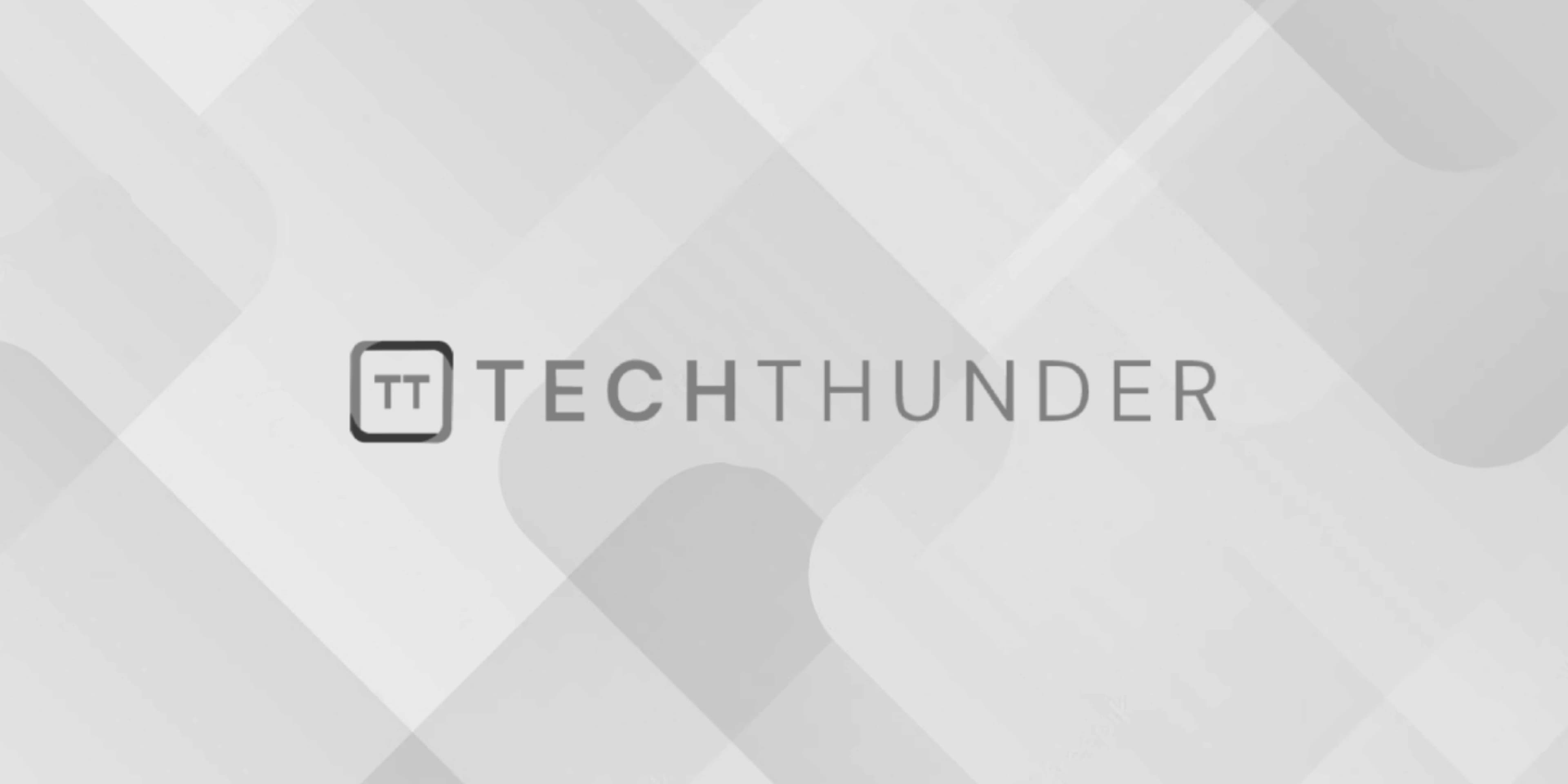
JQuery left side menu
To create a left-side menu using jQuery, you can follow these steps:
- Set up your HTML structure.
- Add CSS to style the menu.
- Use jQuery to handle menu interactions.
Here’s an example of how to create a simple left-side menu:
Step 1: HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>Left Side Menu</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="menu">
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</div>
<div class="content">
<!-- Your main content goes here -->
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Step 2: CSS (styles.css)
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
.menu {
background-color: #333;
height: 100%;
width: 250px;
position: fixed;
top: 0;
left: 0;
padding-top: 20px;
color: #fff;
}
.menu ul {
list-style: none;
padding: 0;
margin: 0;
}
.menu li {
padding: 10px;
}
.menu a {
color: #fff;
text-decoration: none;
}
.content {
margin-left: 250px; /* Width of the menu */
padding: 20px;
}
Step 3: JavaScript (script.js)
$(document).ready(function() {
// Toggle the menu on click of a button or icon
$('.menu-toggle').click(function() {
$('.menu').toggleClass('open');
});
});
The left-side menu is represented by a <div>
with the class “menu” containing a list of menu items. The content section is represented by a <div>
with the class “content.” The menu is initially hidden by using CSS positioning.
The JavaScript code uses jQuery to add a class of “open” to the menu when a menu-toggle button or icon is clicked. The “open” class changes the positioning to make the menu visible on the left side of the page.
You can further customize the menu’s appearance and behavior by adding more CSS styles and JavaScript code according to your requirements. You may also consider using CSS transitions or animations to create smooth transitions when opening and closing the menu.