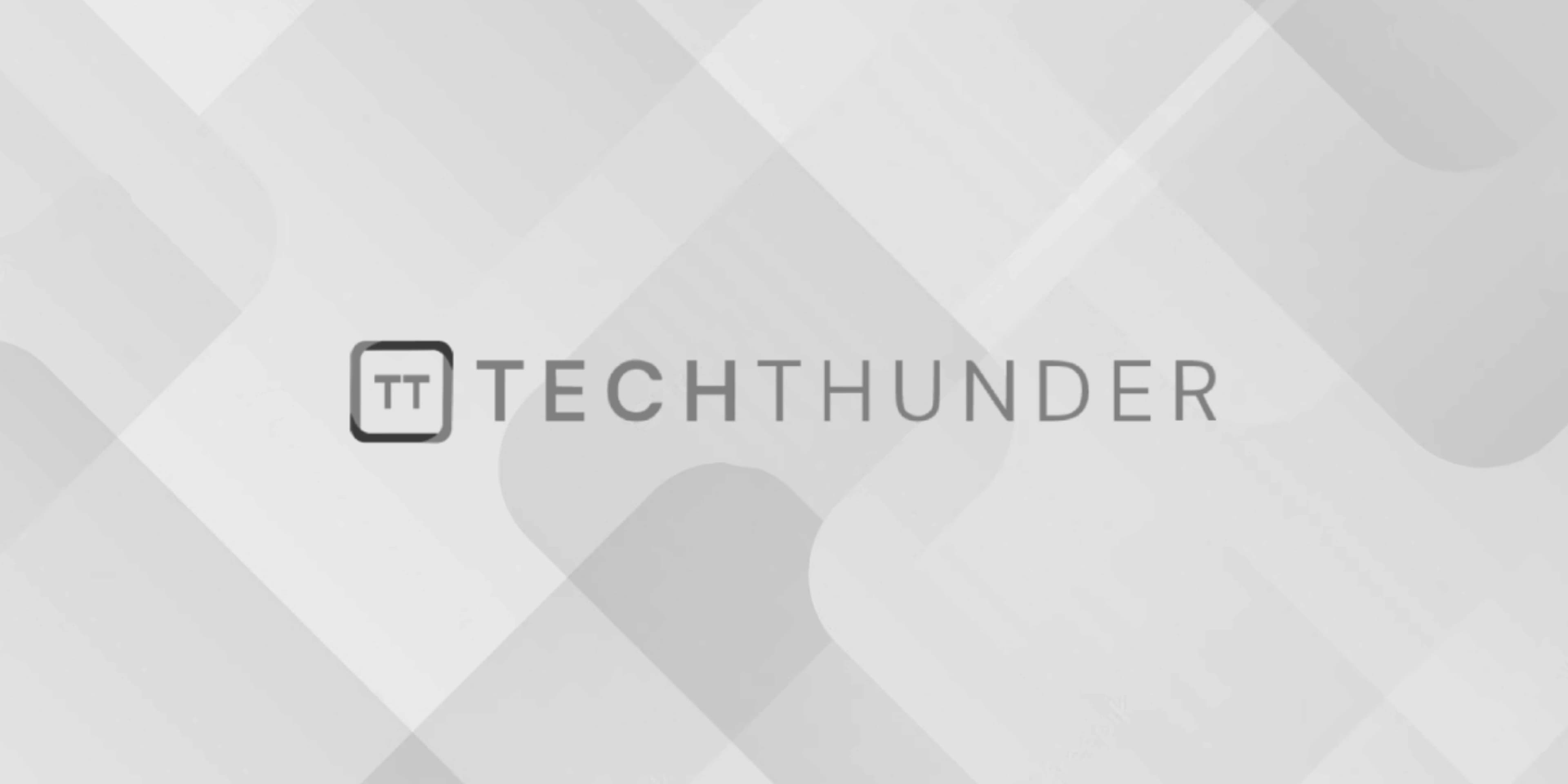
jQuery isArray() method
The jQuery.isArray()
method is a utility function in jQuery used to check whether a given value is an array or not. It is designed to provide a consistent way of determining if a variable is an array, even in older browsers where the native Array.isArray()
method may not be available.
Here’s the basic syntax of the jQuery.isArray()
method:
jQuery.isArray(obj)
Parameters:
obj
: The variable or value to be tested.
Return Value:
The jQuery.isArray()
method returns true
if the specified value is an array, and false
otherwise.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery isArray() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<script>
$(document).ready(function() {
var arr = [1, 2, 3];
var obj = { name: "John", age: 30 };
var str = "Hello, world!";
// Check if the variables are arrays
var arrIsArray = jQuery.isArray(arr);
var objIsArray = jQuery.isArray(obj);
var strIsArray = jQuery.isArray(str);
// Log the results to the console
console.log("arr is array:", arrIsArray); // true
console.log("obj is array:", objIsArray); // false
console.log("str is array:", strIsArray); // false
});
</script>
</body>
</html>
In this example, we have three variables: arr
, obj
, and str
. We use the jQuery.isArray()
method to check whether each of these variables is an array or not. The method returns true
for the arr
variable because it is an array, and false
for the obj
and str
variables because they are not arrays.
Keep in mind that starting from jQuery version 3.0, the recommended way to check if a variable is an array is to use the native Array.isArray()
method, which is more widely supported in modern browsers:
Array.isArray(obj)
For modern projects, it is generally preferable to use Array.isArray()
for better compatibility and performance. However, the jQuery.isArray()
method remains available in jQuery for backward compatibility with older codebases or specific use cases.