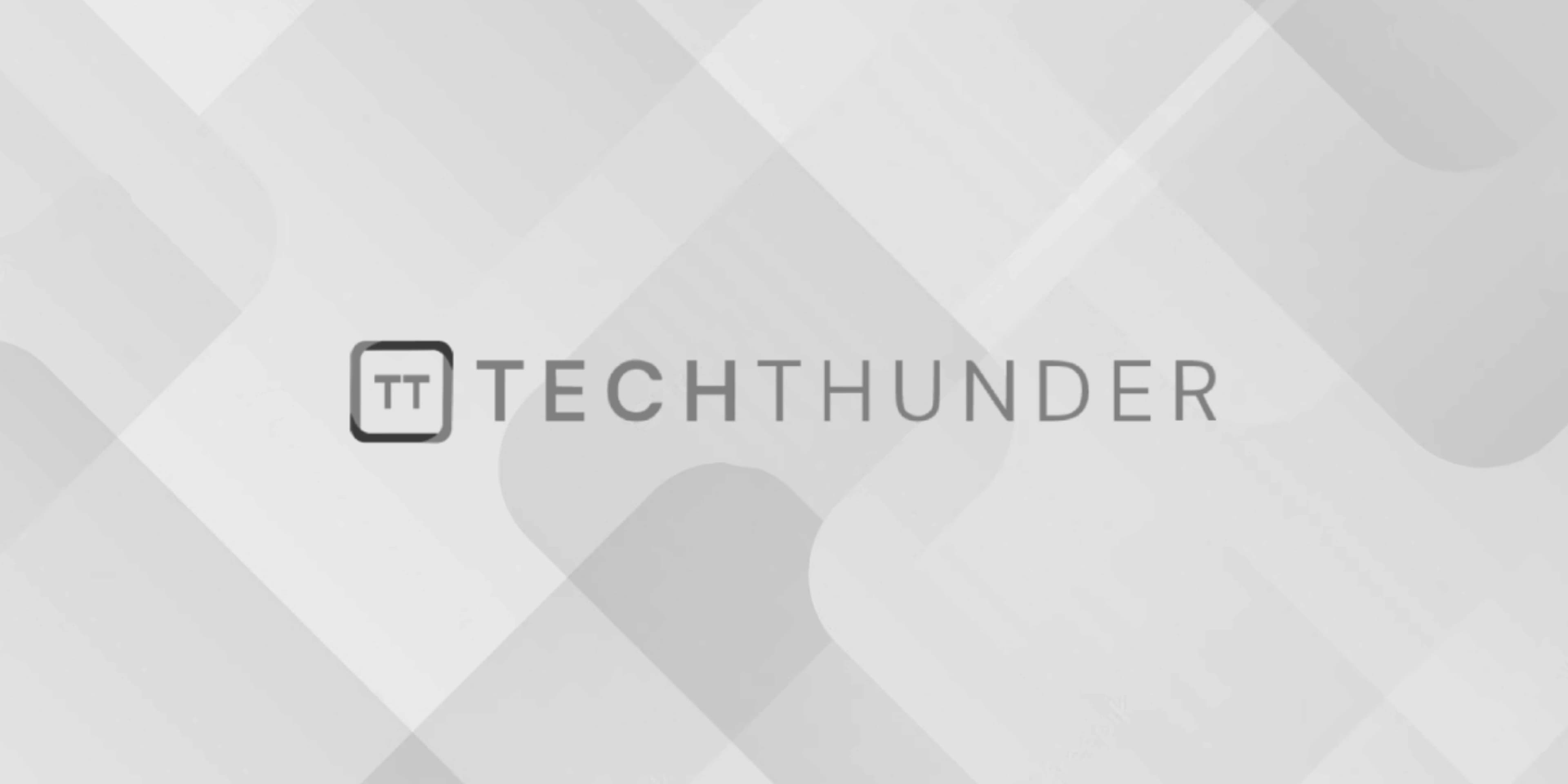
QR Code Generator using HTML, CSS, and jQuery
To create a QR Code generator using HTML, CSS, and jQuery, we can use a QR Code generation library called “qrcode.js.” This library allows us to generate QR Codes based on the data input by the user.
Here’s a step-by-step guide to building a simple QR Code generator:
- Create the HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>QR Code Generator</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="qr-generator">
<h1>QR Code Generator</h1>
<input type="text" id="text-input" placeholder="Enter text or URL...">
<button id="generate-btn">Generate QR Code</button>
<div id="qrcode"></div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="qrcode.min.js"></script>
<script src="script.js"></script>
</body>
</html>
- Create the CSS (styles.css):
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 0;
}
.qr-generator {
max-width: 400px;
margin: 50px auto;
padding: 20px;
border: 2px solid #ccc;
border-radius: 8px;
}
h1 {
margin-top: 0;
}
input[type="text"] {
width: 70%;
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
margin-right: 10px;
}
button {
padding: 8px 16px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
#qrcode {
margin-top: 20px;
}
- Implement the JavaScript (script.js):
$(document).ready(function() {
$('#generate-btn').on('click', function() {
const inputValue = $('#text-input').val();
if (inputValue.trim() !== '') {
generateQRCode(inputValue);
}
});
function generateQRCode(data) {
const qrCodeDiv = document.getElementById('qrcode');
qrCodeDiv.innerHTML = ''; // Clear previous QR code
const qrcode = new QRCode(qrCodeDiv, {
text: data,
width: 200,
height: 200,
});
}
});
- Download the qrcode.min.js file from the qrcode.js GitHub repository: https://github.com/davidshimjs/qrcodejs
Place the downloaded qrcode.min.js file in the same directory as your HTML file.
With this code, you have created a simple QR Code generator. When you enter text or a URL in the input field and click the “Generate QR Code” button, it will display the corresponding QR Code below.
we use the qrcode.js library for QR Code generation. You can explore other QR Code generation libraries if you prefer, or you can customize the appearance and behavior of the QR Code generator to match your project requirements.