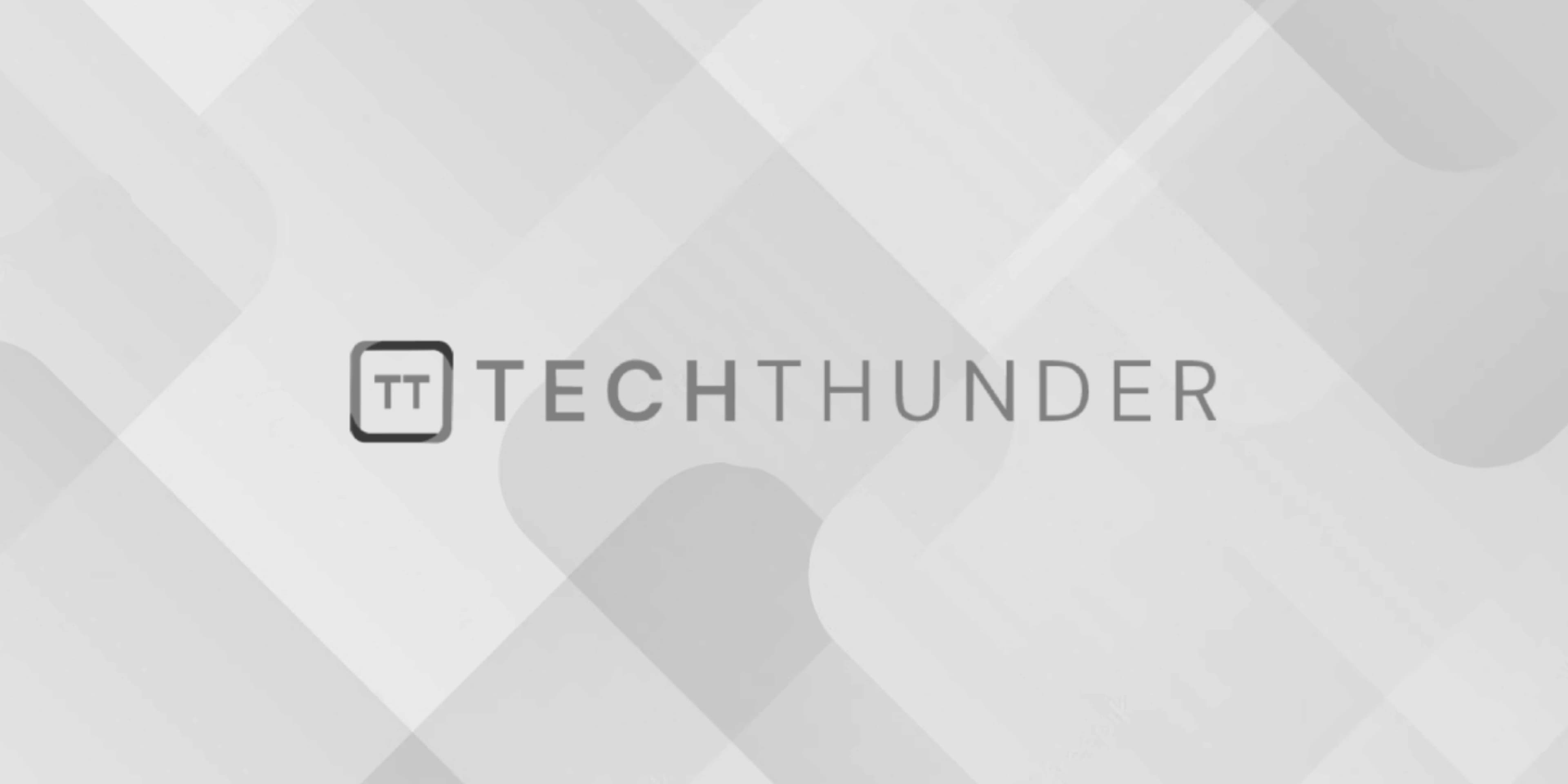
jQuery Palette Color Picker
One popular jQuery Palette Color Picker plugin is “Pickr” (also known as “Pickr2”). It is a simple, customizable, and easy-to-use color picker with various features and options. “Pickr” supports different color representation formats, such as HEX, RGB, and HSL, and allows you to integrate it into your web application easily.
Here’s how you can use the “Pickr” jQuery Palette Color Picker:
Step 1: Include Dependencies
First, include the required CSS and JavaScript files for “Pickr” in your HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Color Picker Example</title>
<!-- Include jQuery library -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<!-- Include Pickr CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/pickr/2.1.1/themes/nano.min.css">
<!-- Include Pickr JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/pickr/2.1.1/pickr.min.js"></script>
</head>
<body>
<!-- Your content goes here -->
<div id="color-picker"></div>
<script src="script.js"></script>
</body>
</html>
Step 2: Initialize Pickr Color Picker
In your JavaScript file (e.g., script.js
), you can use “Pickr” to initialize the color picker:
$(document).ready(function() {
const pickr = Pickr.create({
el: '#color-picker',
theme: 'nano', // You can choose other themes as well
swatches: [
'rgba(244, 67, 54, 1)',
'rgba(233, 30, 99, 0.95)',
'rgba(156, 39, 176, 0.9)',
'rgba(103, 58, 183, 0.85)',
'rgba(63, 81, 181, 0.8)',
'rgba(33, 150, 243, 0.75)',
'rgba(3, 169, 244, 0.7)',
'rgba(0, 188, 212, 0.7)',
'rgba(0, 150, 136, 0.75)',
'rgba(76, 175, 80, 0.8)',
'rgba(139, 195, 74, 0.85)',
'rgba(205, 220, 57, 0.9)',
'rgba(255, 235, 59, 0.95)',
'rgba(255, 193, 7, 1)'
],
components: {
// Main components
preview: true,
opacity: true,
hue: true,
// Input / output Options
interaction: {
hex: true,
rgba: true,
hsla: true,
hsva: true,
cmyk: true,
input: true,
clear: true,
save: true
}
}
});
// Listen for color change events
pickr.on('change', (color, instance) => {
console.log(color.toHEXA().toString()); // Output: HEX representation of the selected color
});
});
In this example, we use “Pickr” to create a color picker inside the #color-picker
element. You can customize the color picker’s appearance and features by adjusting the theme
and components
options. The swatches
option provides some predefined colors for quick selection.
The pickr.on('change', ...)
event listener allows you to capture color change events and retrieve the selected color in different color representation formats (HEX, RGBA, HSLA, etc.).
Please note that “Pickr” is just one example of a jQuery-based color picker plugin. There are other color picker plugins available with different features and customization options. Depending on your specific requirements, you may choose a different plugin that best suits your needs.
Always ensure to use plugins from reputable sources and check their documentation and support to ensure compatibility and reliability.