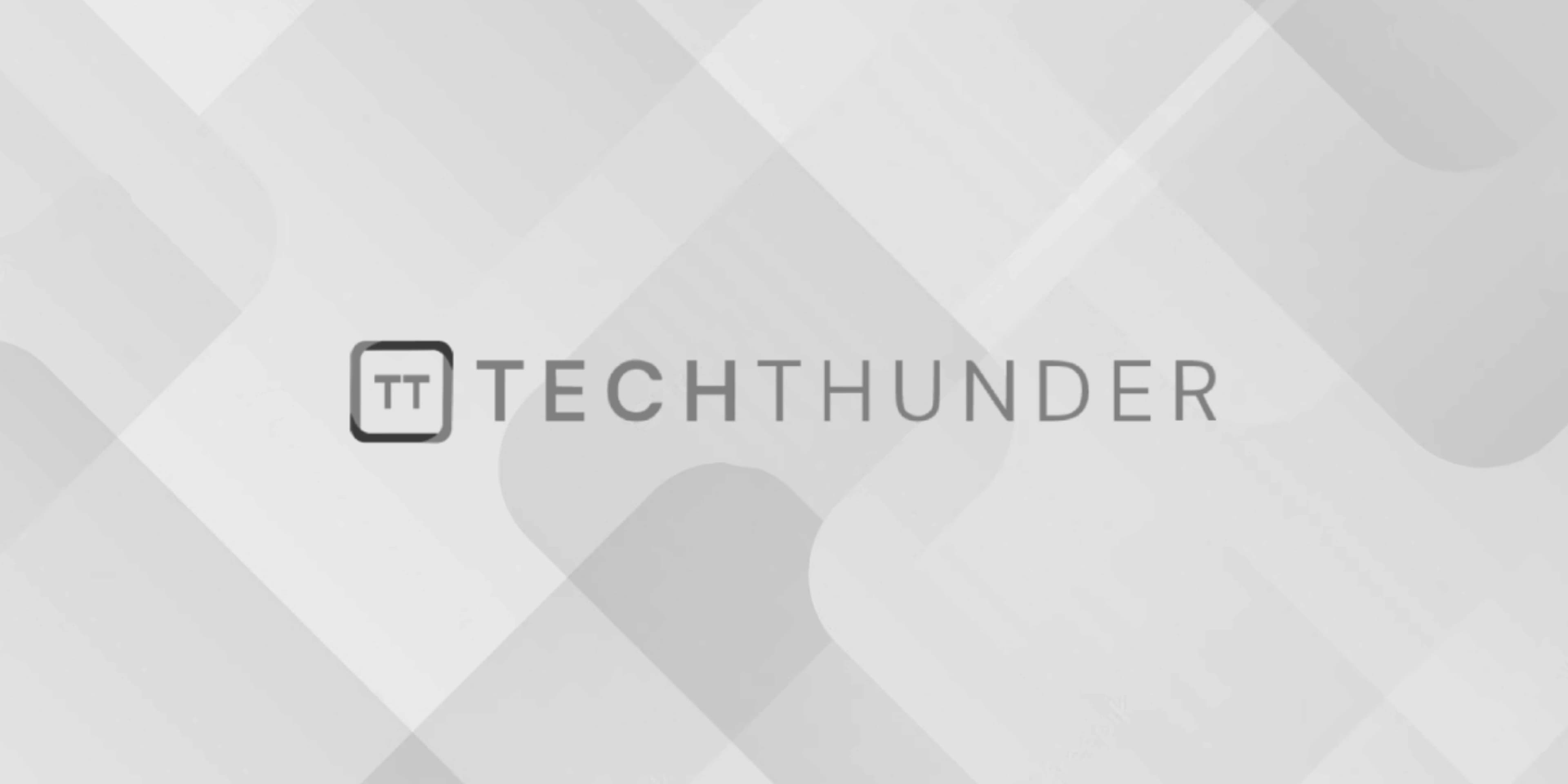
jQuery Animated Border Plugin
A jQuery Animated Border Plugin is a JavaScript library that can be integrated into your web projects to add visually appealing animations to HTML elements’ borders. Here’s what you might expect from such a plugin:
To create an animated border effect around an element using jQuery, follow these steps:
Step 1: Include Dependencies
First, include the required jQuery library in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Animated Border Example</title>
<!-- Include jQuery library -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<!-- Your content goes here -->
<div class="animated-border"></div>
<script src="script.js"></script>
</body>
</html>
Step 2: CSS for Animated Border
In your CSS file (e.g., styles.css
), define the styles for the animated border:
.animated-border {
position: relative;
width: 200px;
height: 100px;
border: 2px solid transparent;
overflow: hidden;
}
.animated-border:before {
content: '';
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
border: 2px solid #f00;
transform-origin: top left;
animation: animateBorder 3s infinite linear;
}
@keyframes animateBorder {
0% {
border-width: 2px;
transform: scaleX(0);
}
100% {
border-width: 2px;
transform: scaleX(1);
}
}
In this example, we create an .animated-border
container and use the ::before
pseudo-element to create the animated border. The border starts from a width of 0 and scales up to a full width using a CSS animation defined by the animateBorder
keyframes.
Step 3: Optional – Modify Animation Duration
You can modify the animation duration (currently set to 3 seconds) by adjusting the animation
property in the .animated-border:before
CSS rule.
Step 4: jQuery (Optional)
If you want to control the animation with jQuery, you can use jQuery to add or remove the animated-border
class dynamically based on user interactions or other events:
$(document).ready(function() {
$('.my-element').hover(
function() {
$(this).addClass('animated-border');
},
function() {
$(this).removeClass('animated-border');
}
);
});
In this example, we use jQuery to add the animated-border
class to the .my-element
when the user hovers over the element and remove it when the hover ends.
Please note that the example above demonstrates a basic animated border effect using CSS and jQuery. You can customize the styles, animation, and jQuery interactions as per your specific project requirements to create more complex and appealing animated borders.