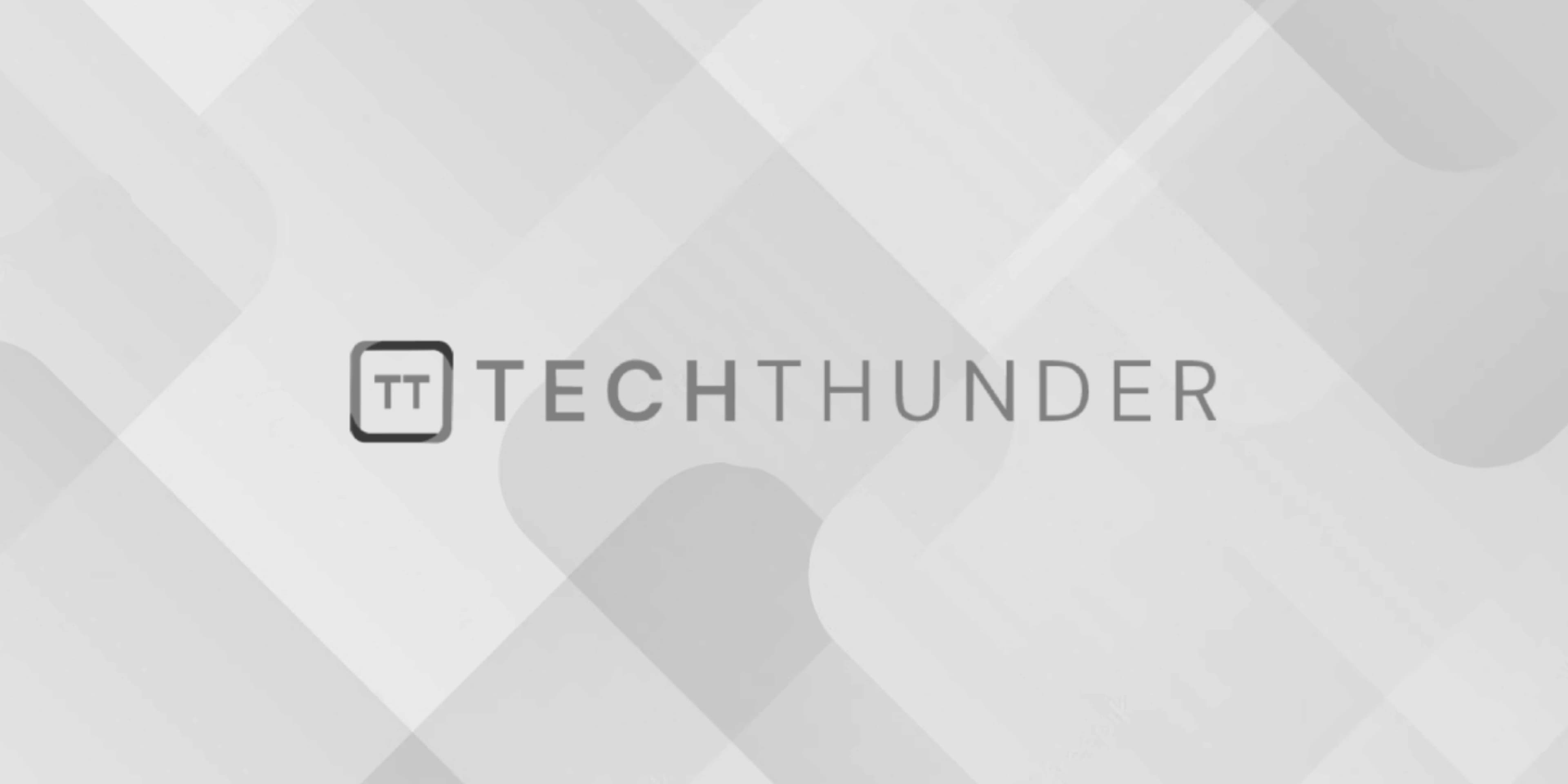
jQuery PayPal HTML Shop
As of my last knowledge update in September 2021, I can provide you with a basic example of a jQuery-powered HTML shop that integrates with PayPal for payments. Keep in mind that actual e-commerce implementations can be more complex and may involve server-side processing for security and inventory management. Below is a simple example of a static HTML shop that utilizes jQuery and PayPal’s sandbox environment for testing payments.
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Simple PayPal Shop</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</head>
<body>
<h1>Simple PayPal Shop</h1>
<div class="shop">
<div class="item">
<img src="item1.jpg" alt="Item 1">
<h2>Item 1</h2>
<p>Price: $10</p>
<button class="buy-btn" data-amount="10">Buy Now</button>
</div>
<div class="item">
<img src="item2.jpg" alt="Item 2">
<h2>Item 2</h2>
<p>Price: $15</p>
<button class="buy-btn" data-amount="15">Buy Now</button>
</div>
</div>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
// Replace the below sandbox client ID with your PayPal sandbox client ID
const paypalClientId = 'YOUR_SANDBOX_CLIENT_ID';
// Load the PayPal script dynamically
$.getScript('https://www.paypal.com/sdk/js?client-id=' + paypalClientId + '¤cy=USD', function() {
// Initialize the PayPal button for each buy button
$('.buy-btn').each(function() {
const amount = $(this).data('amount');
paypal.Buttons({
createOrder: function(data, actions) {
return actions.order.create({
purchase_units: [{
amount: {
value: amount
}
}]
});
},
onApprove: function(data, actions) {
return actions.order.capture().then(function(details) {
alert('Transaction completed! Thank you for your purchase.');
// Perform additional actions (e.g., update inventory, send confirmation emails, etc.) here.
});
}
}).render(this);
});
});
});
In this example, we have a simple HTML shop with two items. Each item has an image, title, price, and a “Buy Now” button with the corresponding data attribute data-amount
, which indicates the item’s price.
When the page is loaded, the script dynamically loads the PayPal SDK script with your sandbox client ID. You need to replace 'YOUR_SANDBOX_CLIENT_ID'
with your actual PayPal sandbox client ID.
For each “Buy Now” button, the script initializes a PayPal button using the PayPal JavaScript SDK. When the user clicks the “Buy Now” button, a PayPal payment dialog will appear, allowing the user to complete the transaction using their PayPal account or credit card in the sandbox environment.
Please note that this is a basic example, and in a real-world scenario, you should perform additional server-side processing, such as verifying the payment on your server, handling shipping details, updating inventory, and managing order history. Additionally, you should use a secure and authenticated server-side environment for processing payments in a production environment.
Remember that technologies and features may evolve over time, so it’s always a good idea to check the latest documentation and best practices provided by PayPal for implementing an e-commerce solution.