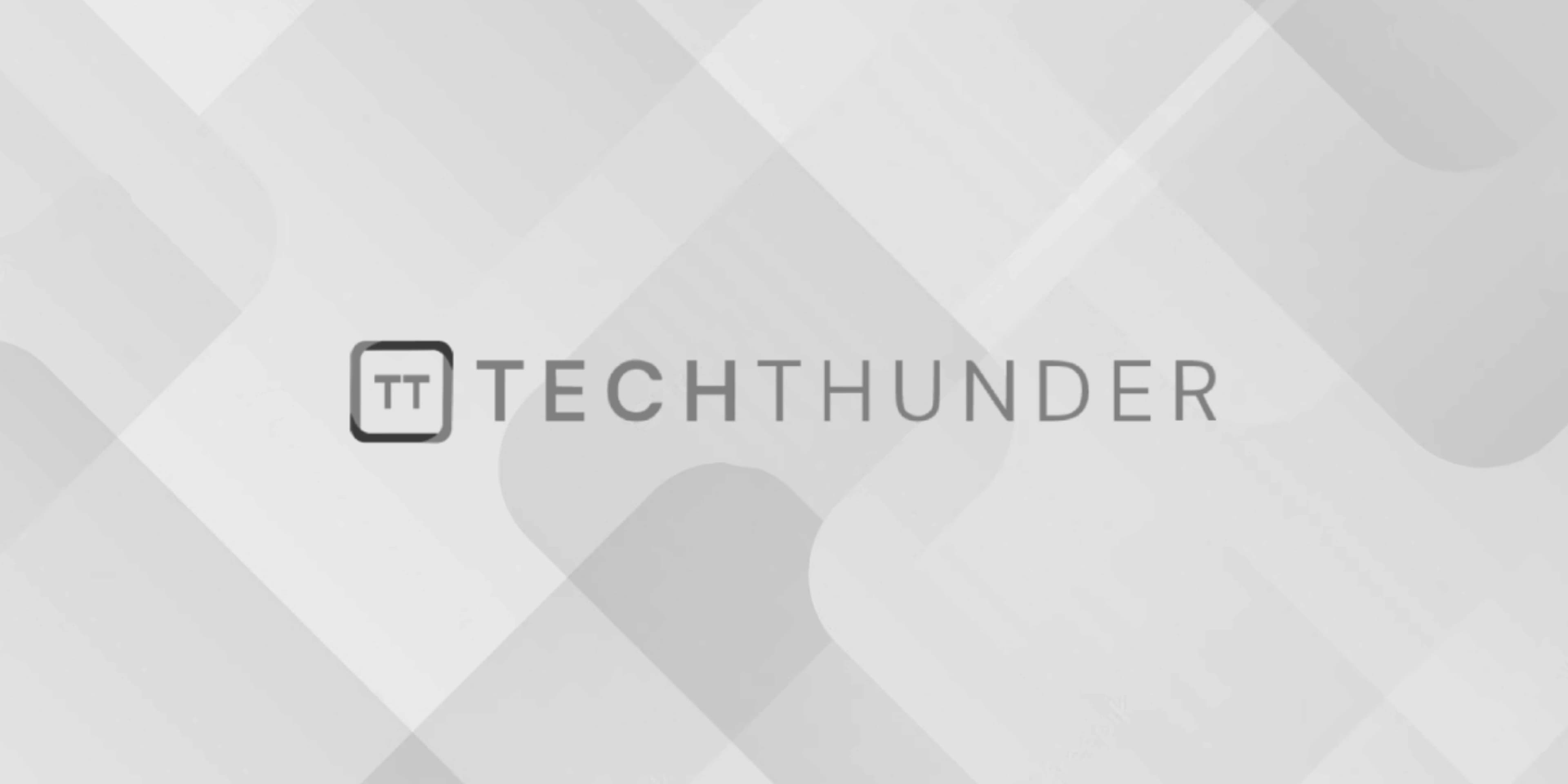
Language Translation with JavaScript
Language translation with JavaScript can be achieved using various APIs and libraries that provide translation services. One popular service is the Google Translate API, which allows you to easily integrate language translation into your web applications using JavaScript.
Please note that the availability and usage of translation services may vary, and some services might require API keys or have usage limits. Make sure to check the terms of service and documentation of the translation service you choose.
Below is an example of how to perform language translation using the Google Translate API and JavaScript:
Step 1: Get an API Key
To use the Google Translate API, you need to obtain an API key from the Google Cloud Console. Follow the instructions provided by Google to create a project and enable the Translate API, and then obtain the API key.
Step 2: Include Google Translate API Script
Include the Google Translate API script in your HTML file. Make sure to replace 'YOUR_API_KEY'
with the actual API key you obtained in Step 1.
<!DOCTYPE html>
<html>
<head>
<title>Language Translation Example</title>
</head>
<body>
<!-- Your content goes here -->
<!-- Include the Google Translate API script -->
<script src="https://translate.google.com/translate_a/element.js?cb=googleTranslateElementInit"></script>
<script src="script.js"></script>
</body>
</html>
Step 3: Initialize Google Translate
Create a JavaScript function to initialize the Google Translate widget. This function will be called when the API script is loaded.
// Callback function to initialize Google Translate
function googleTranslateElementInit() {
new google.translate.TranslateElement({
pageLanguage: 'en', // The default language of your website
includedLanguages: 'es,fr,de', // Comma-separated list of languages to include
layout: google.translate.TranslateElement.InlineLayout.SIMPLE,
}, 'google_translate_element');
}
Step 4: Display the Translation Widget
In your HTML file, add an element (e.g., a <div>
) where the translation widget will be displayed:
<div id="google_translate_element"></div>
Step 5: Test the Translation
Now, when you load your HTML file, the Google Translate widget will be displayed on your web page, allowing users to select different target languages to translate the content.
Keep in mind that the above example uses the Google Translate API to provide a simple translation widget on your website. For more advanced and customized translation features, you might need to explore other translation APIs or libraries that suit your specific requirements.
Additionally, always be mindful of any usage limitations or costs associated with using third-party translation services, and consider the privacy and security implications of handling user content in different languages.