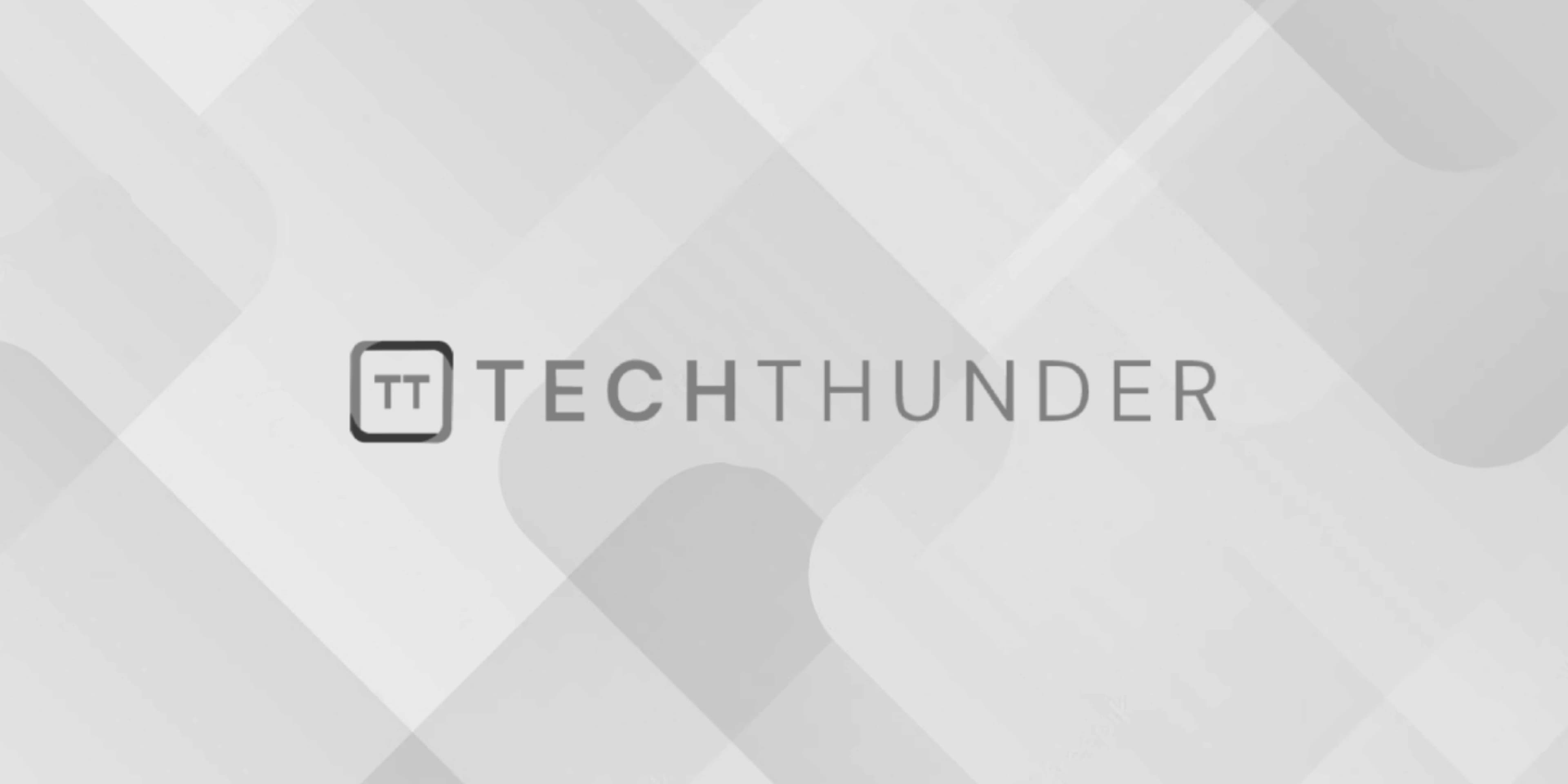
jQuery nextUntil() method
The jQuery.nextUntil()
method is used to select all the following sibling elements of each element in the jQuery object up to, but not including, the element matched by the specified until
selector. It allows you to target a range of sibling elements that appear after the selected element in the DOM hierarchy.
Here’s the basic syntax of the nextUntil()
method:
$(selector).nextUntil(until, [filter])
Parameters:
selector
: A selector expression used to select elements from which you want to filter out those that come after theuntil
element.until
: A selector expression used to select the element that marks the end of the range of elements to be selected. The elements up to, but not including, this element will be selected.filter
: Optional. A selector expression used to further filter the selected following sibling elements.
Return Value:
The nextUntil()
method returns a new jQuery object containing the selected following sibling elements up to, but not including, the until
element.
Example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery nextUntil() Method Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div>Div 1</div>
<p>Paragraph 1</p>
<span>Span 1</span>
<div>Div 2</div>
<p>Paragraph 2</p>
<script>
$(document).ready(function() {
// Select all following sibling elements of the <p> element up to the <div> element
var followingSiblings = $("p").nextUntil("div");
// Log the selected following sibling elements
console.log(followingSiblings);
});
</script>
</body>
</html>
In this example, we use the nextUntil()
method to select all the following sibling elements of the <p>
element up to, but not including, the first <div>
element. The method returns a jQuery object containing the <span>
element, which is the sibling that appears after the <p>
element, as well as the second <p>
element since it is between the first <p>
and the <div>
.
The output of the above example will be:
[<span>Span 1</span>, <p>Paragraph 2</p>]
In this output, we see that the nextUntil()
method successfully selected the desired range of following sibling elements of the <p>
element.
The nextUntil()
method is useful when you want to target a specific range of sibling elements that come after a particular element in the DOM hierarchy. It provides a convenient way to traverse the DOM tree and select elements based on their position relative to other elements.