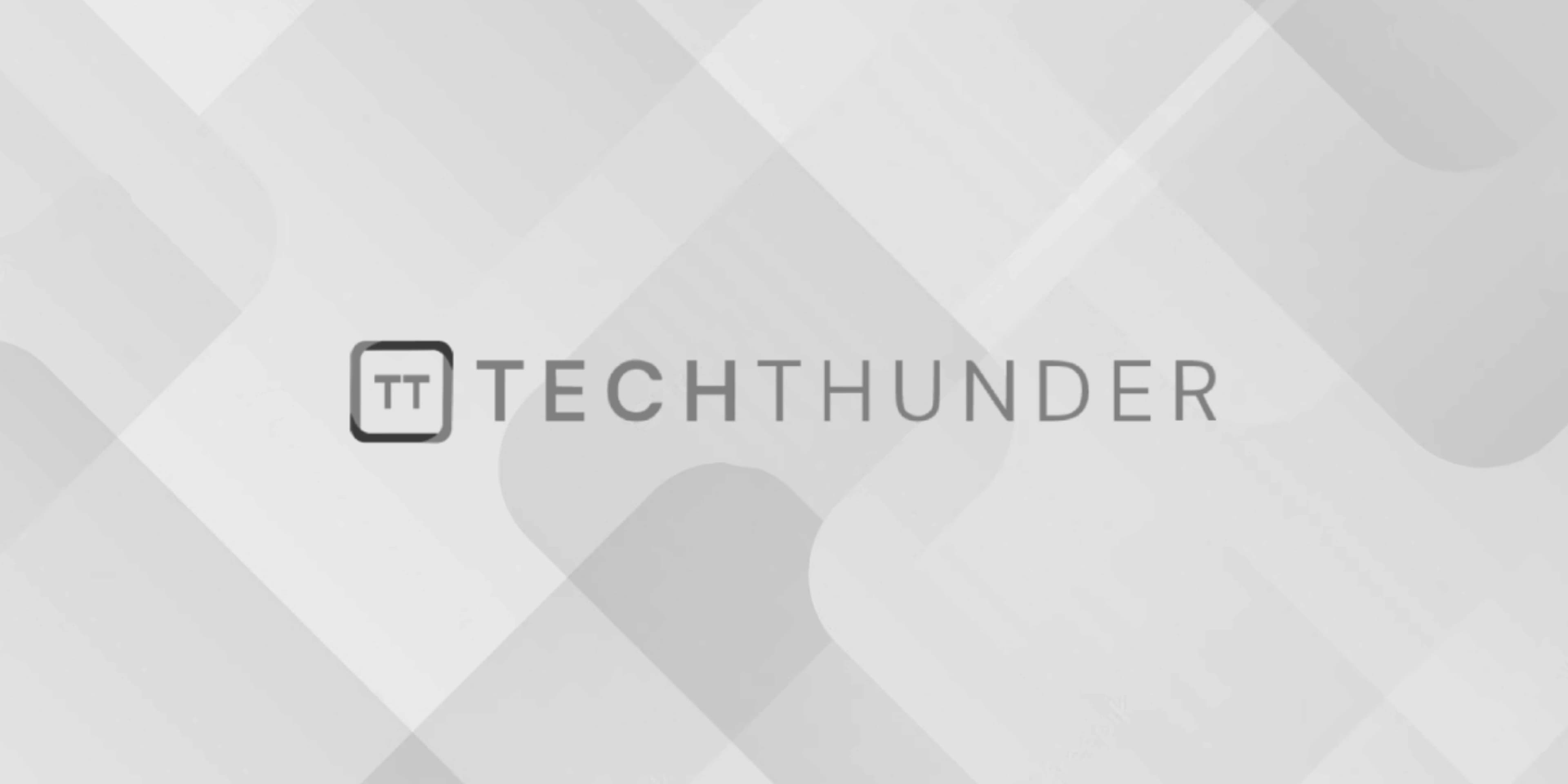
jQuery get Difference between two Dates in days
To get the difference between two dates in days using jQuery, you can use the JavaScript Date
object and some simple calculations. jQuery is not required for this task, as it can be achieved with plain JavaScript.
Here’s a function that calculates the difference between two dates in days:
<!DOCTYPE html>
<html>
<head>
<title>Calculate Date Difference in Days</title>
</head>
<body>
<div>
<label for="date1">Date 1:</label>
<input type="date" id="date1">
</div>
<div>
<label for="date2">Date 2:</label>
<input type="date" id="date2">
</div>
<button id="calculate">Calculate Difference</button>
<div id="result"></div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#calculate").click(function() {
var date1 = new Date($("#date1").val());
var date2 = new Date($("#date2").val());
// Calculate the time difference in milliseconds
var differenceInMs = Math.abs(date2 - date1);
// Convert the time difference to days
var differenceInDays = Math.floor(differenceInMs / (1000 * 60 * 60 * 24));
$("#result").text("Difference in days: " + differenceInDays);
});
});
</script>
</body>
</html>
In this example, we have two date input fields and a button. When the button is clicked, the function calculates the difference in days between the two input dates and displays the result in the result
div.
The JavaScript code first creates two Date
objects using the input values from the date fields. Then, it calculates the absolute difference in milliseconds between the two dates using Math.abs()
. Finally, it divides the time difference in milliseconds by the number of milliseconds in a day (1000 * 60 * 60 * 24
) to get the difference in days and displays it on the page.
Note that this code uses the input type="date"
HTML5 input type for date selection, which is supported by modern browsers. If you need to support older browsers, consider using a date picker library or using different date input formats.