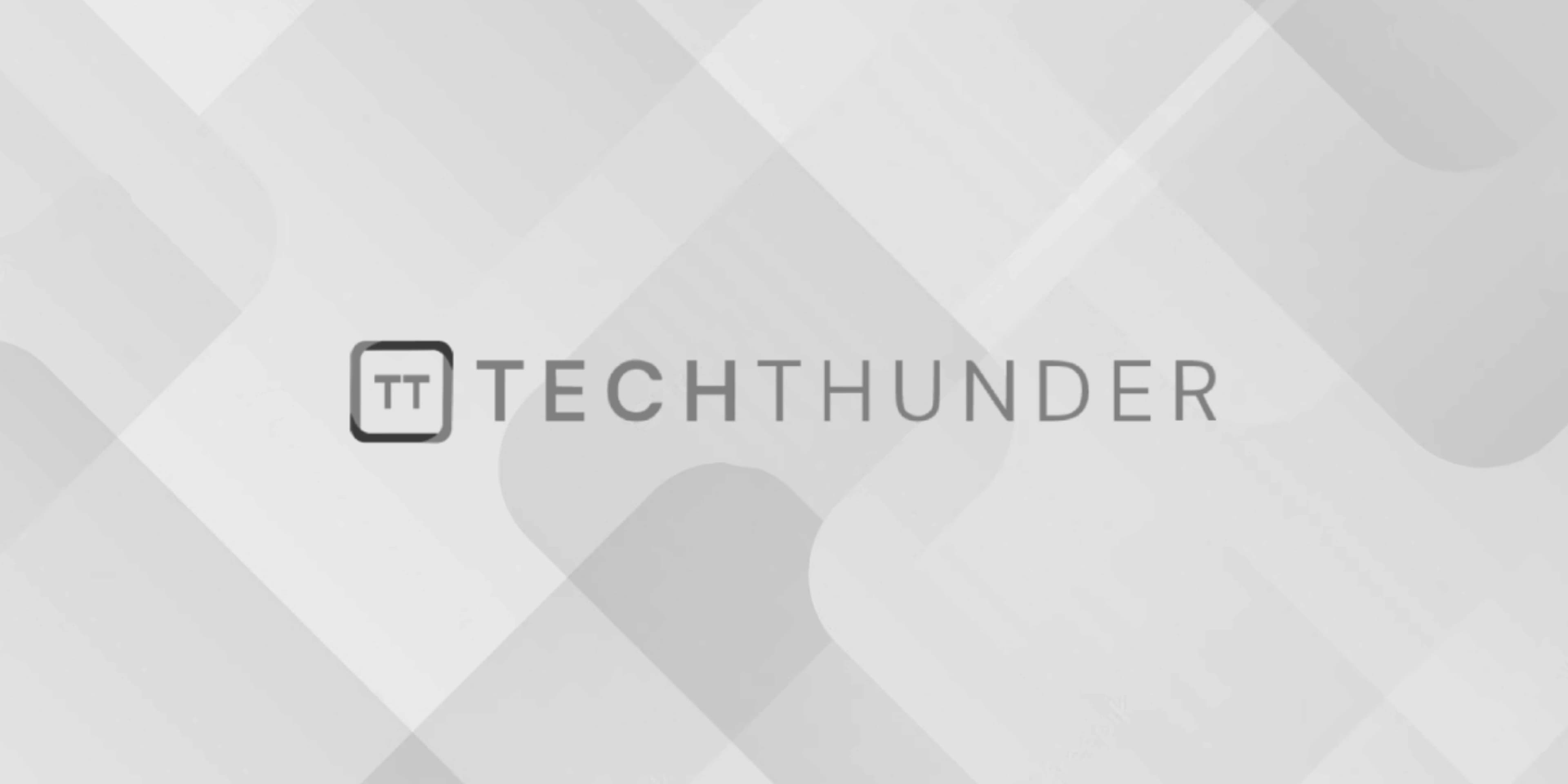
Jquery dragStart Event
There is no native dragStart
event in jQuery. But jQuery does provide a set of drag-related events for handling drag interactions with elements.
The primary drag events provided by jQuery are:
- dragstart: This event is triggered when the user starts dragging an element.
- drag: This event is triggered while the user is dragging an element.
- dragend: This event is triggered when the user finishes dragging an element.
The dragstart
event is particularly useful when you want to perform custom actions at the beginning of a drag operation, such as showing a visual cue or preparing data for the drag.
Here’s an example of how you can use the dragstart
event in jQuery:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>dragstart Event Example</title>
<style>
.drag-me {
width: 100px;
height: 100px;
background-color: #f00;
cursor: move;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</head>
<body>
<div class="drag-me"></div>
</body>
</html>
JavaScript (script.js):
$(document).ready(function() {
$('.drag-me').on('dragstart', function(event) {
// This function will be executed when the drag operation starts
console.log('Drag operation started.');
// Your custom code to handle the dragstart event goes here
});
// Add draggable behavior to the element
$('.drag-me').draggable();
});
In this example, we have a <div>
element with the class “drag-me.” When the user starts dragging this element, the dragstart
event is triggered, and the message “Drag operation started.” will be logged to the browser console.
Please note that the dragstart
event may not work with all elements by default. Some elements, such as images, links, and text selections, have their own default drag behavior. To enable drag events on custom elements, you may need to add the draggable
attribute to the element or use CSS to override the default behavior.
Also, keep in mind that drag-related events are specific to drag-and-drop interactions and may not work as expected on touch devices or older browsers. If you need cross-platform drag-and-drop support, consider using a drag-and-drop library that provides better compatibility and additional features.