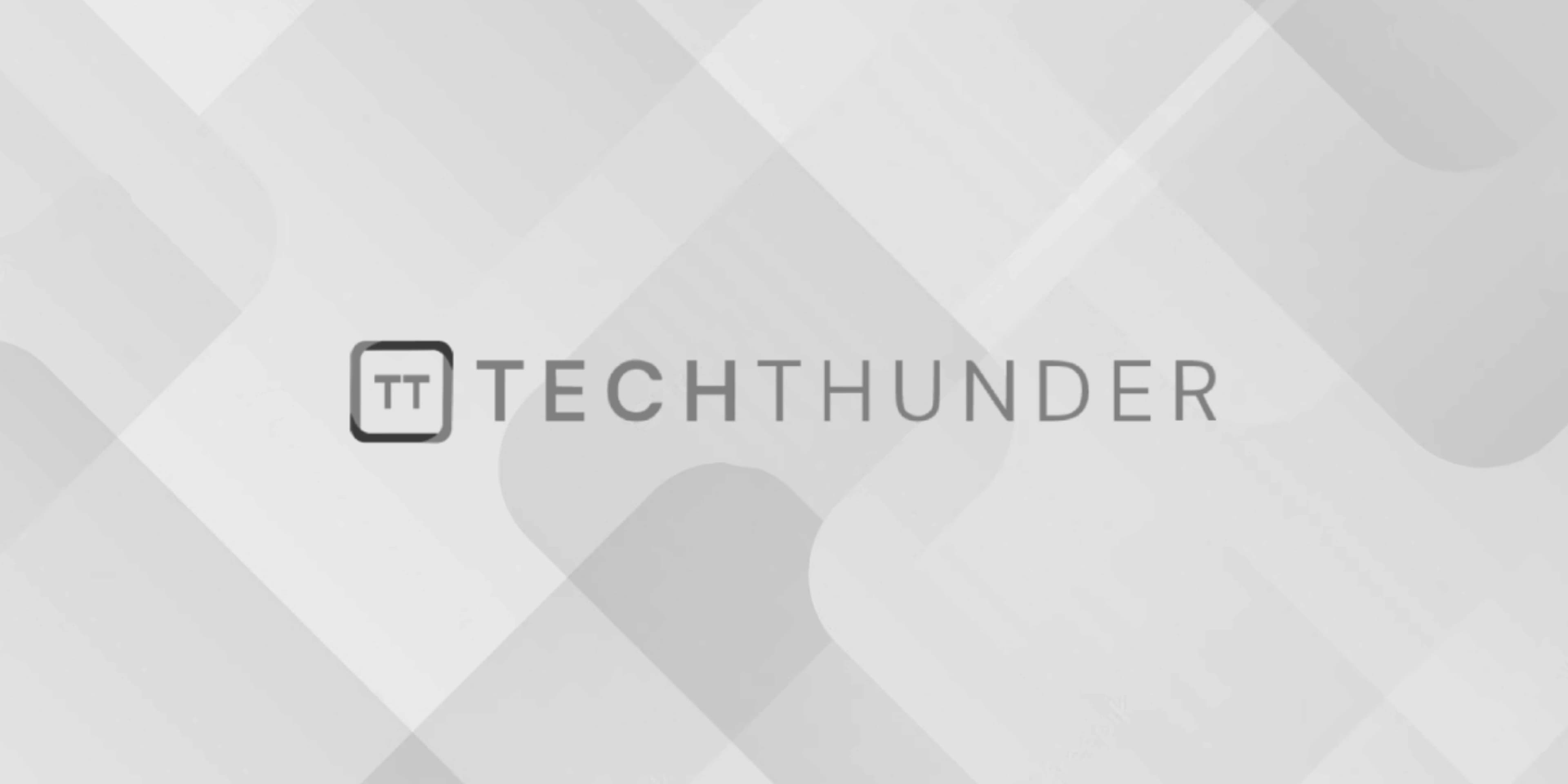
Phone Number Keyboard using HTML and jQuery
To create a phone number keyboard using HTML and jQuery, you can use a combination of HTML elements for the keyboard layout and jQuery to handle the input events.
Here’s a simple example of a phone number keyboard:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Phone Number Keyboard</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="phone-container">
<input type="text" id="phoneInput" readonly>
<div class="keyboard">
<div class="row">
<button class="number">1</button>
<button class="number">2</button>
<button class="number">3</button>
</div>
<div class="row">
<button class="number">4</button>
<button class="number">5</button>
<button class="number">6</button>
</div>
<div class="row">
<button class="number">7</button>
<button class="number">8</button>
<button class="number">9</button>
</div>
<div class="row">
<button id="clear">Clear</button>
<button class="number">0</button>
<button id="delete">Delete</button>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
}
.phone-container {
max-width: 300px;
margin: 50px auto;
}
input[type="text"] {
width: 100%;
padding: 10px;
font-size: 1.5rem;
text-align: center;
border: 2px solid #ccc;
border-radius: 8px;
}
.keyboard {
margin-top: 20px;
}
.row {
display: flex;
justify-content: space-between;
margin-bottom: 5px;
}
button {
width: 30%;
padding: 10px;
font-size: 1.5rem;
background-color: #f2f2f2;
border: 2px solid #ccc;
border-radius: 8px;
cursor: pointer;
}
button:hover {
background-color: #d9d9d9;
}
#clear,
#delete {
background-color: #ff6666;
color: #fff;
}
JavaScript (script.js):
$(document).ready(function() {
let phoneNumber = '';
$('.number').on('click', function() {
const digit = $(this).text();
phoneNumber += digit;
updatePhoneNumber();
});
$('#clear').on('click', function() {
phoneNumber = '';
updatePhoneNumber();
});
$('#delete').on('click', function() {
phoneNumber = phoneNumber.slice(0, -1);
updatePhoneNumber();
});
function updatePhoneNumber() {
$('#phoneInput').val(phoneNumber);
}
});
We create a simple phone number keyboard with HTML buttons for each digit from 0 to 9. The user can click the buttons to input the digits into the phone number input field. We also add “Clear” and “Delete” buttons to clear the phone number or delete the last digit, respectively.
When the user clicks on a digit button, the corresponding digit is appended to the phone number, and the updated phone number is displayed in the input field. The “Clear” button clears the phone number, and the “Delete” button removes the last digit from the phone number.
When you open the HTML file in your browser, you can use the phone number keyboard to input a phone number into the input field. This simple example can be further customized and enhanced to meet the specific requirements of your project.